muMAG Standard Problem #4
A detailed problem description can be found here
Google Colab Link
The demo can be run on Google Colab without any local installation. Use the following link to try it out.
[2]:
!pip install -q magnumnp numpy==1.22.4
Run Demo:
[3]:
#TODO: read latest script content from gitlab repository, as soon as %load works with Colab
from magnumnp import *
import torch
Timer.enable(log_mem = True)
# initialize mesh
eps = 1e-15
n = (100, 25, 1)
dx = (5e-9, 5e-9, 3e-9)
mesh = Mesh(n, dx)
state = State(mesh)
state.material = {
"Ms": 8e5,
"A": 1.3e-11,
"alpha": 0.02
}
# initialize field terms
demag = DemagField()
exchange = ExchangeField()
external = ExternalField([-24.6e-3/constants.mu_0,
+4.3e-3/constants.mu_0,
0.0])
# initialize magnetization that relaxes into s-state
state.m = state.Constant([0,0,0])
state.m[1:-1,:,:,0] = 1.0
state.m[(-1,0),:,:,1] = 1.0
# relax without external field
llg = LLGSolver([demag, exchange])
llg.relax(state)
write_vti(state.m, "data/m0.vti", state)
# perform integration with external field
llg = LLGSolver([demag, exchange, external])
logger = Logger("data", ['t', 'm'])
while state.t < 1e-9-eps:
llg.step(state, 1e-11)
logger << state
Timer.print_report()
2023-11-06 14:23:57 magnum.np:WARNING module 'torch' has no attribute 'compile'
2023-11-06 14:23:57 magnum.np:INFO magnum.np 1.1.3
/home/florian/.local/lib/python3.8/site-packages/torch/cuda/__init__.py:82: UserWarning: CUDA initialization: The NVIDIA driver on your system is too old (found version 10010). Please update your GPU driver by downloading and installing a new version from the URL: http://www.nvidia.com/Download/index.aspx Alternatively, go to: https://pytorch.org to install a PyTorch version that has been compiled with your version of the CUDA driver. (Triggered internally at ../c10/cuda/CUDAFunctions.cpp:112.)
return torch._C._cuda_getDeviceCount() > 0
2023-11-06 14:23:57 magnum.np:INFO [State] running on device: cpu (dtype = float64)
2023-11-06 14:23:57 magnum.np:INFO [Mesh] 100x25x1 (size= 5e-09 x 5e-09 x 3e-09)
2023-11-06 14:23:57 magnum.np:INFO [LLGSolver] using RKF45 solver (atol = 1e-05)
2023-11-06 14:23:57 magnum.np:INFO [DEMAG]: Time calculation of demag kernel = 0.1910877227783203 s
2023-11-06 14:23:58 magnum.np:INFO [LLG] relax: t=1e-11 dE=4.01585 E=9.19749e-19
2023-11-06 14:23:58 magnum.np:INFO [LLG] relax: t=2e-11 dE=0.269003 E=7.24781e-19
2023-11-06 14:23:58 magnum.np:INFO [LLG] relax: t=3e-11 dE=0.0524045 E=6.88691e-19
2023-11-06 14:23:58 magnum.np:INFO [LLG] relax: t=4e-11 dE=0.0177996 E=6.76647e-19
2023-11-06 14:23:58 magnum.np:INFO [LLG] relax: t=5e-11 dE=0.00863123 E=6.70856e-19
2023-11-06 14:23:58 magnum.np:INFO [LLG] relax: t=6e-11 dE=0.00541932 E=6.6724e-19
2023-11-06 14:23:58 magnum.np:INFO [LLG] relax: t=7e-11 dE=0.00400938 E=6.64576e-19
2023-11-06 14:23:58 magnum.np:INFO [LLG] relax: t=8e-11 dE=0.00325665 E=6.62418e-19
2023-11-06 14:23:58 magnum.np:INFO [LLG] relax: t=9e-11 dE=0.00278723 E=6.60577e-19
2023-11-06 14:23:58 magnum.np:INFO [LLG] relax: t=1e-10 dE=0.00246026 E=6.58956e-19
2023-11-06 14:23:59 magnum.np:INFO [LLG] relax: t=1.1e-10 dE=0.00221527 E=6.575e-19
2023-11-06 14:23:59 magnum.np:INFO [LLG] relax: t=1.2e-10 dE=0.00202292 E=6.56172e-19
2023-11-06 14:23:59 magnum.np:INFO [LLG] relax: t=1.3e-10 dE=0.00186731 E=6.54949e-19
2023-11-06 14:23:59 magnum.np:INFO [LLG] relax: t=1.4e-10 dE=0.00173885 E=6.53812e-19
2023-11-06 14:23:59 magnum.np:INFO [LLG] relax: t=1.5e-10 dE=0.00163121 E=6.52748e-19
2023-11-06 14:23:59 magnum.np:INFO [LLG] relax: t=1.6e-10 dE=0.00153991 E=6.51744e-19
2023-11-06 14:23:59 magnum.np:INFO [LLG] relax: t=1.7e-10 dE=0.0014616 E=6.50793e-19
2023-11-06 14:23:59 magnum.np:INFO [LLG] relax: t=1.8e-10 dE=0.00139366 E=6.49887e-19
2023-11-06 14:23:59 magnum.np:INFO [LLG] relax: t=1.9e-10 dE=0.00133399 E=6.49021e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=2e-10 dE=0.00128089 E=6.48191e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=2.1e-10 dE=0.00123297 E=6.47393e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=2.2e-10 dE=0.0011891 E=6.46624e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=2.3e-10 dE=0.00114834 E=6.45882e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=2.4e-10 dE=0.00110994 E=6.45166e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=2.5e-10 dE=0.00107331 E=6.44474e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=2.6e-10 dE=0.00103798 E=6.43806e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=2.7e-10 dE=0.00100357 E=6.43161e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=2.8e-10 dE=0.000969828 E=6.42537e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=2.9e-10 dE=0.000936556 E=6.41936e-19
2023-11-06 14:24:00 magnum.np:INFO [LLG] relax: t=3e-10 dE=0.000903626 E=6.41357e-19
2023-11-06 14:24:01 magnum.np:INFO [LLG] relax: t=3.1e-10 dE=0.000870966 E=6.40799e-19
2023-11-06 14:24:01 magnum.np:INFO [LLG] relax: t=3.2e-10 dE=0.000838534 E=6.40262e-19
2023-11-06 14:24:01 magnum.np:INFO [LLG] relax: t=3.3e-10 dE=0.000806336 E=6.39746e-19
2023-11-06 14:24:01 magnum.np:INFO [LLG] relax: t=3.4e-10 dE=0.000774395 E=6.39251e-19
2023-11-06 14:24:01 magnum.np:INFO [LLG] relax: t=3.5e-10 dE=0.000742751 E=6.38776e-19
2023-11-06 14:24:01 magnum.np:INFO [LLG] relax: t=3.6e-10 dE=0.000711464 E=6.38322e-19
2023-11-06 14:24:01 magnum.np:INFO [LLG] relax: t=3.7e-10 dE=0.000680588 E=6.37888e-19
2023-11-06 14:24:01 magnum.np:INFO [LLG] relax: t=3.8e-10 dE=0.000650197 E=6.37474e-19
2023-11-06 14:24:01 magnum.np:INFO [LLG] relax: t=3.9e-10 dE=0.000620354 E=6.37078e-19
2023-11-06 14:24:02 magnum.np:INFO [LLG] relax: t=4e-10 dE=0.000591127 E=6.36702e-19
2023-11-06 14:24:02 magnum.np:INFO [LLG] relax: t=4.1e-10 dE=0.000562576 E=6.36344e-19
2023-11-06 14:24:02 magnum.np:INFO [LLG] relax: t=4.2e-10 dE=0.000534755 E=6.36004e-19
2023-11-06 14:24:02 magnum.np:INFO [LLG] relax: t=4.3e-10 dE=0.000507712 E=6.35681e-19
2023-11-06 14:24:02 magnum.np:INFO [LLG] relax: t=4.4e-10 dE=0.000481493 E=6.35375e-19
2023-11-06 14:24:02 magnum.np:INFO [LLG] relax: t=4.5e-10 dE=0.000456131 E=6.35086e-19
2023-11-06 14:24:02 magnum.np:INFO [LLG] relax: t=4.6e-10 dE=0.000431654 E=6.34812e-19
2023-11-06 14:24:02 magnum.np:INFO [LLG] relax: t=4.7e-10 dE=0.000408082 E=6.34553e-19
2023-11-06 14:24:02 magnum.np:INFO [LLG] relax: t=4.8e-10 dE=0.000385428 E=6.34308e-19
2023-11-06 14:24:02 magnum.np:INFO [LLG] relax: t=4.9e-10 dE=0.000363702 E=6.34077e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=5e-10 dE=0.000342899 E=6.3386e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=5.1e-10 dE=0.000323022 E=6.33655e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=5.2e-10 dE=0.000304056 E=6.33463e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=5.3e-10 dE=0.000285989 E=6.33282e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=5.4e-10 dE=0.000268813 E=6.33112e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=5.5e-10 dE=0.000252491 E=6.32952e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=5.6e-10 dE=0.000237012 E=6.32802e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=5.7e-10 dE=0.000222349 E=6.32661e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=5.8e-10 dE=0.000208472 E=6.32529e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=5.9e-10 dE=0.000195358 E=6.32406e-19
2023-11-06 14:24:03 magnum.np:INFO [LLG] relax: t=6e-10 dE=0.000182974 E=6.3229e-19
2023-11-06 14:24:04 magnum.np:INFO [LLG] relax: t=6.1e-10 dE=0.000171292 E=6.32182e-19
2023-11-06 14:24:04 magnum.np:INFO [LLG] relax: t=6.2e-10 dE=0.000160284 E=6.3208e-19
2023-11-06 14:24:04 magnum.np:INFO [LLG] relax: t=6.3e-10 dE=0.000149917 E=6.31986e-19
2023-11-06 14:24:04 magnum.np:INFO [LLG] relax: t=6.4e-10 dE=0.000140162 E=6.31897e-19
2023-11-06 14:24:04 magnum.np:INFO [LLG] relax: t=6.5e-10 dE=0.000130998 E=6.31814e-19
2023-11-06 14:24:04 magnum.np:INFO [LLG] relax: t=6.6e-10 dE=0.00012238 E=6.31737e-19
2023-11-06 14:24:04 magnum.np:INFO [LLG] relax: t=6.7e-10 dE=0.00011429 E=6.31665e-19
2023-11-06 14:24:04 magnum.np:INFO [LLG] relax: t=6.8e-10 dE=0.000106705 E=6.31597e-19
2023-11-06 14:24:04 magnum.np:INFO [LLG] relax: t=6.9e-10 dE=9.95866e-05 E=6.31535e-19
2023-11-06 14:24:04 magnum.np:INFO [LLG] relax: t=7e-10 dE=9.29203e-05 E=6.31476e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=7.1e-10 dE=8.66724e-05 E=6.31421e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=7.2e-10 dE=8.08249e-05 E=6.3137e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=7.3e-10 dE=7.53497e-05 E=6.31323e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=7.4e-10 dE=7.0235e-05 E=6.31278e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=7.5e-10 dE=6.54502e-05 E=6.31237e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=7.6e-10 dE=6.09752e-05 E=6.31198e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=7.7e-10 dE=5.67971e-05 E=6.31163e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=7.8e-10 dE=5.28934e-05 E=6.31129e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=7.9e-10 dE=4.92528e-05 E=6.31098e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=8e-10 dE=4.58498e-05 E=6.31069e-19
2023-11-06 14:24:05 magnum.np:INFO [LLG] relax: t=8.1e-10 dE=4.26779e-05 E=6.31042e-19
2023-11-06 14:24:06 magnum.np:INFO [LLG] relax: t=8.2e-10 dE=3.97174e-05 E=6.31017e-19
2023-11-06 14:24:06 magnum.np:INFO [LLG] relax: t=8.3e-10 dE=3.69585e-05 E=6.30994e-19
2023-11-06 14:24:06 magnum.np:INFO [LLG] relax: t=8.4e-10 dE=3.4384e-05 E=6.30972e-19
2023-11-06 14:24:06 magnum.np:INFO [LLG] relax: t=8.5e-10 dE=3.19882e-05 E=6.30952e-19
2023-11-06 14:24:06 magnum.np:INFO [LLG] relax: t=8.6e-10 dE=2.9751e-05 E=6.30933e-19
2023-11-06 14:24:06 magnum.np:INFO [LLG] relax: t=8.7e-10 dE=2.76699e-05 E=6.30916e-19
2023-11-06 14:24:06 magnum.np:INFO [LLG] relax: t=8.8e-10 dE=2.57294e-05 E=6.30899e-19
2023-11-06 14:24:06 magnum.np:INFO [LLG] relax: t=8.9e-10 dE=2.39234e-05 E=6.30884e-19
2023-11-06 14:24:06 magnum.np:INFO [LLG] relax: t=9e-10 dE=2.2242e-05 E=6.3087e-19
2023-11-06 14:24:06 magnum.np:INFO [LLG] relax: t=9.1e-10 dE=2.06762e-05 E=6.30857e-19
2023-11-06 14:24:07 magnum.np:INFO [LLG] relax: t=9.2e-10 dE=1.92184e-05 E=6.30845e-19
2023-11-06 14:24:07 magnum.np:INFO [LLG] relax: t=9.3e-10 dE=1.78631e-05 E=6.30834e-19
2023-11-06 14:24:07 magnum.np:INFO [LLG] relax: t=9.4e-10 dE=1.65992e-05 E=6.30823e-19
2023-11-06 14:24:07 magnum.np:INFO [LLG] relax: t=9.5e-10 dE=1.54274e-05 E=6.30814e-19
2023-11-06 14:24:07 magnum.np:INFO [LLG] relax: t=9.6e-10 dE=1.4333e-05 E=6.30805e-19
2023-11-06 14:24:07 magnum.np:INFO [LLG] relax: t=9.7e-10 dE=1.33177e-05 E=6.30796e-19
2023-11-06 14:24:07 magnum.np:INFO [LLG] relax: t=9.8e-10 dE=1.23722e-05 E=6.30788e-19
2023-11-06 14:24:07 magnum.np:INFO [LLG] relax: t=9.9e-10 dE=1.14944e-05 E=6.30781e-19
2023-11-06 14:24:07 magnum.np:INFO [LLG] relax: t=1e-09 dE=1.06767e-05 E=6.30774e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.01e-09 dE=9.91608e-06 E=6.30768e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.02e-09 dE=9.21307e-06 E=6.30762e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.03e-09 dE=8.55403e-06 E=6.30757e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.04e-09 dE=7.94488e-06 E=6.30752e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.05e-09 dE=7.37734e-06 E=6.30747e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.06e-09 dE=6.8509e-06 E=6.30743e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.07e-09 dE=6.36187e-06 E=6.30739e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.08e-09 dE=5.90072e-06 E=6.30735e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.09e-09 dE=5.49059e-06 E=6.30732e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.1e-09 dE=5.09101e-06 E=6.30729e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.11e-09 dE=4.72604e-06 E=6.30726e-19
2023-11-06 14:24:08 magnum.np:INFO [LLG] relax: t=1.12e-09 dE=4.38809e-06 E=6.30723e-19
2023-11-06 14:24:09 magnum.np:INFO [LLG] relax: t=1.13e-09 dE=4.07336e-06 E=6.3072e-19
2023-11-06 14:24:09 magnum.np:INFO [LLG] relax: t=1.14e-09 dE=3.7808e-06 E=6.30718e-19
2023-11-06 14:24:09 magnum.np:INFO [LLG] relax: t=1.15e-09 dE=3.50941e-06 E=6.30716e-19
2023-11-06 14:24:09 magnum.np:INFO [LLG] relax: t=1.16e-09 dE=3.25893e-06 E=6.30714e-19
2023-11-06 14:24:09 magnum.np:INFO [LLG] relax: t=1.17e-09 dE=3.02325e-06 E=6.30712e-19
2023-11-06 14:24:09 magnum.np:INFO [LLG] relax: t=1.18e-09 dE=2.80649e-06 E=6.3071e-19
2023-11-06 14:24:09 magnum.np:INFO [LLG] relax: t=1.19e-09 dE=2.59762e-06 E=6.30708e-19
2023-11-06 14:24:09 magnum.np:INFO [LLG] relax: t=1.2e-09 dE=2.42423e-06 E=6.30707e-19
2023-11-06 14:24:09 magnum.np:INFO [LLG] relax: t=1.21e-09 dE=2.24335e-06 E=6.30705e-19
2023-11-06 14:24:09 magnum.np:INFO [LLG] relax: t=1.22e-09 dE=2.08069e-06 E=6.30704e-19
2023-11-06 14:24:10 magnum.np:INFO [LLG] relax: t=1.23e-09 dE=1.93274e-06 E=6.30703e-19
2023-11-06 14:24:10 magnum.np:INFO [LLG] relax: t=1.24e-09 dE=1.79282e-06 E=6.30702e-19
2023-11-06 14:24:10 magnum.np:INFO [LLG] relax: t=1.25e-09 dE=1.6626e-06 E=6.30701e-19
2023-11-06 14:24:10 magnum.np:INFO [LLG] relax: t=1.26e-09 dE=1.54594e-06 E=6.307e-19
2023-11-06 14:24:10 magnum.np:INFO [LLG] relax: t=1.27e-09 dE=1.43201e-06 E=6.30699e-19
2023-11-06 14:24:10 magnum.np:INFO [LLG] relax: t=1.28e-09 dE=1.3289e-06 E=6.30698e-19
2023-11-06 14:24:10 magnum.np:INFO [LLG] relax: t=1.29e-09 dE=1.23514e-06 E=6.30697e-19
2023-11-06 14:24:10 magnum.np:INFO [LLG] relax: t=1.3e-09 dE=1.144e-06 E=6.30696e-19
2023-11-06 14:24:10 magnum.np:INFO [LLG] relax: t=1.31e-09 dE=1.0623e-06 E=6.30696e-19
2023-11-06 14:24:10 magnum.np:INFO [LLG] relax: t=1.32e-09 dE=9.85183e-07 E=6.30695e-19
2023-11-06 14:24:10 magnum.np:INFO [LLGSolver] using RKF45 solver (atol = 1e-05)
2023-11-06 14:24:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1e-11
2023-11-06 14:24:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=2e-11
2023-11-06 14:24:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=3e-11
2023-11-06 14:24:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=4e-11
2023-11-06 14:24:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=5e-11
2023-11-06 14:24:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=6e-11
2023-11-06 14:24:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=7e-11
2023-11-06 14:24:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=8e-11
2023-11-06 14:24:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=9e-11
2023-11-06 14:24:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1e-10
2023-11-06 14:24:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.1e-10
2023-11-06 14:24:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.2e-10
2023-11-06 14:24:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.3e-10
2023-11-06 14:24:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.4e-10
2023-11-06 14:24:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.5e-10
2023-11-06 14:24:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.6e-10
2023-11-06 14:24:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.7e-10
2023-11-06 14:24:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.8e-10
2023-11-06 14:24:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.9e-10
2023-11-06 14:24:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=2e-10
2023-11-06 14:24:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.1e-10
2023-11-06 14:24:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.2e-10
2023-11-06 14:24:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.3e-10
2023-11-06 14:24:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.4e-10
2023-11-06 14:24:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.5e-10
2023-11-06 14:24:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.6e-10
2023-11-06 14:24:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.7e-10
2023-11-06 14:24:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.8e-10
2023-11-06 14:24:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.9e-10
2023-11-06 14:24:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=3e-10
2023-11-06 14:24:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.1e-10
2023-11-06 14:24:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.2e-10
2023-11-06 14:24:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.3e-10
2023-11-06 14:24:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.4e-10
2023-11-06 14:24:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.5e-10
2023-11-06 14:24:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.6e-10
2023-11-06 14:24:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.7e-10
2023-11-06 14:24:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.8e-10
2023-11-06 14:24:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.9e-10
2023-11-06 14:24:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=4e-10
2023-11-06 14:24:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.1e-10
2023-11-06 14:24:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.2e-10
2023-11-06 14:24:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.3e-10
2023-11-06 14:24:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.4e-10
2023-11-06 14:24:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.5e-10
2023-11-06 14:24:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.6e-10
2023-11-06 14:24:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.7e-10
2023-11-06 14:24:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.8e-10
2023-11-06 14:24:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.9e-10
2023-11-06 14:24:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=5e-10
2023-11-06 14:24:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.1e-10
2023-11-06 14:24:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.2e-10
2023-11-06 14:24:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.3e-10
2023-11-06 14:24:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.4e-10
2023-11-06 14:24:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.5e-10
2023-11-06 14:24:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.6e-10
2023-11-06 14:24:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.7e-10
2023-11-06 14:24:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.8e-10
2023-11-06 14:24:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.9e-10
2023-11-06 14:24:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=6e-10
2023-11-06 14:24:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.1e-10
2023-11-06 14:24:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.2e-10
2023-11-06 14:24:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.3e-10
2023-11-06 14:24:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.4e-10
2023-11-06 14:24:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.5e-10
2023-11-06 14:24:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.6e-10
2023-11-06 14:24:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.7e-10
2023-11-06 14:24:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.8e-10
2023-11-06 14:24:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.9e-10
2023-11-06 14:24:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=7e-10
2023-11-06 14:24:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.1e-10
2023-11-06 14:24:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.2e-10
2023-11-06 14:24:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.3e-10
2023-11-06 14:24:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.4e-10
2023-11-06 14:24:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.5e-10
2023-11-06 14:24:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.6e-10
2023-11-06 14:24:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.7e-10
2023-11-06 14:24:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.8e-10
2023-11-06 14:24:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.9e-10
2023-11-06 14:24:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8e-10
2023-11-06 14:24:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.1e-10
2023-11-06 14:24:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.2e-10
2023-11-06 14:24:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.3e-10
2023-11-06 14:24:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.4e-10
2023-11-06 14:24:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.5e-10
2023-11-06 14:24:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.6e-10
2023-11-06 14:24:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.7e-10
2023-11-06 14:24:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.8e-10
2023-11-06 14:24:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.9e-10
2023-11-06 14:24:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=9e-10
2023-11-06 14:24:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.1e-10
2023-11-06 14:24:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.2e-10
2023-11-06 14:24:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.3e-10
2023-11-06 14:24:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.4e-10
2023-11-06 14:24:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.5e-10
2023-11-06 14:24:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.6e-10
2023-11-06 14:24:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.7e-10
2023-11-06 14:24:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.8e-10
2023-11-06 14:24:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.9e-10
2023-11-06 14:24:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1e-09
====================================================================================
TIMER REPORT
====================================================================================
Operation No of calls Avg time [ms] Total time [s] Memory [MB]
------------------- ------------- --------------- ---------------- -------------
LLGSolver.relax 1 13356.2 13.3562 6.88672
DemagField.h 5131 1.29731 6.65649 6.82812
ExchangeField.h 5131 0.70471 3.61587 0
LLGSolver.step 100 127.107 12.7107 1.52734
DemagField.h 4788 1.19447 5.71914 1.52734
ExchangeField.h 4788 0.664887 3.18348 0
ExternalField.h 4788 0.0634773 0.30393 0
------------------- ------------- --------------- ---------------- -------------
Total 26.1452
Missing 0.0782211
====================================================================================
Plot Results:
[4]:
from os import path
if not path.isdir("ref"):
!mkdir ref
!wget -P ref https://gitlab.com/magnum.np/magnum.np/raw/main/demos/sp4/ref/m.dat
[5]:
#TODO: read latest script content from gitlab repository, as soon as %load works with Colab
import numpy as np
import matplotlib.pyplot as plt
data = np.loadtxt("data/log.dat")
ref = np.loadtxt("ref/m.dat")
fig, ax = plt.subplots(figsize=(15, 5))
cycle = plt.rcParams['axes.prop_cycle'].by_key()['color']
ax.plot(data[:,0]*1e9, data[:,1], '-', color = cycle[0], label = "magnum.np - x")
ax.plot(ref[:,0]*1e9, ref[:,1], '-', color = cycle[0], linewidth = 6, alpha = 0.4, label = "reference - x")
ax.plot(data[:,0]*1e9, data[:,2], '-', color = cycle[1], label = "magnum.np - y")
ax.plot(ref[:,0]*1e9, ref[:,2], '-', color = cycle[1], linewidth = 6, alpha = 0.4, label = "reference - y")
ax.plot(data[:,0]*1e9, data[:,3], '-', color = cycle[2], label = "magnum.np - z")
ax.plot(ref[:,0]*1e9, ref[:,3], '-', color = cycle[2], linewidth = 6, alpha = 0.4, label = "reference - z")
ax.set_xlim([0,1])
ax.set_xlabel("Time t[ns]")
ax.set_ylabel("Magnetization $m$")
ax.legend(ncol=3)
ax.grid()
fig.savefig("data/results.png")
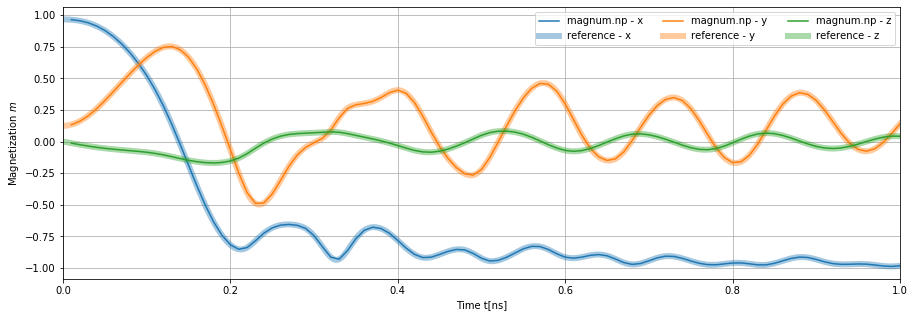