Standard Problem for Ferromagnetic Resonance Simulation
Google Colab Link
The demo can be run on Google Colab without any local installation. Use the following link to try it out.
[1]:
!pip install -q magnumnp numpy==1.22.4
Run Demo:
[2]:
from magnumnp import *
import torch
from math import sin, cos, pi
Timer.enable()
# initialize mesh
eps = 1e-15
n = (24, 24, 2)
l = (120e-9, 120e-9, 10e-9)
dx = (l[0]/n[0], l[1]/n[1], l[2]/n[2])
origin = (-n[0]*dx[0]/2., -n[1]*dx[1]/2., -n[2]*dx[2]/2.)
mesh = Mesh(n, dx, origin=origin)
# initialize state
state = State(mesh)
state.material = {
"alpha": 1.,
"Ms": 800e3,
"A": 13e-12
}
state.m = state.Constant([0, 0, 1])
#relax state
demag = DemagField()
exchange = ExchangeField()
bias = ExternalField(80e3*state.Tensor([1., 0.715, 0]).normalize())
llg = LLGSolver([demag, exchange, bias])
llg.relax(state)
write_vti(state.m, "data/m0.vti")
#integrate
state.t = 0.0
state.material["alpha"] = 0.008
bias = ExternalField(80e3*state.Tensor([1., 0.7, 0]).normalize())
llg = LLGSolver([demag, exchange, bias])
logger = Logger("data", ['t', 'm'], ["m"])
while state.t < 10e-9-eps:
llg.step(state, 5e-12)
logger << state
Timer.print_report()
2023-05-08 12:48:13 magnum.np:INFO magnum.np 1.0.9
2023-05-08 12:48:13 magnum.np:INFO [State] running on device: cpu (dtype = float64)
2023-05-08 12:48:13 magnum.np:INFO [Mesh] 24x24x2 (size= 5e-09 x 5e-09 x 5e-09)
2023-05-08 12:48:14 magnum.np:INFO [LLGSolver] using RKF45 solver (atol = 1e-05)
2023-05-08 12:48:37 magnum.np:INFO [DEMAG]: Time calculation of demag kernel = 23.676497220993042 s
2023-05-08 12:49:04 magnum.np:INFO [LLG] relax: t=1e-11 dE=0.0479888 E=4.57608e-17
2023-05-08 12:49:04 magnum.np:INFO [LLG] relax: t=2e-11 dE=0.180908 E=3.87505e-17
2023-05-08 12:49:04 magnum.np:INFO [LLG] relax: t=3e-11 dE=0.504086 E=2.57635e-17
2023-05-08 12:49:05 magnum.np:INFO [LLG] relax: t=4e-11 dE=0.595849 E=1.61441e-17
2023-05-08 12:49:05 magnum.np:INFO [LLG] relax: t=5e-11 dE=0.300941 E=1.24095e-17
2023-05-08 12:49:05 magnum.np:INFO [LLG] relax: t=6e-11 dE=0.137214 E=1.09122e-17
2023-05-08 12:49:05 magnum.np:INFO [LLG] relax: t=7e-11 dE=0.123262 E=9.71477e-18
2023-05-08 12:49:06 magnum.np:INFO [LLG] relax: t=8e-11 dE=0.1693 E=8.30819e-18
2023-05-08 12:49:06 magnum.np:INFO [LLG] relax: t=9e-11 dE=0.243295 E=6.6824e-18
2023-05-08 12:49:06 magnum.np:INFO [LLG] relax: t=1e-10 dE=0.348205 E=4.95651e-18
2023-05-08 12:49:07 magnum.np:INFO [LLG] relax: t=1.1e-10 dE=0.527855 E=3.2441e-18
2023-05-08 12:49:07 magnum.np:INFO [LLG] relax: t=1.2e-10 dE=1.00216 E=1.6203e-18
2023-05-08 12:49:07 magnum.np:INFO [LLG] relax: t=1.3e-10 dE=11.3816 E=1.30864e-19
2023-05-08 12:49:07 magnum.np:INFO [LLG] relax: t=1.4e-10 dE=1.10986 E=-1.19115e-18
2023-05-08 12:49:08 magnum.np:INFO [LLG] relax: t=1.5e-10 dE=0.485691 E=-2.31601e-18
2023-05-08 12:49:08 magnum.np:INFO [LLG] relax: t=1.6e-10 dE=0.282741 E=-3.22897e-18
2023-05-08 12:49:08 magnum.np:INFO [LLG] relax: t=1.7e-10 dE=0.18195 E=-3.94716e-18
2023-05-08 12:49:09 magnum.np:INFO [LLG] relax: t=1.8e-10 dE=0.125674 E=-4.51451e-18
2023-05-08 12:49:09 magnum.np:INFO [LLG] relax: t=1.9e-10 dE=0.0937003 E=-4.98126e-18
2023-05-08 12:49:09 magnum.np:INFO [LLG] relax: t=2e-10 dE=0.0753759 E=-5.38733e-18
2023-05-08 12:49:10 magnum.np:INFO [LLG] relax: t=2.1e-10 dE=0.0639119 E=-5.75516e-18
2023-05-08 12:49:10 magnum.np:INFO [LLG] relax: t=2.2e-10 dE=0.0548782 E=-6.08933e-18
2023-05-08 12:49:10 magnum.np:INFO [LLG] relax: t=2.3e-10 dE=0.045887 E=-6.38219e-18
2023-05-08 12:49:11 magnum.np:INFO [LLG] relax: t=2.4e-10 dE=0.0364353 E=-6.62352e-18
2023-05-08 12:49:11 magnum.np:INFO [LLG] relax: t=2.5e-10 dE=0.0272359 E=-6.80897e-18
2023-05-08 12:49:11 magnum.np:INFO [LLG] relax: t=2.6e-10 dE=0.0192283 E=-6.94246e-18
2023-05-08 12:49:12 magnum.np:INFO [LLG] relax: t=2.7e-10 dE=0.0129387 E=-7.03346e-18
2023-05-08 12:49:12 magnum.np:INFO [LLG] relax: t=2.8e-10 dE=0.0083927 E=-7.09299e-18
2023-05-08 12:49:12 magnum.np:INFO [LLG] relax: t=2.9e-10 dE=0.00530992 E=-7.13086e-18
2023-05-08 12:49:13 magnum.np:INFO [LLG] relax: t=3e-10 dE=0.00331309 E=-7.15456e-18
2023-05-08 12:49:13 magnum.np:INFO [LLG] relax: t=3.1e-10 dE=0.0020566 E=-7.1693e-18
2023-05-08 12:49:13 magnum.np:INFO [LLG] relax: t=3.2e-10 dE=0.00127704 E=-7.17847e-18
2023-05-08 12:49:14 magnum.np:INFO [LLG] relax: t=3.3e-10 dE=0.000794932 E=-7.18418e-18
2023-05-08 12:49:14 magnum.np:INFO [LLG] relax: t=3.4e-10 dE=0.000496029 E=-7.18775e-18
2023-05-08 12:49:14 magnum.np:INFO [LLG] relax: t=3.5e-10 dE=0.000309987 E=-7.18998e-18
2023-05-08 12:49:15 magnum.np:INFO [LLG] relax: t=3.6e-10 dE=0.000193864 E=-7.19137e-18
2023-05-08 12:49:15 magnum.np:INFO [LLG] relax: t=3.7e-10 dE=0.000121301 E=-7.19224e-18
2023-05-08 12:49:15 magnum.np:INFO [LLG] relax: t=3.8e-10 dE=7.59595e-05 E=-7.19279e-18
2023-05-08 12:49:16 magnum.np:INFO [LLG] relax: t=3.9e-10 dE=4.76358e-05 E=-7.19313e-18
2023-05-08 12:49:16 magnum.np:INFO [LLG] relax: t=4e-10 dE=2.99393e-05 E=-7.19335e-18
2023-05-08 12:49:17 magnum.np:INFO [LLG] relax: t=4.1e-10 dE=1.88697e-05 E=-7.19348e-18
2023-05-08 12:49:17 magnum.np:INFO [LLG] relax: t=4.2e-10 dE=1.19298e-05 E=-7.19357e-18
2023-05-08 12:49:17 magnum.np:INFO [LLG] relax: t=4.3e-10 dE=7.56547e-06 E=-7.19362e-18
2023-05-08 12:49:17 magnum.np:INFO [LLG] relax: t=4.4e-10 dE=4.811e-06 E=-7.19366e-18
2023-05-08 12:49:18 magnum.np:INFO [LLG] relax: t=4.5e-10 dE=3.06627e-06 E=-7.19368e-18
2023-05-08 12:49:18 magnum.np:INFO [LLG] relax: t=4.6e-10 dE=1.95752e-06 E=-7.19369e-18
2023-05-08 12:49:19 magnum.np:INFO [LLG] relax: t=4.7e-10 dE=1.25105e-06 E=-7.1937e-18
2023-05-08 12:49:19 magnum.np:INFO [LLG] relax: t=4.8e-10 dE=8.00024e-07 E=-7.19371e-18
/home/nina/git/magnum.np/venv/lib/python3.8/site-packages/pyvista/core/grid.py:508: PyVistaDeprecationWarning: `dims` argument is deprecated. Please use `dimensions`.
warnings.warn(
2023-05-08 12:49:19 magnum.np:INFO [LLGSolver] using RKF45 solver (atol = 1e-05)
2023-05-08 12:49:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=5e-12
2023-05-08 12:49:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=1e-11
2023-05-08 12:49:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.5e-11
2023-05-08 12:49:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=2e-11
2023-05-08 12:49:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.5e-11
2023-05-08 12:49:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=3e-11
2023-05-08 12:49:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.5e-11
2023-05-08 12:49:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=4e-11
2023-05-08 12:49:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.5e-11
2023-05-08 12:49:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=5e-11
2023-05-08 12:49:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.5e-11
2023-05-08 12:49:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=6e-11
2023-05-08 12:49:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.5e-11
2023-05-08 12:49:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=7e-11
2023-05-08 12:49:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.5e-11
2023-05-08 12:49:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=8e-11
2023-05-08 12:49:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.5e-11
2023-05-08 12:49:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=9e-11
2023-05-08 12:49:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.5e-11
2023-05-08 12:49:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=1e-10
2023-05-08 12:49:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.05e-10
2023-05-08 12:49:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.1e-10
2023-05-08 12:49:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.15e-10
2023-05-08 12:49:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.2e-10
2023-05-08 12:49:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.25e-10
2023-05-08 12:49:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.3e-10
2023-05-08 12:49:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.35e-10
2023-05-08 12:49:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.4e-10
2023-05-08 12:49:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.45e-10
2023-05-08 12:49:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.5e-10
2023-05-08 12:49:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.55e-10
2023-05-08 12:49:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.6e-10
2023-05-08 12:49:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.65e-10
2023-05-08 12:49:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.7e-10
2023-05-08 12:49:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.75e-10
2023-05-08 12:49:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.8e-10
2023-05-08 12:49:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.85e-10
2023-05-08 12:49:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.9e-10
2023-05-08 12:49:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.95e-10
2023-05-08 12:49:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=2e-10
2023-05-08 12:49:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.05e-10
2023-05-08 12:49:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.1e-10
2023-05-08 12:49:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.15e-10
2023-05-08 12:49:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.2e-10
2023-05-08 12:49:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.25e-10
2023-05-08 12:49:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.3e-10
2023-05-08 12:49:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.35e-10
2023-05-08 12:49:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.4e-10
2023-05-08 12:49:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.45e-10
2023-05-08 12:49:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.5e-10
2023-05-08 12:49:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.55e-10
2023-05-08 12:49:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.6e-10
2023-05-08 12:49:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.65e-10
2023-05-08 12:49:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.7e-10
2023-05-08 12:49:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.75e-10
2023-05-08 12:49:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.8e-10
2023-05-08 12:49:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.85e-10
2023-05-08 12:49:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.9e-10
2023-05-08 12:49:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.95e-10
2023-05-08 12:49:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=3e-10
2023-05-08 12:49:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.05e-10
2023-05-08 12:49:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.1e-10
2023-05-08 12:49:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.15e-10
2023-05-08 12:49:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.2e-10
2023-05-08 12:49:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.25e-10
2023-05-08 12:49:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.3e-10
2023-05-08 12:49:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.35e-10
2023-05-08 12:49:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.4e-10
2023-05-08 12:49:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.45e-10
2023-05-08 12:49:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.5e-10
2023-05-08 12:49:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.55e-10
2023-05-08 12:49:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.6e-10
2023-05-08 12:49:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.65e-10
2023-05-08 12:49:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.7e-10
2023-05-08 12:49:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.75e-10
2023-05-08 12:49:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.8e-10
2023-05-08 12:49:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.85e-10
2023-05-08 12:49:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.9e-10
2023-05-08 12:49:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.95e-10
2023-05-08 12:49:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=4e-10
2023-05-08 12:49:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.05e-10
2023-05-08 12:49:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.1e-10
2023-05-08 12:49:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.15e-10
2023-05-08 12:49:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.2e-10
2023-05-08 12:49:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.25e-10
2023-05-08 12:49:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.3e-10
2023-05-08 12:49:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.35e-10
2023-05-08 12:49:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.4e-10
2023-05-08 12:49:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.45e-10
2023-05-08 12:49:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.5e-10
2023-05-08 12:49:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.55e-10
2023-05-08 12:49:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.6e-10
2023-05-08 12:49:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.65e-10
2023-05-08 12:49:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.7e-10
2023-05-08 12:49:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.75e-10
2023-05-08 12:49:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.8e-10
2023-05-08 12:49:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.85e-10
2023-05-08 12:49:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.9e-10
2023-05-08 12:49:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.95e-10
2023-05-08 12:49:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=5e-10
2023-05-08 12:49:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.05e-10
2023-05-08 12:49:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.1e-10
2023-05-08 12:49:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.15e-10
2023-05-08 12:49:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.2e-10
2023-05-08 12:49:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.25e-10
2023-05-08 12:49:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.3e-10
2023-05-08 12:49:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.35e-10
2023-05-08 12:49:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.4e-10
2023-05-08 12:49:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.45e-10
2023-05-08 12:49:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.5e-10
2023-05-08 12:49:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.55e-10
2023-05-08 12:49:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.6e-10
2023-05-08 12:49:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.65e-10
2023-05-08 12:49:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.7e-10
2023-05-08 12:49:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.75e-10
2023-05-08 12:49:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.8e-10
2023-05-08 12:49:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.85e-10
2023-05-08 12:49:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.9e-10
2023-05-08 12:49:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.95e-10
2023-05-08 12:49:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=6e-10
2023-05-08 12:49:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.05e-10
2023-05-08 12:49:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.1e-10
2023-05-08 12:49:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.15e-10
2023-05-08 12:49:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.2e-10
2023-05-08 12:49:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.25e-10
2023-05-08 12:49:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.3e-10
2023-05-08 12:49:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.35e-10
2023-05-08 12:49:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.4e-10
2023-05-08 12:49:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.45e-10
2023-05-08 12:49:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.5e-10
2023-05-08 12:50:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.55e-10
2023-05-08 12:50:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.6e-10
2023-05-08 12:50:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.65e-10
2023-05-08 12:50:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.7e-10
2023-05-08 12:50:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.75e-10
2023-05-08 12:50:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.8e-10
2023-05-08 12:50:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.85e-10
2023-05-08 12:50:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.9e-10
2023-05-08 12:50:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.95e-10
2023-05-08 12:50:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=7e-10
2023-05-08 12:50:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.05e-10
2023-05-08 12:50:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.1e-10
2023-05-08 12:50:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.15e-10
2023-05-08 12:50:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.2e-10
2023-05-08 12:50:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.25e-10
2023-05-08 12:50:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.3e-10
2023-05-08 12:50:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.35e-10
2023-05-08 12:50:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.4e-10
2023-05-08 12:50:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.45e-10
2023-05-08 12:50:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.5e-10
2023-05-08 12:50:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.55e-10
2023-05-08 12:50:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.6e-10
2023-05-08 12:50:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.65e-10
2023-05-08 12:50:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.7e-10
2023-05-08 12:50:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.75e-10
2023-05-08 12:50:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.8e-10
2023-05-08 12:50:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.85e-10
2023-05-08 12:50:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.9e-10
2023-05-08 12:50:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.95e-10
2023-05-08 12:50:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=8e-10
2023-05-08 12:50:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.05e-10
2023-05-08 12:50:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.1e-10
2023-05-08 12:50:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.15e-10
2023-05-08 12:50:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.2e-10
2023-05-08 12:50:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.25e-10
2023-05-08 12:50:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.3e-10
2023-05-08 12:50:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.35e-10
2023-05-08 12:50:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.4e-10
2023-05-08 12:50:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.45e-10
2023-05-08 12:50:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.5e-10
2023-05-08 12:50:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.55e-10
2023-05-08 12:50:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.6e-10
2023-05-08 12:50:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.65e-10
2023-05-08 12:50:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.7e-10
2023-05-08 12:50:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.75e-10
2023-05-08 12:50:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.8e-10
2023-05-08 12:50:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.85e-10
2023-05-08 12:50:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.9e-10
2023-05-08 12:50:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.95e-10
2023-05-08 12:50:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=9e-10
2023-05-08 12:50:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.05e-10
2023-05-08 12:50:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.1e-10
2023-05-08 12:50:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.15e-10
2023-05-08 12:50:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.2e-10
2023-05-08 12:50:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.25e-10
2023-05-08 12:50:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.3e-10
2023-05-08 12:50:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.35e-10
2023-05-08 12:50:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.4e-10
2023-05-08 12:50:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.45e-10
2023-05-08 12:50:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.5e-10
2023-05-08 12:50:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.55e-10
2023-05-08 12:50:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.6e-10
2023-05-08 12:50:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.65e-10
2023-05-08 12:50:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.7e-10
2023-05-08 12:50:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.75e-10
2023-05-08 12:50:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.8e-10
2023-05-08 12:50:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.85e-10
2023-05-08 12:50:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.9e-10
2023-05-08 12:50:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.95e-10
2023-05-08 12:50:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=1e-09
2023-05-08 12:50:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.005e-09
2023-05-08 12:50:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.01e-09
2023-05-08 12:50:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.015e-09
2023-05-08 12:50:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.02e-09
2023-05-08 12:50:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.025e-09
2023-05-08 12:50:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.03e-09
2023-05-08 12:50:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.035e-09
2023-05-08 12:50:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.04e-09
2023-05-08 12:50:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.045e-09
2023-05-08 12:50:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.05e-09
2023-05-08 12:50:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.055e-09
2023-05-08 12:50:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.06e-09
2023-05-08 12:50:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.065e-09
2023-05-08 12:50:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.07e-09
2023-05-08 12:50:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.075e-09
2023-05-08 12:50:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.08e-09
2023-05-08 12:50:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.085e-09
2023-05-08 12:50:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.09e-09
2023-05-08 12:50:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.095e-09
2023-05-08 12:50:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.1e-09
2023-05-08 12:50:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.105e-09
2023-05-08 12:50:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.11e-09
2023-05-08 12:50:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.115e-09
2023-05-08 12:50:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.12e-09
2023-05-08 12:50:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.125e-09
2023-05-08 12:50:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.13e-09
2023-05-08 12:50:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.135e-09
2023-05-08 12:50:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.14e-09
2023-05-08 12:50:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.145e-09
2023-05-08 12:50:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.15e-09
2023-05-08 12:50:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.155e-09
2023-05-08 12:50:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.16e-09
2023-05-08 12:50:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.165e-09
2023-05-08 12:50:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.17e-09
2023-05-08 12:50:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.175e-09
2023-05-08 12:50:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.18e-09
2023-05-08 12:50:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.185e-09
2023-05-08 12:50:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.19e-09
2023-05-08 12:50:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.195e-09
2023-05-08 12:50:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.2e-09
2023-05-08 12:50:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.205e-09
2023-05-08 12:50:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.21e-09
2023-05-08 12:50:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.215e-09
2023-05-08 12:50:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.22e-09
2023-05-08 12:50:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.225e-09
2023-05-08 12:50:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.23e-09
2023-05-08 12:50:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.235e-09
2023-05-08 12:50:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.24e-09
2023-05-08 12:50:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.245e-09
2023-05-08 12:50:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.25e-09
2023-05-08 12:50:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.255e-09
2023-05-08 12:50:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.26e-09
2023-05-08 12:50:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.265e-09
2023-05-08 12:50:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.27e-09
2023-05-08 12:50:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.275e-09
2023-05-08 12:50:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.28e-09
2023-05-08 12:50:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.285e-09
2023-05-08 12:50:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.29e-09
2023-05-08 12:50:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.295e-09
2023-05-08 12:50:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.3e-09
2023-05-08 12:50:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.305e-09
2023-05-08 12:50:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.31e-09
2023-05-08 12:50:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.315e-09
2023-05-08 12:50:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.32e-09
2023-05-08 12:50:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.325e-09
2023-05-08 12:50:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.33e-09
2023-05-08 12:50:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.335e-09
2023-05-08 12:50:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.34e-09
2023-05-08 12:50:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.345e-09
2023-05-08 12:50:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.35e-09
2023-05-08 12:50:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.355e-09
2023-05-08 12:50:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.36e-09
2023-05-08 12:50:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.365e-09
2023-05-08 12:50:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.37e-09
2023-05-08 12:50:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.375e-09
2023-05-08 12:50:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.38e-09
2023-05-08 12:50:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.385e-09
2023-05-08 12:50:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.39e-09
2023-05-08 12:50:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.395e-09
2023-05-08 12:50:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.4e-09
2023-05-08 12:50:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.405e-09
2023-05-08 12:50:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.41e-09
2023-05-08 12:50:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.415e-09
2023-05-08 12:50:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.42e-09
2023-05-08 12:50:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.425e-09
2023-05-08 12:50:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.43e-09
2023-05-08 12:50:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.435e-09
2023-05-08 12:50:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.44e-09
2023-05-08 12:50:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.445e-09
2023-05-08 12:50:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.45e-09
2023-05-08 12:50:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.455e-09
2023-05-08 12:50:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.46e-09
2023-05-08 12:50:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.465e-09
2023-05-08 12:50:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.47e-09
2023-05-08 12:50:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.475e-09
2023-05-08 12:50:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.48e-09
2023-05-08 12:50:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.485e-09
2023-05-08 12:50:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.49e-09
2023-05-08 12:50:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.495e-09
2023-05-08 12:50:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.5e-09
2023-05-08 12:50:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.505e-09
2023-05-08 12:50:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.51e-09
2023-05-08 12:50:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.515e-09
2023-05-08 12:50:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.52e-09
2023-05-08 12:50:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.525e-09
2023-05-08 12:50:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.53e-09
2023-05-08 12:50:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.535e-09
2023-05-08 12:50:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.54e-09
2023-05-08 12:50:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.545e-09
2023-05-08 12:50:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.55e-09
2023-05-08 12:50:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.555e-09
2023-05-08 12:50:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.56e-09
2023-05-08 12:50:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.565e-09
2023-05-08 12:50:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.57e-09
2023-05-08 12:50:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.575e-09
2023-05-08 12:50:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.58e-09
2023-05-08 12:50:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.585e-09
2023-05-08 12:50:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.59e-09
2023-05-08 12:50:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.595e-09
2023-05-08 12:50:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.6e-09
2023-05-08 12:51:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.605e-09
2023-05-08 12:51:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.61e-09
2023-05-08 12:51:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.615e-09
2023-05-08 12:51:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.62e-09
2023-05-08 12:51:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.625e-09
2023-05-08 12:51:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.63e-09
2023-05-08 12:51:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.635e-09
2023-05-08 12:51:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.64e-09
2023-05-08 12:51:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.645e-09
2023-05-08 12:51:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.65e-09
2023-05-08 12:51:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.655e-09
2023-05-08 12:51:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.66e-09
2023-05-08 12:51:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.665e-09
2023-05-08 12:51:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.67e-09
2023-05-08 12:51:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.675e-09
2023-05-08 12:51:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.68e-09
2023-05-08 12:51:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.685e-09
2023-05-08 12:51:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.69e-09
2023-05-08 12:51:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.695e-09
2023-05-08 12:51:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.7e-09
2023-05-08 12:51:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.705e-09
2023-05-08 12:51:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.71e-09
2023-05-08 12:51:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.715e-09
2023-05-08 12:51:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.72e-09
2023-05-08 12:51:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.725e-09
2023-05-08 12:51:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.73e-09
2023-05-08 12:51:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.735e-09
2023-05-08 12:51:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.74e-09
2023-05-08 12:51:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.745e-09
2023-05-08 12:51:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.75e-09
2023-05-08 12:51:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.755e-09
2023-05-08 12:51:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.76e-09
2023-05-08 12:51:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.765e-09
2023-05-08 12:51:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.77e-09
2023-05-08 12:51:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.775e-09
2023-05-08 12:51:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.78e-09
2023-05-08 12:51:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.785e-09
2023-05-08 12:51:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.79e-09
2023-05-08 12:51:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.795e-09
2023-05-08 12:51:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.8e-09
2023-05-08 12:51:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.805e-09
2023-05-08 12:51:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.81e-09
2023-05-08 12:51:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.815e-09
2023-05-08 12:51:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.82e-09
2023-05-08 12:51:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.825e-09
2023-05-08 12:51:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.83e-09
2023-05-08 12:51:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.835e-09
2023-05-08 12:51:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.84e-09
2023-05-08 12:51:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.845e-09
2023-05-08 12:51:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.85e-09
2023-05-08 12:51:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.855e-09
2023-05-08 12:51:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.86e-09
2023-05-08 12:51:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.865e-09
2023-05-08 12:51:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.87e-09
2023-05-08 12:51:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.875e-09
2023-05-08 12:51:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.88e-09
2023-05-08 12:51:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.885e-09
2023-05-08 12:51:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.89e-09
2023-05-08 12:51:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.895e-09
2023-05-08 12:51:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.9e-09
2023-05-08 12:51:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.905e-09
2023-05-08 12:51:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.91e-09
2023-05-08 12:51:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.915e-09
2023-05-08 12:51:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.92e-09
2023-05-08 12:51:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.925e-09
2023-05-08 12:51:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.93e-09
2023-05-08 12:51:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.935e-09
2023-05-08 12:51:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.94e-09
2023-05-08 12:51:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.945e-09
2023-05-08 12:51:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.95e-09
2023-05-08 12:51:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.955e-09
2023-05-08 12:51:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.96e-09
2023-05-08 12:51:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.965e-09
2023-05-08 12:51:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.97e-09
2023-05-08 12:51:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.975e-09
2023-05-08 12:51:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.98e-09
2023-05-08 12:51:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.985e-09
2023-05-08 12:51:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.99e-09
2023-05-08 12:51:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=1.995e-09
2023-05-08 12:51:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=2e-09
2023-05-08 12:51:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.005e-09
2023-05-08 12:51:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.01e-09
2023-05-08 12:51:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.015e-09
2023-05-08 12:51:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.02e-09
2023-05-08 12:51:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.025e-09
2023-05-08 12:51:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.03e-09
2023-05-08 12:51:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.035e-09
2023-05-08 12:51:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.04e-09
2023-05-08 12:51:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.045e-09
2023-05-08 12:51:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.05e-09
2023-05-08 12:51:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.055e-09
2023-05-08 12:51:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.06e-09
2023-05-08 12:51:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.065e-09
2023-05-08 12:51:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.07e-09
2023-05-08 12:51:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.075e-09
2023-05-08 12:51:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.08e-09
2023-05-08 12:51:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.085e-09
2023-05-08 12:51:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.09e-09
2023-05-08 12:51:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.095e-09
2023-05-08 12:51:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.1e-09
2023-05-08 12:51:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.105e-09
2023-05-08 12:51:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.11e-09
2023-05-08 12:51:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.115e-09
2023-05-08 12:51:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.12e-09
2023-05-08 12:51:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.125e-09
2023-05-08 12:51:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.13e-09
2023-05-08 12:51:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.135e-09
2023-05-08 12:51:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.14e-09
2023-05-08 12:51:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.145e-09
2023-05-08 12:51:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.15e-09
2023-05-08 12:51:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.155e-09
2023-05-08 12:51:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.16e-09
2023-05-08 12:51:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.165e-09
2023-05-08 12:51:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.17e-09
2023-05-08 12:51:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.175e-09
2023-05-08 12:51:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.18e-09
2023-05-08 12:51:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.185e-09
2023-05-08 12:51:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.19e-09
2023-05-08 12:51:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.195e-09
2023-05-08 12:51:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.2e-09
2023-05-08 12:51:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.205e-09
2023-05-08 12:51:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.21e-09
2023-05-08 12:51:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.215e-09
2023-05-08 12:51:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.22e-09
2023-05-08 12:51:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.225e-09
2023-05-08 12:51:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.23e-09
2023-05-08 12:51:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.235e-09
2023-05-08 12:51:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.24e-09
2023-05-08 12:51:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.245e-09
2023-05-08 12:51:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.25e-09
2023-05-08 12:51:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.255e-09
2023-05-08 12:51:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.26e-09
2023-05-08 12:51:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.265e-09
2023-05-08 12:51:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.27e-09
2023-05-08 12:51:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.275e-09
2023-05-08 12:51:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.28e-09
2023-05-08 12:51:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.285e-09
2023-05-08 12:51:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.29e-09
2023-05-08 12:51:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.295e-09
2023-05-08 12:51:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.3e-09
2023-05-08 12:51:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.305e-09
2023-05-08 12:51:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.31e-09
2023-05-08 12:51:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.315e-09
2023-05-08 12:51:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.32e-09
2023-05-08 12:51:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.325e-09
2023-05-08 12:51:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.33e-09
2023-05-08 12:51:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.335e-09
2023-05-08 12:51:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.34e-09
2023-05-08 12:51:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.345e-09
2023-05-08 12:51:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.35e-09
2023-05-08 12:51:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.355e-09
2023-05-08 12:51:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.36e-09
2023-05-08 12:51:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.365e-09
2023-05-08 12:51:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.37e-09
2023-05-08 12:51:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.375e-09
2023-05-08 12:51:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.38e-09
2023-05-08 12:51:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.385e-09
2023-05-08 12:51:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.39e-09
2023-05-08 12:51:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.395e-09
2023-05-08 12:51:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.4e-09
2023-05-08 12:51:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.405e-09
2023-05-08 12:51:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.41e-09
2023-05-08 12:51:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.415e-09
2023-05-08 12:51:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.42e-09
2023-05-08 12:51:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.425e-09
2023-05-08 12:51:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.43e-09
2023-05-08 12:51:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.435e-09
2023-05-08 12:51:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.44e-09
2023-05-08 12:51:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.445e-09
2023-05-08 12:51:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.45e-09
2023-05-08 12:51:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.455e-09
2023-05-08 12:51:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.46e-09
2023-05-08 12:51:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.465e-09
2023-05-08 12:51:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.47e-09
2023-05-08 12:51:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.475e-09
2023-05-08 12:51:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.48e-09
2023-05-08 12:51:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.485e-09
2023-05-08 12:51:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.49e-09
2023-05-08 12:51:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.495e-09
2023-05-08 12:51:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.5e-09
2023-05-08 12:51:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.505e-09
2023-05-08 12:51:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.51e-09
2023-05-08 12:51:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.515e-09
2023-05-08 12:51:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.52e-09
2023-05-08 12:51:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.525e-09
2023-05-08 12:51:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.53e-09
2023-05-08 12:51:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.535e-09
2023-05-08 12:51:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.54e-09
2023-05-08 12:52:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.545e-09
2023-05-08 12:52:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.55e-09
2023-05-08 12:52:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.555e-09
2023-05-08 12:52:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.56e-09
2023-05-08 12:52:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.565e-09
2023-05-08 12:52:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.57e-09
2023-05-08 12:52:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.575e-09
2023-05-08 12:52:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.58e-09
2023-05-08 12:52:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.585e-09
2023-05-08 12:52:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.59e-09
2023-05-08 12:52:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.595e-09
2023-05-08 12:52:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.6e-09
2023-05-08 12:52:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.605e-09
2023-05-08 12:52:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.61e-09
2023-05-08 12:52:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.615e-09
2023-05-08 12:52:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.62e-09
2023-05-08 12:52:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.625e-09
2023-05-08 12:52:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.63e-09
2023-05-08 12:52:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.635e-09
2023-05-08 12:52:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.64e-09
2023-05-08 12:52:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.645e-09
2023-05-08 12:52:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.65e-09
2023-05-08 12:52:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.655e-09
2023-05-08 12:52:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.66e-09
2023-05-08 12:52:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.665e-09
2023-05-08 12:52:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.67e-09
2023-05-08 12:52:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.675e-09
2023-05-08 12:52:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.68e-09
2023-05-08 12:52:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.685e-09
2023-05-08 12:52:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.69e-09
2023-05-08 12:52:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.695e-09
2023-05-08 12:52:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.7e-09
2023-05-08 12:52:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.705e-09
2023-05-08 12:52:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.71e-09
2023-05-08 12:52:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.715e-09
2023-05-08 12:52:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.72e-09
2023-05-08 12:52:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.725e-09
2023-05-08 12:52:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.73e-09
2023-05-08 12:52:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.735e-09
2023-05-08 12:52:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.74e-09
2023-05-08 12:52:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.745e-09
2023-05-08 12:52:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.75e-09
2023-05-08 12:52:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.755e-09
2023-05-08 12:52:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.76e-09
2023-05-08 12:52:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.765e-09
2023-05-08 12:52:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.77e-09
2023-05-08 12:52:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.775e-09
2023-05-08 12:52:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.78e-09
2023-05-08 12:52:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.785e-09
2023-05-08 12:52:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.79e-09
2023-05-08 12:52:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.795e-09
2023-05-08 12:52:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.8e-09
2023-05-08 12:52:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.805e-09
2023-05-08 12:52:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.81e-09
2023-05-08 12:52:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.815e-09
2023-05-08 12:52:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.82e-09
2023-05-08 12:52:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.825e-09
2023-05-08 12:52:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.83e-09
2023-05-08 12:52:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.835e-09
2023-05-08 12:52:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.84e-09
2023-05-08 12:52:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.845e-09
2023-05-08 12:52:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.85e-09
2023-05-08 12:52:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.855e-09
2023-05-08 12:52:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.86e-09
2023-05-08 12:52:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.865e-09
2023-05-08 12:52:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.87e-09
2023-05-08 12:52:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.875e-09
2023-05-08 12:52:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.88e-09
2023-05-08 12:52:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.885e-09
2023-05-08 12:52:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.89e-09
2023-05-08 12:52:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.895e-09
2023-05-08 12:52:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.9e-09
2023-05-08 12:52:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.905e-09
2023-05-08 12:52:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.91e-09
2023-05-08 12:52:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.915e-09
2023-05-08 12:52:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.92e-09
2023-05-08 12:52:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.925e-09
2023-05-08 12:52:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.93e-09
2023-05-08 12:52:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.935e-09
2023-05-08 12:52:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.94e-09
2023-05-08 12:52:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.945e-09
2023-05-08 12:52:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.95e-09
2023-05-08 12:52:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.955e-09
2023-05-08 12:52:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.96e-09
2023-05-08 12:52:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.965e-09
2023-05-08 12:52:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.97e-09
2023-05-08 12:52:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.975e-09
2023-05-08 12:52:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.98e-09
2023-05-08 12:52:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.985e-09
2023-05-08 12:52:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.99e-09
2023-05-08 12:52:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=2.995e-09
2023-05-08 12:52:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=3e-09
2023-05-08 12:52:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.005e-09
2023-05-08 12:52:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.01e-09
2023-05-08 12:52:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.015e-09
2023-05-08 12:52:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.02e-09
2023-05-08 12:52:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.025e-09
2023-05-08 12:52:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.03e-09
2023-05-08 12:52:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.035e-09
2023-05-08 12:52:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.04e-09
2023-05-08 12:52:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.045e-09
2023-05-08 12:52:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.05e-09
2023-05-08 12:52:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.055e-09
2023-05-08 12:52:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.06e-09
2023-05-08 12:52:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.065e-09
2023-05-08 12:52:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.07e-09
2023-05-08 12:52:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.075e-09
2023-05-08 12:52:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.08e-09
2023-05-08 12:52:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.085e-09
2023-05-08 12:52:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.09e-09
2023-05-08 12:52:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.095e-09
2023-05-08 12:52:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.1e-09
2023-05-08 12:52:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.105e-09
2023-05-08 12:52:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.11e-09
2023-05-08 12:52:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.115e-09
2023-05-08 12:52:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.12e-09
2023-05-08 12:52:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.125e-09
2023-05-08 12:52:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.13e-09
2023-05-08 12:52:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.135e-09
2023-05-08 12:52:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.14e-09
2023-05-08 12:52:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.145e-09
2023-05-08 12:52:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.15e-09
2023-05-08 12:52:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.155e-09
2023-05-08 12:52:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.16e-09
2023-05-08 12:52:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.165e-09
2023-05-08 12:52:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.17e-09
2023-05-08 12:52:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.175e-09
2023-05-08 12:52:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.18e-09
2023-05-08 12:52:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.185e-09
2023-05-08 12:52:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.19e-09
2023-05-08 12:52:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.195e-09
2023-05-08 12:52:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.2e-09
2023-05-08 12:52:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.205e-09
2023-05-08 12:52:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.21e-09
2023-05-08 12:52:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.215e-09
2023-05-08 12:52:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.22e-09
2023-05-08 12:52:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.225e-09
2023-05-08 12:52:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.23e-09
2023-05-08 12:52:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.235e-09
2023-05-08 12:52:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.24e-09
2023-05-08 12:52:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.245e-09
2023-05-08 12:52:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.25e-09
2023-05-08 12:52:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.255e-09
2023-05-08 12:52:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.26e-09
2023-05-08 12:52:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.265e-09
2023-05-08 12:52:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.27e-09
2023-05-08 12:52:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.275e-09
2023-05-08 12:52:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.28e-09
2023-05-08 12:52:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.285e-09
2023-05-08 12:52:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.29e-09
2023-05-08 12:52:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.295e-09
2023-05-08 12:52:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.3e-09
2023-05-08 12:52:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.305e-09
2023-05-08 12:52:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.31e-09
2023-05-08 12:52:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.315e-09
2023-05-08 12:52:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.32e-09
2023-05-08 12:52:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.325e-09
2023-05-08 12:52:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.33e-09
2023-05-08 12:52:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.335e-09
2023-05-08 12:52:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.34e-09
2023-05-08 12:52:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.345e-09
2023-05-08 12:52:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.35e-09
2023-05-08 12:52:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.355e-09
2023-05-08 12:52:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.36e-09
2023-05-08 12:52:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.365e-09
2023-05-08 12:52:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.37e-09
2023-05-08 12:52:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.375e-09
2023-05-08 12:52:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.38e-09
2023-05-08 12:52:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.385e-09
2023-05-08 12:52:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.39e-09
2023-05-08 12:52:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.395e-09
2023-05-08 12:52:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.4e-09
2023-05-08 12:52:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.405e-09
2023-05-08 12:52:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.41e-09
2023-05-08 12:52:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.415e-09
2023-05-08 12:52:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.42e-09
2023-05-08 12:52:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.425e-09
2023-05-08 12:52:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.43e-09
2023-05-08 12:52:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.435e-09
2023-05-08 12:52:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.44e-09
2023-05-08 12:52:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.445e-09
2023-05-08 12:52:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.45e-09
2023-05-08 12:52:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.455e-09
2023-05-08 12:53:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.46e-09
2023-05-08 12:53:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.465e-09
2023-05-08 12:53:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.47e-09
2023-05-08 12:53:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.475e-09
2023-05-08 12:53:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.48e-09
2023-05-08 12:53:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.485e-09
2023-05-08 12:53:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.49e-09
2023-05-08 12:53:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.495e-09
2023-05-08 12:53:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.5e-09
2023-05-08 12:53:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.505e-09
2023-05-08 12:53:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.51e-09
2023-05-08 12:53:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.515e-09
2023-05-08 12:53:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.52e-09
2023-05-08 12:53:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.525e-09
2023-05-08 12:53:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.53e-09
2023-05-08 12:53:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.535e-09
2023-05-08 12:53:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.54e-09
2023-05-08 12:53:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.545e-09
2023-05-08 12:53:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.55e-09
2023-05-08 12:53:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.555e-09
2023-05-08 12:53:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.56e-09
2023-05-08 12:53:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.565e-09
2023-05-08 12:53:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.57e-09
2023-05-08 12:53:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.575e-09
2023-05-08 12:53:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.58e-09
2023-05-08 12:53:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.585e-09
2023-05-08 12:53:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.59e-09
2023-05-08 12:53:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.595e-09
2023-05-08 12:53:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.6e-09
2023-05-08 12:53:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.605e-09
2023-05-08 12:53:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.61e-09
2023-05-08 12:53:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.615e-09
2023-05-08 12:53:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.62e-09
2023-05-08 12:53:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.625e-09
2023-05-08 12:53:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.63e-09
2023-05-08 12:53:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.635e-09
2023-05-08 12:53:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.64e-09
2023-05-08 12:53:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.645e-09
2023-05-08 12:53:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.65e-09
2023-05-08 12:53:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.655e-09
2023-05-08 12:53:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.66e-09
2023-05-08 12:53:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.665e-09
2023-05-08 12:53:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.67e-09
2023-05-08 12:53:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.675e-09
2023-05-08 12:53:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.68e-09
2023-05-08 12:53:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.685e-09
2023-05-08 12:53:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.69e-09
2023-05-08 12:53:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.695e-09
2023-05-08 12:53:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.7e-09
2023-05-08 12:53:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.705e-09
2023-05-08 12:53:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.71e-09
2023-05-08 12:53:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.715e-09
2023-05-08 12:53:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.72e-09
2023-05-08 12:53:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.725e-09
2023-05-08 12:53:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.73e-09
2023-05-08 12:53:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.735e-09
2023-05-08 12:53:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.74e-09
2023-05-08 12:53:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.745e-09
2023-05-08 12:53:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.75e-09
2023-05-08 12:53:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.755e-09
2023-05-08 12:53:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.76e-09
2023-05-08 12:53:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.765e-09
2023-05-08 12:53:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.77e-09
2023-05-08 12:53:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.775e-09
2023-05-08 12:53:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.78e-09
2023-05-08 12:53:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.785e-09
2023-05-08 12:53:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.79e-09
2023-05-08 12:53:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.795e-09
2023-05-08 12:53:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.8e-09
2023-05-08 12:53:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.805e-09
2023-05-08 12:53:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.81e-09
2023-05-08 12:53:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.815e-09
2023-05-08 12:53:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.82e-09
2023-05-08 12:53:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.825e-09
2023-05-08 12:53:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.83e-09
2023-05-08 12:53:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.835e-09
2023-05-08 12:53:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.84e-09
2023-05-08 12:53:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.845e-09
2023-05-08 12:53:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.85e-09
2023-05-08 12:53:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.855e-09
2023-05-08 12:53:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.86e-09
2023-05-08 12:53:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.865e-09
2023-05-08 12:53:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.87e-09
2023-05-08 12:53:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.875e-09
2023-05-08 12:53:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.88e-09
2023-05-08 12:53:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.885e-09
2023-05-08 12:53:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.89e-09
2023-05-08 12:53:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.895e-09
2023-05-08 12:53:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.9e-09
2023-05-08 12:53:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.905e-09
2023-05-08 12:53:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.91e-09
2023-05-08 12:53:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.915e-09
2023-05-08 12:53:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.92e-09
2023-05-08 12:53:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.925e-09
2023-05-08 12:53:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.93e-09
2023-05-08 12:53:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.935e-09
2023-05-08 12:53:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.94e-09
2023-05-08 12:53:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.945e-09
2023-05-08 12:53:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.95e-09
2023-05-08 12:53:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.955e-09
2023-05-08 12:53:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.96e-09
2023-05-08 12:53:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.965e-09
2023-05-08 12:53:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.97e-09
2023-05-08 12:53:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.975e-09
2023-05-08 12:53:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.98e-09
2023-05-08 12:53:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.985e-09
2023-05-08 12:53:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.99e-09
2023-05-08 12:53:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=3.995e-09
2023-05-08 12:53:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=4e-09
2023-05-08 12:53:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.005e-09
2023-05-08 12:53:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.01e-09
2023-05-08 12:53:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.015e-09
2023-05-08 12:53:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.02e-09
2023-05-08 12:53:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.025e-09
2023-05-08 12:53:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.03e-09
2023-05-08 12:53:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.035e-09
2023-05-08 12:53:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.04e-09
2023-05-08 12:53:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.045e-09
2023-05-08 12:53:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.05e-09
2023-05-08 12:53:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.055e-09
2023-05-08 12:53:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.06e-09
2023-05-08 12:53:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.065e-09
2023-05-08 12:53:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.07e-09
2023-05-08 12:53:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.075e-09
2023-05-08 12:53:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.08e-09
2023-05-08 12:53:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.085e-09
2023-05-08 12:53:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.09e-09
2023-05-08 12:53:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.095e-09
2023-05-08 12:53:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.1e-09
2023-05-08 12:53:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.105e-09
2023-05-08 12:53:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.11e-09
2023-05-08 12:53:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.115e-09
2023-05-08 12:53:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.12e-09
2023-05-08 12:53:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.125e-09
2023-05-08 12:53:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.13e-09
2023-05-08 12:53:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.135e-09
2023-05-08 12:53:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.14e-09
2023-05-08 12:53:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.145e-09
2023-05-08 12:53:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.15e-09
2023-05-08 12:53:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.155e-09
2023-05-08 12:53:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.16e-09
2023-05-08 12:53:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.165e-09
2023-05-08 12:53:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.17e-09
2023-05-08 12:53:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.175e-09
2023-05-08 12:53:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.18e-09
2023-05-08 12:53:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.185e-09
2023-05-08 12:53:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.19e-09
2023-05-08 12:53:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.195e-09
2023-05-08 12:53:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.2e-09
2023-05-08 12:53:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.205e-09
2023-05-08 12:53:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.21e-09
2023-05-08 12:53:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.215e-09
2023-05-08 12:53:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.22e-09
2023-05-08 12:53:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.225e-09
2023-05-08 12:53:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.23e-09
2023-05-08 12:53:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.235e-09
2023-05-08 12:53:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.24e-09
2023-05-08 12:53:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.245e-09
2023-05-08 12:53:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.25e-09
2023-05-08 12:53:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.255e-09
2023-05-08 12:53:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.26e-09
2023-05-08 12:53:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.265e-09
2023-05-08 12:53:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.27e-09
2023-05-08 12:53:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.275e-09
2023-05-08 12:53:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.28e-09
2023-05-08 12:53:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.285e-09
2023-05-08 12:53:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.29e-09
2023-05-08 12:53:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.295e-09
2023-05-08 12:53:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.3e-09
2023-05-08 12:53:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.305e-09
2023-05-08 12:53:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.31e-09
2023-05-08 12:53:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.315e-09
2023-05-08 12:53:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.32e-09
2023-05-08 12:53:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.325e-09
2023-05-08 12:53:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.33e-09
2023-05-08 12:53:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.335e-09
2023-05-08 12:53:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.34e-09
2023-05-08 12:53:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.345e-09
2023-05-08 12:53:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.35e-09
2023-05-08 12:53:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.355e-09
2023-05-08 12:54:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.36e-09
2023-05-08 12:54:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.365e-09
2023-05-08 12:54:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.37e-09
2023-05-08 12:54:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.375e-09
2023-05-08 12:54:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.38e-09
2023-05-08 12:54:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.385e-09
2023-05-08 12:54:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.39e-09
2023-05-08 12:54:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.395e-09
2023-05-08 12:54:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.4e-09
2023-05-08 12:54:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.405e-09
2023-05-08 12:54:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.41e-09
2023-05-08 12:54:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.415e-09
2023-05-08 12:54:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.42e-09
2023-05-08 12:54:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.425e-09
2023-05-08 12:54:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.43e-09
2023-05-08 12:54:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.435e-09
2023-05-08 12:54:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.44e-09
2023-05-08 12:54:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.445e-09
2023-05-08 12:54:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.45e-09
2023-05-08 12:54:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.455e-09
2023-05-08 12:54:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.46e-09
2023-05-08 12:54:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.465e-09
2023-05-08 12:54:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.47e-09
2023-05-08 12:54:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.475e-09
2023-05-08 12:54:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.48e-09
2023-05-08 12:54:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.485e-09
2023-05-08 12:54:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.49e-09
2023-05-08 12:54:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.495e-09
2023-05-08 12:54:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.5e-09
2023-05-08 12:54:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.505e-09
2023-05-08 12:54:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.51e-09
2023-05-08 12:54:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.515e-09
2023-05-08 12:54:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.52e-09
2023-05-08 12:54:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.525e-09
2023-05-08 12:54:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.53e-09
2023-05-08 12:54:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.535e-09
2023-05-08 12:54:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.54e-09
2023-05-08 12:54:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.545e-09
2023-05-08 12:54:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.55e-09
2023-05-08 12:54:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.555e-09
2023-05-08 12:54:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.56e-09
2023-05-08 12:54:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.565e-09
2023-05-08 12:54:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.57e-09
2023-05-08 12:54:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.575e-09
2023-05-08 12:54:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.58e-09
2023-05-08 12:54:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.585e-09
2023-05-08 12:54:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.59e-09
2023-05-08 12:54:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.595e-09
2023-05-08 12:54:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.6e-09
2023-05-08 12:54:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.605e-09
2023-05-08 12:54:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.61e-09
2023-05-08 12:54:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.615e-09
2023-05-08 12:54:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.62e-09
2023-05-08 12:54:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.625e-09
2023-05-08 12:54:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.63e-09
2023-05-08 12:54:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.635e-09
2023-05-08 12:54:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.64e-09
2023-05-08 12:54:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.645e-09
2023-05-08 12:54:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.65e-09
2023-05-08 12:54:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.655e-09
2023-05-08 12:54:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.66e-09
2023-05-08 12:54:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.665e-09
2023-05-08 12:54:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.67e-09
2023-05-08 12:54:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.675e-09
2023-05-08 12:54:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.68e-09
2023-05-08 12:54:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.685e-09
2023-05-08 12:54:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.69e-09
2023-05-08 12:54:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.695e-09
2023-05-08 12:54:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.7e-09
2023-05-08 12:54:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.705e-09
2023-05-08 12:54:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.71e-09
2023-05-08 12:54:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.715e-09
2023-05-08 12:54:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.72e-09
2023-05-08 12:54:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.725e-09
2023-05-08 12:54:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.73e-09
2023-05-08 12:54:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.735e-09
2023-05-08 12:54:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.74e-09
2023-05-08 12:54:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.745e-09
2023-05-08 12:54:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.75e-09
2023-05-08 12:54:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.755e-09
2023-05-08 12:54:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.76e-09
2023-05-08 12:54:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.765e-09
2023-05-08 12:54:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.77e-09
2023-05-08 12:54:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.775e-09
2023-05-08 12:54:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.78e-09
2023-05-08 12:54:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.785e-09
2023-05-08 12:54:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.79e-09
2023-05-08 12:54:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.795e-09
2023-05-08 12:54:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.8e-09
2023-05-08 12:54:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.805e-09
2023-05-08 12:54:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.81e-09
2023-05-08 12:54:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.815e-09
2023-05-08 12:54:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.82e-09
2023-05-08 12:54:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.825e-09
2023-05-08 12:54:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.83e-09
2023-05-08 12:54:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.835e-09
2023-05-08 12:54:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.84e-09
2023-05-08 12:54:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.845e-09
2023-05-08 12:54:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.85e-09
2023-05-08 12:54:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.855e-09
2023-05-08 12:54:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.86e-09
2023-05-08 12:54:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.865e-09
2023-05-08 12:54:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.87e-09
2023-05-08 12:54:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.875e-09
2023-05-08 12:54:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.88e-09
2023-05-08 12:54:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.885e-09
2023-05-08 12:54:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.89e-09
2023-05-08 12:54:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.895e-09
2023-05-08 12:54:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.9e-09
2023-05-08 12:54:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.905e-09
2023-05-08 12:54:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.91e-09
2023-05-08 12:54:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.915e-09
2023-05-08 12:54:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.92e-09
2023-05-08 12:54:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.925e-09
2023-05-08 12:54:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.93e-09
2023-05-08 12:54:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.935e-09
2023-05-08 12:54:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.94e-09
2023-05-08 12:54:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.945e-09
2023-05-08 12:54:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.95e-09
2023-05-08 12:54:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.955e-09
2023-05-08 12:54:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.96e-09
2023-05-08 12:54:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.965e-09
2023-05-08 12:54:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.97e-09
2023-05-08 12:54:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.975e-09
2023-05-08 12:54:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.98e-09
2023-05-08 12:54:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.985e-09
2023-05-08 12:54:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.99e-09
2023-05-08 12:54:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=4.995e-09
2023-05-08 12:54:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=5e-09
2023-05-08 12:54:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.005e-09
2023-05-08 12:54:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.01e-09
2023-05-08 12:54:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.015e-09
2023-05-08 12:54:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.02e-09
2023-05-08 12:54:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.025e-09
2023-05-08 12:54:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.03e-09
2023-05-08 12:54:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.035e-09
2023-05-08 12:54:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.04e-09
2023-05-08 12:54:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.045e-09
2023-05-08 12:54:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.05e-09
2023-05-08 12:54:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.055e-09
2023-05-08 12:54:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.06e-09
2023-05-08 12:54:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.065e-09
2023-05-08 12:54:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.07e-09
2023-05-08 12:54:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.075e-09
2023-05-08 12:54:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.08e-09
2023-05-08 12:54:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.085e-09
2023-05-08 12:54:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.09e-09
2023-05-08 12:54:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.095e-09
2023-05-08 12:54:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.1e-09
2023-05-08 12:54:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.105e-09
2023-05-08 12:54:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.11e-09
2023-05-08 12:54:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.115e-09
2023-05-08 12:54:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.12e-09
2023-05-08 12:54:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.125e-09
2023-05-08 12:54:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.13e-09
2023-05-08 12:54:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.135e-09
2023-05-08 12:54:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.14e-09
2023-05-08 12:54:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.145e-09
2023-05-08 12:54:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.15e-09
2023-05-08 12:54:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.155e-09
2023-05-08 12:54:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.16e-09
2023-05-08 12:54:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.165e-09
2023-05-08 12:54:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.17e-09
2023-05-08 12:54:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.175e-09
2023-05-08 12:55:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.18e-09
2023-05-08 12:55:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.185e-09
2023-05-08 12:55:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.19e-09
2023-05-08 12:55:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.195e-09
2023-05-08 12:55:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.2e-09
2023-05-08 12:55:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.205e-09
2023-05-08 12:55:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.21e-09
2023-05-08 12:55:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.215e-09
2023-05-08 12:55:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.22e-09
2023-05-08 12:55:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.225e-09
2023-05-08 12:55:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.23e-09
2023-05-08 12:55:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.235e-09
2023-05-08 12:55:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.24e-09
2023-05-08 12:55:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.245e-09
2023-05-08 12:55:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.25e-09
2023-05-08 12:55:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.255e-09
2023-05-08 12:55:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.26e-09
2023-05-08 12:55:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.265e-09
2023-05-08 12:55:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.27e-09
2023-05-08 12:55:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.275e-09
2023-05-08 12:55:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.28e-09
2023-05-08 12:55:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.285e-09
2023-05-08 12:55:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.29e-09
2023-05-08 12:55:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.295e-09
2023-05-08 12:55:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.3e-09
2023-05-08 12:55:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.305e-09
2023-05-08 12:55:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.31e-09
2023-05-08 12:55:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.315e-09
2023-05-08 12:55:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.32e-09
2023-05-08 12:55:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.325e-09
2023-05-08 12:55:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.33e-09
2023-05-08 12:55:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.335e-09
2023-05-08 12:55:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.34e-09
2023-05-08 12:55:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.345e-09
2023-05-08 12:55:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.35e-09
2023-05-08 12:55:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.355e-09
2023-05-08 12:55:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.36e-09
2023-05-08 12:55:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.365e-09
2023-05-08 12:55:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.37e-09
2023-05-08 12:55:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.375e-09
2023-05-08 12:55:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.38e-09
2023-05-08 12:55:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.385e-09
2023-05-08 12:55:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.39e-09
2023-05-08 12:55:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.395e-09
2023-05-08 12:55:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.4e-09
2023-05-08 12:55:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.405e-09
2023-05-08 12:55:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.41e-09
2023-05-08 12:55:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.415e-09
2023-05-08 12:55:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.42e-09
2023-05-08 12:55:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.425e-09
2023-05-08 12:55:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.43e-09
2023-05-08 12:55:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.435e-09
2023-05-08 12:55:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.44e-09
2023-05-08 12:55:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.445e-09
2023-05-08 12:55:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.45e-09
2023-05-08 12:55:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.455e-09
2023-05-08 12:55:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.46e-09
2023-05-08 12:55:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.465e-09
2023-05-08 12:55:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.47e-09
2023-05-08 12:55:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.475e-09
2023-05-08 12:55:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.48e-09
2023-05-08 12:55:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.485e-09
2023-05-08 12:55:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.49e-09
2023-05-08 12:55:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.495e-09
2023-05-08 12:55:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.5e-09
2023-05-08 12:55:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.505e-09
2023-05-08 12:55:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.51e-09
2023-05-08 12:55:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.515e-09
2023-05-08 12:55:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.52e-09
2023-05-08 12:55:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.525e-09
2023-05-08 12:55:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.53e-09
2023-05-08 12:55:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.535e-09
2023-05-08 12:55:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.54e-09
2023-05-08 12:55:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.545e-09
2023-05-08 12:55:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.55e-09
2023-05-08 12:55:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.555e-09
2023-05-08 12:55:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.56e-09
2023-05-08 12:55:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.565e-09
2023-05-08 12:55:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.57e-09
2023-05-08 12:55:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.575e-09
2023-05-08 12:55:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.58e-09
2023-05-08 12:55:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.585e-09
2023-05-08 12:55:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.59e-09
2023-05-08 12:55:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.595e-09
2023-05-08 12:55:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.6e-09
2023-05-08 12:55:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.605e-09
2023-05-08 12:55:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.61e-09
2023-05-08 12:55:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.615e-09
2023-05-08 12:55:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.62e-09
2023-05-08 12:55:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.625e-09
2023-05-08 12:55:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.63e-09
2023-05-08 12:55:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.635e-09
2023-05-08 12:55:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.64e-09
2023-05-08 12:55:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.645e-09
2023-05-08 12:55:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.65e-09
2023-05-08 12:55:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.655e-09
2023-05-08 12:55:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.66e-09
2023-05-08 12:55:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.665e-09
2023-05-08 12:55:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.67e-09
2023-05-08 12:55:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.675e-09
2023-05-08 12:55:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.68e-09
2023-05-08 12:55:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.685e-09
2023-05-08 12:55:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.69e-09
2023-05-08 12:55:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.695e-09
2023-05-08 12:55:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.7e-09
2023-05-08 12:55:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.705e-09
2023-05-08 12:55:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.71e-09
2023-05-08 12:55:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.715e-09
2023-05-08 12:55:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.72e-09
2023-05-08 12:55:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.725e-09
2023-05-08 12:55:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.73e-09
2023-05-08 12:55:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.735e-09
2023-05-08 12:55:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.74e-09
2023-05-08 12:55:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.745e-09
2023-05-08 12:55:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.75e-09
2023-05-08 12:55:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.755e-09
2023-05-08 12:55:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.76e-09
2023-05-08 12:55:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.765e-09
2023-05-08 12:55:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.77e-09
2023-05-08 12:55:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.775e-09
2023-05-08 12:55:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.78e-09
2023-05-08 12:55:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.785e-09
2023-05-08 12:55:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.79e-09
2023-05-08 12:55:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.795e-09
2023-05-08 12:55:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.8e-09
2023-05-08 12:55:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.805e-09
2023-05-08 12:55:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.81e-09
2023-05-08 12:55:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.815e-09
2023-05-08 12:55:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.82e-09
2023-05-08 12:55:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.825e-09
2023-05-08 12:55:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.83e-09
2023-05-08 12:55:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.835e-09
2023-05-08 12:55:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.84e-09
2023-05-08 12:55:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.845e-09
2023-05-08 12:55:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.85e-09
2023-05-08 12:55:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.855e-09
2023-05-08 12:55:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.86e-09
2023-05-08 12:55:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.865e-09
2023-05-08 12:55:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.87e-09
2023-05-08 12:55:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.875e-09
2023-05-08 12:55:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.88e-09
2023-05-08 12:55:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.885e-09
2023-05-08 12:55:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.89e-09
2023-05-08 12:55:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.895e-09
2023-05-08 12:55:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.9e-09
2023-05-08 12:55:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.905e-09
2023-05-08 12:55:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.91e-09
2023-05-08 12:55:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.915e-09
2023-05-08 12:55:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.92e-09
2023-05-08 12:55:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.925e-09
2023-05-08 12:56:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.93e-09
2023-05-08 12:56:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.935e-09
2023-05-08 12:56:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.94e-09
2023-05-08 12:56:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.945e-09
2023-05-08 12:56:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.95e-09
2023-05-08 12:56:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.955e-09
2023-05-08 12:56:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.96e-09
2023-05-08 12:56:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.965e-09
2023-05-08 12:56:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.97e-09
2023-05-08 12:56:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.975e-09
2023-05-08 12:56:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.98e-09
2023-05-08 12:56:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.985e-09
2023-05-08 12:56:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.99e-09
2023-05-08 12:56:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=5.995e-09
2023-05-08 12:56:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=6e-09
2023-05-08 12:56:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.005e-09
2023-05-08 12:56:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.01e-09
2023-05-08 12:56:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.015e-09
2023-05-08 12:56:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.02e-09
2023-05-08 12:56:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.025e-09
2023-05-08 12:56:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.03e-09
2023-05-08 12:56:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.035e-09
2023-05-08 12:56:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.04e-09
2023-05-08 12:56:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.045e-09
2023-05-08 12:56:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.05e-09
2023-05-08 12:56:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.055e-09
2023-05-08 12:56:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.06e-09
2023-05-08 12:56:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.065e-09
2023-05-08 12:56:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.07e-09
2023-05-08 12:56:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.075e-09
2023-05-08 12:56:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.08e-09
2023-05-08 12:56:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.085e-09
2023-05-08 12:56:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.09e-09
2023-05-08 12:56:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.095e-09
2023-05-08 12:56:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.1e-09
2023-05-08 12:56:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.105e-09
2023-05-08 12:56:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.11e-09
2023-05-08 12:56:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.115e-09
2023-05-08 12:56:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.12e-09
2023-05-08 12:56:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.125e-09
2023-05-08 12:56:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.13e-09
2023-05-08 12:56:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.135e-09
2023-05-08 12:56:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.14e-09
2023-05-08 12:56:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.145e-09
2023-05-08 12:56:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.15e-09
2023-05-08 12:56:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.155e-09
2023-05-08 12:56:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.16e-09
2023-05-08 12:56:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.165e-09
2023-05-08 12:56:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.17e-09
2023-05-08 12:56:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.175e-09
2023-05-08 12:56:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.18e-09
2023-05-08 12:56:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.185e-09
2023-05-08 12:56:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.19e-09
2023-05-08 12:56:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.195e-09
2023-05-08 12:56:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.2e-09
2023-05-08 12:56:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.205e-09
2023-05-08 12:56:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.21e-09
2023-05-08 12:56:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.215e-09
2023-05-08 12:56:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.22e-09
2023-05-08 12:56:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.225e-09
2023-05-08 12:56:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.23e-09
2023-05-08 12:56:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.235e-09
2023-05-08 12:56:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.24e-09
2023-05-08 12:56:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.245e-09
2023-05-08 12:56:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.25e-09
2023-05-08 12:56:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.255e-09
2023-05-08 12:56:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.26e-09
2023-05-08 12:56:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.265e-09
2023-05-08 12:56:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.27e-09
2023-05-08 12:56:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.275e-09
2023-05-08 12:56:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.28e-09
2023-05-08 12:56:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.285e-09
2023-05-08 12:56:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.29e-09
2023-05-08 12:56:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.295e-09
2023-05-08 12:56:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.3e-09
2023-05-08 12:56:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.305e-09
2023-05-08 12:56:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.31e-09
2023-05-08 12:56:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.315e-09
2023-05-08 12:56:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.32e-09
2023-05-08 12:56:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.325e-09
2023-05-08 12:56:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.33e-09
2023-05-08 12:56:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.335e-09
2023-05-08 12:56:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.34e-09
2023-05-08 12:56:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.345e-09
2023-05-08 12:56:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.35e-09
2023-05-08 12:56:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.355e-09
2023-05-08 12:56:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.36e-09
2023-05-08 12:56:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.365e-09
2023-05-08 12:56:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.37e-09
2023-05-08 12:56:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.375e-09
2023-05-08 12:56:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.38e-09
2023-05-08 12:56:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.385e-09
2023-05-08 12:56:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.39e-09
2023-05-08 12:56:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.395e-09
2023-05-08 12:56:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.4e-09
2023-05-08 12:56:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.405e-09
2023-05-08 12:56:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.41e-09
2023-05-08 12:56:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.415e-09
2023-05-08 12:56:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.42e-09
2023-05-08 12:56:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.425e-09
2023-05-08 12:56:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.43e-09
2023-05-08 12:56:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.435e-09
2023-05-08 12:56:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.44e-09
2023-05-08 12:56:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.445e-09
2023-05-08 12:56:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.45e-09
2023-05-08 12:56:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.455e-09
2023-05-08 12:56:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.46e-09
2023-05-08 12:56:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.465e-09
2023-05-08 12:56:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.47e-09
2023-05-08 12:56:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.475e-09
2023-05-08 12:56:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.48e-09
2023-05-08 12:56:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.485e-09
2023-05-08 12:56:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.49e-09
2023-05-08 12:56:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.495e-09
2023-05-08 12:56:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.5e-09
2023-05-08 12:56:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.505e-09
2023-05-08 12:56:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.51e-09
2023-05-08 12:56:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.515e-09
2023-05-08 12:56:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.52e-09
2023-05-08 12:56:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.525e-09
2023-05-08 12:56:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.53e-09
2023-05-08 12:56:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.535e-09
2023-05-08 12:56:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.54e-09
2023-05-08 12:56:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.545e-09
2023-05-08 12:56:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.55e-09
2023-05-08 12:56:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.555e-09
2023-05-08 12:56:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.56e-09
2023-05-08 12:56:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.565e-09
2023-05-08 12:56:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.57e-09
2023-05-08 12:56:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.575e-09
2023-05-08 12:56:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.58e-09
2023-05-08 12:56:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.585e-09
2023-05-08 12:56:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.59e-09
2023-05-08 12:56:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.595e-09
2023-05-08 12:56:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.6e-09
2023-05-08 12:56:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.605e-09
2023-05-08 12:56:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.61e-09
2023-05-08 12:56:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.615e-09
2023-05-08 12:56:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.62e-09
2023-05-08 12:56:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.625e-09
2023-05-08 12:56:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.63e-09
2023-05-08 12:56:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.635e-09
2023-05-08 12:56:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.64e-09
2023-05-08 12:56:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.645e-09
2023-05-08 12:56:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.65e-09
2023-05-08 12:56:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.655e-09
2023-05-08 12:56:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.66e-09
2023-05-08 12:56:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.665e-09
2023-05-08 12:56:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.67e-09
2023-05-08 12:56:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.675e-09
2023-05-08 12:56:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.68e-09
2023-05-08 12:56:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.685e-09
2023-05-08 12:56:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.69e-09
2023-05-08 12:56:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.695e-09
2023-05-08 12:56:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.7e-09
2023-05-08 12:56:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.705e-09
2023-05-08 12:56:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.71e-09
2023-05-08 12:56:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.715e-09
2023-05-08 12:56:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.72e-09
2023-05-08 12:56:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.725e-09
2023-05-08 12:56:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.73e-09
2023-05-08 12:56:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.735e-09
2023-05-08 12:56:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.74e-09
2023-05-08 12:56:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.745e-09
2023-05-08 12:56:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.75e-09
2023-05-08 12:57:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.755e-09
2023-05-08 12:57:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.76e-09
2023-05-08 12:57:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.765e-09
2023-05-08 12:57:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.77e-09
2023-05-08 12:57:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.775e-09
2023-05-08 12:57:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.78e-09
2023-05-08 12:57:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.785e-09
2023-05-08 12:57:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.79e-09
2023-05-08 12:57:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.795e-09
2023-05-08 12:57:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.8e-09
2023-05-08 12:57:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.805e-09
2023-05-08 12:57:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.81e-09
2023-05-08 12:57:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.815e-09
2023-05-08 12:57:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.82e-09
2023-05-08 12:57:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.825e-09
2023-05-08 12:57:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.83e-09
2023-05-08 12:57:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.835e-09
2023-05-08 12:57:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.84e-09
2023-05-08 12:57:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.845e-09
2023-05-08 12:57:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.85e-09
2023-05-08 12:57:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.855e-09
2023-05-08 12:57:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.86e-09
2023-05-08 12:57:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.865e-09
2023-05-08 12:57:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.87e-09
2023-05-08 12:57:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.875e-09
2023-05-08 12:57:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.88e-09
2023-05-08 12:57:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.885e-09
2023-05-08 12:57:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.89e-09
2023-05-08 12:57:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.895e-09
2023-05-08 12:57:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.9e-09
2023-05-08 12:57:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.905e-09
2023-05-08 12:57:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.91e-09
2023-05-08 12:57:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.915e-09
2023-05-08 12:57:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.92e-09
2023-05-08 12:57:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.925e-09
2023-05-08 12:57:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.93e-09
2023-05-08 12:57:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.935e-09
2023-05-08 12:57:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.94e-09
2023-05-08 12:57:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.945e-09
2023-05-08 12:57:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.95e-09
2023-05-08 12:57:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.955e-09
2023-05-08 12:57:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.96e-09
2023-05-08 12:57:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.965e-09
2023-05-08 12:57:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.97e-09
2023-05-08 12:57:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.975e-09
2023-05-08 12:57:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.98e-09
2023-05-08 12:57:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.985e-09
2023-05-08 12:57:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.99e-09
2023-05-08 12:57:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=6.995e-09
2023-05-08 12:57:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=7e-09
2023-05-08 12:57:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.005e-09
2023-05-08 12:57:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.01e-09
2023-05-08 12:57:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.015e-09
2023-05-08 12:57:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.02e-09
2023-05-08 12:57:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.025e-09
2023-05-08 12:57:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.03e-09
2023-05-08 12:57:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.035e-09
2023-05-08 12:57:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.04e-09
2023-05-08 12:57:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.045e-09
2023-05-08 12:57:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.05e-09
2023-05-08 12:57:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.055e-09
2023-05-08 12:57:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.06e-09
2023-05-08 12:57:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.065e-09
2023-05-08 12:57:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.07e-09
2023-05-08 12:57:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.075e-09
2023-05-08 12:57:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.08e-09
2023-05-08 12:57:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.085e-09
2023-05-08 12:57:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.09e-09
2023-05-08 12:57:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.095e-09
2023-05-08 12:57:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.1e-09
2023-05-08 12:57:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.105e-09
2023-05-08 12:57:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.11e-09
2023-05-08 12:57:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.115e-09
2023-05-08 12:57:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.12e-09
2023-05-08 12:57:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.125e-09
2023-05-08 12:57:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.13e-09
2023-05-08 12:57:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.135e-09
2023-05-08 12:57:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.14e-09
2023-05-08 12:57:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.145e-09
2023-05-08 12:57:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.15e-09
2023-05-08 12:57:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.155e-09
2023-05-08 12:57:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.16e-09
2023-05-08 12:57:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.165e-09
2023-05-08 12:57:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.17e-09
2023-05-08 12:57:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.175e-09
2023-05-08 12:57:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.18e-09
2023-05-08 12:57:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.185e-09
2023-05-08 12:57:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.19e-09
2023-05-08 12:57:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.195e-09
2023-05-08 12:57:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.2e-09
2023-05-08 12:57:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.205e-09
2023-05-08 12:57:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.21e-09
2023-05-08 12:57:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.215e-09
2023-05-08 12:57:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.22e-09
2023-05-08 12:57:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.225e-09
2023-05-08 12:57:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.23e-09
2023-05-08 12:57:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.235e-09
2023-05-08 12:57:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.24e-09
2023-05-08 12:57:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.245e-09
2023-05-08 12:57:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.25e-09
2023-05-08 12:57:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.255e-09
2023-05-08 12:57:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.26e-09
2023-05-08 12:57:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.265e-09
2023-05-08 12:57:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.27e-09
2023-05-08 12:57:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.275e-09
2023-05-08 12:57:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.28e-09
2023-05-08 12:57:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.285e-09
2023-05-08 12:57:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.29e-09
2023-05-08 12:57:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.295e-09
2023-05-08 12:57:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.3e-09
2023-05-08 12:57:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.305e-09
2023-05-08 12:57:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.31e-09
2023-05-08 12:57:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.315e-09
2023-05-08 12:57:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.32e-09
2023-05-08 12:57:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.325e-09
2023-05-08 12:57:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.33e-09
2023-05-08 12:57:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.335e-09
2023-05-08 12:57:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.34e-09
2023-05-08 12:57:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.345e-09
2023-05-08 12:57:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.35e-09
2023-05-08 12:57:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.355e-09
2023-05-08 12:57:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.36e-09
2023-05-08 12:57:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.365e-09
2023-05-08 12:57:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.37e-09
2023-05-08 12:57:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.375e-09
2023-05-08 12:57:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.38e-09
2023-05-08 12:57:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.385e-09
2023-05-08 12:57:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.39e-09
2023-05-08 12:57:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.395e-09
2023-05-08 12:57:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.4e-09
2023-05-08 12:57:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.405e-09
2023-05-08 12:57:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.41e-09
2023-05-08 12:57:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.415e-09
2023-05-08 12:57:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.42e-09
2023-05-08 12:57:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.425e-09
2023-05-08 12:57:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.43e-09
2023-05-08 12:57:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.435e-09
2023-05-08 12:57:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.44e-09
2023-05-08 12:57:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.445e-09
2023-05-08 12:57:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.45e-09
2023-05-08 12:57:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.455e-09
2023-05-08 12:57:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.46e-09
2023-05-08 12:57:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.465e-09
2023-05-08 12:57:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.47e-09
2023-05-08 12:57:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.475e-09
2023-05-08 12:57:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.48e-09
2023-05-08 12:57:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.485e-09
2023-05-08 12:57:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.49e-09
2023-05-08 12:57:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.495e-09
2023-05-08 12:57:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.5e-09
2023-05-08 12:57:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.505e-09
2023-05-08 12:57:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.51e-09
2023-05-08 12:57:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.515e-09
2023-05-08 12:57:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.52e-09
2023-05-08 12:57:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.525e-09
2023-05-08 12:57:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.53e-09
2023-05-08 12:57:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.535e-09
2023-05-08 12:57:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.54e-09
2023-05-08 12:57:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.545e-09
2023-05-08 12:57:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.55e-09
2023-05-08 12:57:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.555e-09
2023-05-08 12:57:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.56e-09
2023-05-08 12:57:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.565e-09
2023-05-08 12:57:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.57e-09
2023-05-08 12:57:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.575e-09
2023-05-08 12:57:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.58e-09
2023-05-08 12:57:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.585e-09
2023-05-08 12:57:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.59e-09
2023-05-08 12:57:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.595e-09
2023-05-08 12:57:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.6e-09
2023-05-08 12:57:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.605e-09
2023-05-08 12:57:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.61e-09
2023-05-08 12:57:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.615e-09
2023-05-08 12:57:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.62e-09
2023-05-08 12:57:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.625e-09
2023-05-08 12:57:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.63e-09
2023-05-08 12:57:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.635e-09
2023-05-08 12:57:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.64e-09
2023-05-08 12:57:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.645e-09
2023-05-08 12:57:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.65e-09
2023-05-08 12:57:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.655e-09
2023-05-08 12:57:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.66e-09
2023-05-08 12:57:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.665e-09
2023-05-08 12:57:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.67e-09
2023-05-08 12:57:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.675e-09
2023-05-08 12:57:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.68e-09
2023-05-08 12:57:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.685e-09
2023-05-08 12:57:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.69e-09
2023-05-08 12:57:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.695e-09
2023-05-08 12:57:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.7e-09
2023-05-08 12:57:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.705e-09
2023-05-08 12:57:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.71e-09
2023-05-08 12:57:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.715e-09
2023-05-08 12:57:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.72e-09
2023-05-08 12:57:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.725e-09
2023-05-08 12:57:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.73e-09
2023-05-08 12:57:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.735e-09
2023-05-08 12:57:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.74e-09
2023-05-08 12:57:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.745e-09
2023-05-08 12:57:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.75e-09
2023-05-08 12:57:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.755e-09
2023-05-08 12:57:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.76e-09
2023-05-08 12:58:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.765e-09
2023-05-08 12:58:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.77e-09
2023-05-08 12:58:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.775e-09
2023-05-08 12:58:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.78e-09
2023-05-08 12:58:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.785e-09
2023-05-08 12:58:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.79e-09
2023-05-08 12:58:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.795e-09
2023-05-08 12:58:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.8e-09
2023-05-08 12:58:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.805e-09
2023-05-08 12:58:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.81e-09
2023-05-08 12:58:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.815e-09
2023-05-08 12:58:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.82e-09
2023-05-08 12:58:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.825e-09
2023-05-08 12:58:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.83e-09
2023-05-08 12:58:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.835e-09
2023-05-08 12:58:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.84e-09
2023-05-08 12:58:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.845e-09
2023-05-08 12:58:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.85e-09
2023-05-08 12:58:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.855e-09
2023-05-08 12:58:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.86e-09
2023-05-08 12:58:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.865e-09
2023-05-08 12:58:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.87e-09
2023-05-08 12:58:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.875e-09
2023-05-08 12:58:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.88e-09
2023-05-08 12:58:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.885e-09
2023-05-08 12:58:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.89e-09
2023-05-08 12:58:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.895e-09
2023-05-08 12:58:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.9e-09
2023-05-08 12:58:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.905e-09
2023-05-08 12:58:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.91e-09
2023-05-08 12:58:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.915e-09
2023-05-08 12:58:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.92e-09
2023-05-08 12:58:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.925e-09
2023-05-08 12:58:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.93e-09
2023-05-08 12:58:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.935e-09
2023-05-08 12:58:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.94e-09
2023-05-08 12:58:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.945e-09
2023-05-08 12:58:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.95e-09
2023-05-08 12:58:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.955e-09
2023-05-08 12:58:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.96e-09
2023-05-08 12:58:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.965e-09
2023-05-08 12:58:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.97e-09
2023-05-08 12:58:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.975e-09
2023-05-08 12:58:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.98e-09
2023-05-08 12:58:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.985e-09
2023-05-08 12:58:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.99e-09
2023-05-08 12:58:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=7.995e-09
2023-05-08 12:58:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=8e-09
2023-05-08 12:58:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.005e-09
2023-05-08 12:58:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.01e-09
2023-05-08 12:58:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.015e-09
2023-05-08 12:58:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.02e-09
2023-05-08 12:58:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.025e-09
2023-05-08 12:58:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.03e-09
2023-05-08 12:58:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.035e-09
2023-05-08 12:58:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.04e-09
2023-05-08 12:58:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.045e-09
2023-05-08 12:58:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.05e-09
2023-05-08 12:58:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.055e-09
2023-05-08 12:58:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.06e-09
2023-05-08 12:58:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.065e-09
2023-05-08 12:58:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.07e-09
2023-05-08 12:58:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.075e-09
2023-05-08 12:58:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.08e-09
2023-05-08 12:58:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.085e-09
2023-05-08 12:58:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.09e-09
2023-05-08 12:58:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.095e-09
2023-05-08 12:58:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.1e-09
2023-05-08 12:58:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.105e-09
2023-05-08 12:58:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.11e-09
2023-05-08 12:58:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.115e-09
2023-05-08 12:58:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.12e-09
2023-05-08 12:58:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.125e-09
2023-05-08 12:58:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.13e-09
2023-05-08 12:58:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.135e-09
2023-05-08 12:58:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.14e-09
2023-05-08 12:58:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.145e-09
2023-05-08 12:58:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.15e-09
2023-05-08 12:58:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.155e-09
2023-05-08 12:58:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.16e-09
2023-05-08 12:58:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.165e-09
2023-05-08 12:58:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.17e-09
2023-05-08 12:58:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.175e-09
2023-05-08 12:58:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.18e-09
2023-05-08 12:58:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.185e-09
2023-05-08 12:58:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.19e-09
2023-05-08 12:58:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.195e-09
2023-05-08 12:58:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.2e-09
2023-05-08 12:58:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.205e-09
2023-05-08 12:58:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.21e-09
2023-05-08 12:58:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.215e-09
2023-05-08 12:58:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.22e-09
2023-05-08 12:58:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.225e-09
2023-05-08 12:58:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.23e-09
2023-05-08 12:58:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.235e-09
2023-05-08 12:58:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.24e-09
2023-05-08 12:58:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.245e-09
2023-05-08 12:58:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.25e-09
2023-05-08 12:58:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.255e-09
2023-05-08 12:58:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.26e-09
2023-05-08 12:58:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.265e-09
2023-05-08 12:58:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.27e-09
2023-05-08 12:58:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.275e-09
2023-05-08 12:58:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.28e-09
2023-05-08 12:58:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.285e-09
2023-05-08 12:58:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.29e-09
2023-05-08 12:58:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.295e-09
2023-05-08 12:58:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.3e-09
2023-05-08 12:58:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.305e-09
2023-05-08 12:58:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.31e-09
2023-05-08 12:58:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.315e-09
2023-05-08 12:58:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.32e-09
2023-05-08 12:58:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.325e-09
2023-05-08 12:58:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.33e-09
2023-05-08 12:58:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.335e-09
2023-05-08 12:58:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.34e-09
2023-05-08 12:58:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.345e-09
2023-05-08 12:58:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.35e-09
2023-05-08 12:58:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.355e-09
2023-05-08 12:58:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.36e-09
2023-05-08 12:58:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.365e-09
2023-05-08 12:58:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.37e-09
2023-05-08 12:58:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.375e-09
2023-05-08 12:58:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.38e-09
2023-05-08 12:58:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.385e-09
2023-05-08 12:58:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.39e-09
2023-05-08 12:58:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.395e-09
2023-05-08 12:58:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.4e-09
2023-05-08 12:58:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.405e-09
2023-05-08 12:58:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.41e-09
2023-05-08 12:58:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.415e-09
2023-05-08 12:58:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.42e-09
2023-05-08 12:58:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.425e-09
2023-05-08 12:58:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.43e-09
2023-05-08 12:58:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.435e-09
2023-05-08 12:58:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.44e-09
2023-05-08 12:58:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.445e-09
2023-05-08 12:58:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.45e-09
2023-05-08 12:58:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.455e-09
2023-05-08 12:58:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.46e-09
2023-05-08 12:58:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.465e-09
2023-05-08 12:58:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.47e-09
2023-05-08 12:58:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.475e-09
2023-05-08 12:58:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.48e-09
2023-05-08 12:58:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.485e-09
2023-05-08 12:58:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.49e-09
2023-05-08 12:58:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.495e-09
2023-05-08 12:58:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.5e-09
2023-05-08 12:58:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.505e-09
2023-05-08 12:58:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.51e-09
2023-05-08 12:58:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.515e-09
2023-05-08 12:58:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.52e-09
2023-05-08 12:58:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.525e-09
2023-05-08 12:58:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.53e-09
2023-05-08 12:58:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.535e-09
2023-05-08 12:58:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.54e-09
2023-05-08 12:58:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.545e-09
2023-05-08 12:58:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.55e-09
2023-05-08 12:58:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.555e-09
2023-05-08 12:58:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.56e-09
2023-05-08 12:58:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.565e-09
2023-05-08 12:58:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.57e-09
2023-05-08 12:58:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.575e-09
2023-05-08 12:58:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.58e-09
2023-05-08 12:58:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.585e-09
2023-05-08 12:58:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.59e-09
2023-05-08 12:58:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.595e-09
2023-05-08 12:58:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.6e-09
2023-05-08 12:58:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.605e-09
2023-05-08 12:58:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.61e-09
2023-05-08 12:58:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.615e-09
2023-05-08 12:58:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.62e-09
2023-05-08 12:58:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.625e-09
2023-05-08 12:58:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.63e-09
2023-05-08 12:58:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.635e-09
2023-05-08 12:58:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.64e-09
2023-05-08 12:58:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.645e-09
2023-05-08 12:58:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.65e-09
2023-05-08 12:58:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.655e-09
2023-05-08 12:58:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.66e-09
2023-05-08 12:58:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.665e-09
2023-05-08 12:58:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.67e-09
2023-05-08 12:58:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.675e-09
2023-05-08 12:58:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.68e-09
2023-05-08 12:58:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.685e-09
2023-05-08 12:58:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.69e-09
2023-05-08 12:58:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.695e-09
2023-05-08 12:58:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.7e-09
2023-05-08 12:58:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.705e-09
2023-05-08 12:58:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.71e-09
2023-05-08 12:58:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.715e-09
2023-05-08 12:58:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.72e-09
2023-05-08 12:58:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.725e-09
2023-05-08 12:58:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.73e-09
2023-05-08 12:58:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.735e-09
2023-05-08 12:58:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.74e-09
2023-05-08 12:59:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.745e-09
2023-05-08 12:59:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.75e-09
2023-05-08 12:59:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.755e-09
2023-05-08 12:59:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.76e-09
2023-05-08 12:59:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.765e-09
2023-05-08 12:59:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.77e-09
2023-05-08 12:59:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.775e-09
2023-05-08 12:59:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.78e-09
2023-05-08 12:59:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.785e-09
2023-05-08 12:59:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.79e-09
2023-05-08 12:59:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.795e-09
2023-05-08 12:59:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.8e-09
2023-05-08 12:59:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.805e-09
2023-05-08 12:59:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.81e-09
2023-05-08 12:59:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.815e-09
2023-05-08 12:59:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.82e-09
2023-05-08 12:59:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.825e-09
2023-05-08 12:59:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.83e-09
2023-05-08 12:59:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.835e-09
2023-05-08 12:59:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.84e-09
2023-05-08 12:59:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.845e-09
2023-05-08 12:59:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.85e-09
2023-05-08 12:59:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.855e-09
2023-05-08 12:59:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.86e-09
2023-05-08 12:59:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.865e-09
2023-05-08 12:59:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.87e-09
2023-05-08 12:59:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.875e-09
2023-05-08 12:59:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.88e-09
2023-05-08 12:59:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.885e-09
2023-05-08 12:59:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.89e-09
2023-05-08 12:59:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.895e-09
2023-05-08 12:59:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.9e-09
2023-05-08 12:59:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.905e-09
2023-05-08 12:59:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.91e-09
2023-05-08 12:59:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.915e-09
2023-05-08 12:59:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.92e-09
2023-05-08 12:59:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.925e-09
2023-05-08 12:59:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.93e-09
2023-05-08 12:59:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.935e-09
2023-05-08 12:59:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.94e-09
2023-05-08 12:59:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.945e-09
2023-05-08 12:59:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.95e-09
2023-05-08 12:59:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.955e-09
2023-05-08 12:59:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.96e-09
2023-05-08 12:59:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.965e-09
2023-05-08 12:59:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.97e-09
2023-05-08 12:59:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.975e-09
2023-05-08 12:59:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.98e-09
2023-05-08 12:59:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.985e-09
2023-05-08 12:59:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.99e-09
2023-05-08 12:59:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=8.995e-09
2023-05-08 12:59:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=9e-09
2023-05-08 12:59:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.005e-09
2023-05-08 12:59:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.01e-09
2023-05-08 12:59:17 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.015e-09
2023-05-08 12:59:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.02e-09
2023-05-08 12:59:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.025e-09
2023-05-08 12:59:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.03e-09
2023-05-08 12:59:18 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.035e-09
2023-05-08 12:59:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.04e-09
2023-05-08 12:59:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.045e-09
2023-05-08 12:59:19 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.05e-09
2023-05-08 12:59:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.055e-09
2023-05-08 12:59:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.06e-09
2023-05-08 12:59:20 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.065e-09
2023-05-08 12:59:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.07e-09
2023-05-08 12:59:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.075e-09
2023-05-08 12:59:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.08e-09
2023-05-08 12:59:21 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.085e-09
2023-05-08 12:59:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.09e-09
2023-05-08 12:59:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.095e-09
2023-05-08 12:59:22 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.1e-09
2023-05-08 12:59:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.105e-09
2023-05-08 12:59:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.11e-09
2023-05-08 12:59:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.115e-09
2023-05-08 12:59:23 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.12e-09
2023-05-08 12:59:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.125e-09
2023-05-08 12:59:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.13e-09
2023-05-08 12:59:24 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.135e-09
2023-05-08 12:59:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.14e-09
2023-05-08 12:59:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.145e-09
2023-05-08 12:59:25 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.15e-09
2023-05-08 12:59:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.155e-09
2023-05-08 12:59:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.16e-09
2023-05-08 12:59:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.165e-09
2023-05-08 12:59:26 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.17e-09
2023-05-08 12:59:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.175e-09
2023-05-08 12:59:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.18e-09
2023-05-08 12:59:27 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.185e-09
2023-05-08 12:59:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.19e-09
2023-05-08 12:59:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.195e-09
2023-05-08 12:59:28 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.2e-09
2023-05-08 12:59:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.205e-09
2023-05-08 12:59:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.21e-09
2023-05-08 12:59:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.215e-09
2023-05-08 12:59:29 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.22e-09
2023-05-08 12:59:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.225e-09
2023-05-08 12:59:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.23e-09
2023-05-08 12:59:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.235e-09
2023-05-08 12:59:30 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.24e-09
2023-05-08 12:59:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.245e-09
2023-05-08 12:59:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.25e-09
2023-05-08 12:59:31 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.255e-09
2023-05-08 12:59:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.26e-09
2023-05-08 12:59:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.265e-09
2023-05-08 12:59:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.27e-09
2023-05-08 12:59:32 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.275e-09
2023-05-08 12:59:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.28e-09
2023-05-08 12:59:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.285e-09
2023-05-08 12:59:33 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.29e-09
2023-05-08 12:59:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.295e-09
2023-05-08 12:59:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.3e-09
2023-05-08 12:59:34 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.305e-09
2023-05-08 12:59:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.31e-09
2023-05-08 12:59:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.315e-09
2023-05-08 12:59:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.32e-09
2023-05-08 12:59:35 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.325e-09
2023-05-08 12:59:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.33e-09
2023-05-08 12:59:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.335e-09
2023-05-08 12:59:36 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.34e-09
2023-05-08 12:59:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.345e-09
2023-05-08 12:59:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.35e-09
2023-05-08 12:59:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.355e-09
2023-05-08 12:59:37 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.36e-09
2023-05-08 12:59:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.365e-09
2023-05-08 12:59:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.37e-09
2023-05-08 12:59:38 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.375e-09
2023-05-08 12:59:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.38e-09
2023-05-08 12:59:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.385e-09
2023-05-08 12:59:39 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.39e-09
2023-05-08 12:59:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.395e-09
2023-05-08 12:59:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.4e-09
2023-05-08 12:59:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.405e-09
2023-05-08 12:59:40 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.41e-09
2023-05-08 12:59:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.415e-09
2023-05-08 12:59:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.42e-09
2023-05-08 12:59:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.425e-09
2023-05-08 12:59:41 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.43e-09
2023-05-08 12:59:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.435e-09
2023-05-08 12:59:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.44e-09
2023-05-08 12:59:42 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.445e-09
2023-05-08 12:59:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.45e-09
2023-05-08 12:59:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.455e-09
2023-05-08 12:59:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.46e-09
2023-05-08 12:59:43 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.465e-09
2023-05-08 12:59:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.47e-09
2023-05-08 12:59:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.475e-09
2023-05-08 12:59:44 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.48e-09
2023-05-08 12:59:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.485e-09
2023-05-08 12:59:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.49e-09
2023-05-08 12:59:45 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.495e-09
2023-05-08 12:59:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.5e-09
2023-05-08 12:59:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.505e-09
2023-05-08 12:59:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.51e-09
2023-05-08 12:59:46 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.515e-09
2023-05-08 12:59:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.52e-09
2023-05-08 12:59:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.525e-09
2023-05-08 12:59:47 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.53e-09
2023-05-08 12:59:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.535e-09
2023-05-08 12:59:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.54e-09
2023-05-08 12:59:48 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.545e-09
2023-05-08 12:59:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.55e-09
2023-05-08 12:59:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.555e-09
2023-05-08 12:59:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.56e-09
2023-05-08 12:59:49 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.565e-09
2023-05-08 12:59:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.57e-09
2023-05-08 12:59:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.575e-09
2023-05-08 12:59:50 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.58e-09
2023-05-08 12:59:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.585e-09
2023-05-08 12:59:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.59e-09
2023-05-08 12:59:51 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.595e-09
2023-05-08 12:59:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.6e-09
2023-05-08 12:59:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.605e-09
2023-05-08 12:59:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.61e-09
2023-05-08 12:59:52 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.615e-09
2023-05-08 12:59:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.62e-09
2023-05-08 12:59:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.625e-09
2023-05-08 12:59:53 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.63e-09
2023-05-08 12:59:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.635e-09
2023-05-08 12:59:54 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.64e-09
2023-05-08 12:59:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.645e-09
2023-05-08 12:59:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.65e-09
2023-05-08 12:59:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.655e-09
2023-05-08 12:59:55 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.66e-09
2023-05-08 12:59:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.665e-09
2023-05-08 12:59:56 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.67e-09
2023-05-08 12:59:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.675e-09
2023-05-08 12:59:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.68e-09
2023-05-08 12:59:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.685e-09
2023-05-08 12:59:57 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.69e-09
2023-05-08 12:59:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.695e-09
2023-05-08 12:59:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.7e-09
2023-05-08 12:59:58 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.705e-09
2023-05-08 12:59:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.71e-09
2023-05-08 12:59:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.715e-09
2023-05-08 12:59:59 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.72e-09
2023-05-08 13:00:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.725e-09
2023-05-08 13:00:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.73e-09
2023-05-08 13:00:00 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.735e-09
2023-05-08 13:00:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.74e-09
2023-05-08 13:00:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.745e-09
2023-05-08 13:00:01 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.75e-09
2023-05-08 13:00:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.755e-09
2023-05-08 13:00:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.76e-09
2023-05-08 13:00:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.765e-09
2023-05-08 13:00:02 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.77e-09
2023-05-08 13:00:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.775e-09
2023-05-08 13:00:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.78e-09
2023-05-08 13:00:03 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.785e-09
2023-05-08 13:00:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.79e-09
2023-05-08 13:00:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.795e-09
2023-05-08 13:00:04 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.8e-09
2023-05-08 13:00:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.805e-09
2023-05-08 13:00:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.81e-09
2023-05-08 13:00:05 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.815e-09
2023-05-08 13:00:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.82e-09
2023-05-08 13:00:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.825e-09
2023-05-08 13:00:06 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.83e-09
2023-05-08 13:00:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.835e-09
2023-05-08 13:00:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.84e-09
2023-05-08 13:00:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.845e-09
2023-05-08 13:00:07 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.85e-09
2023-05-08 13:00:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.855e-09
2023-05-08 13:00:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.86e-09
2023-05-08 13:00:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.865e-09
2023-05-08 13:00:08 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.87e-09
2023-05-08 13:00:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.875e-09
2023-05-08 13:00:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.88e-09
2023-05-08 13:00:09 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.885e-09
2023-05-08 13:00:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.89e-09
2023-05-08 13:00:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.895e-09
2023-05-08 13:00:10 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.9e-09
2023-05-08 13:00:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.905e-09
2023-05-08 13:00:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.91e-09
2023-05-08 13:00:11 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.915e-09
2023-05-08 13:00:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.92e-09
2023-05-08 13:00:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.925e-09
2023-05-08 13:00:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.93e-09
2023-05-08 13:00:12 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.935e-09
2023-05-08 13:00:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.94e-09
2023-05-08 13:00:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.945e-09
2023-05-08 13:00:13 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.95e-09
2023-05-08 13:00:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.955e-09
2023-05-08 13:00:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.96e-09
2023-05-08 13:00:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.965e-09
2023-05-08 13:00:14 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.97e-09
2023-05-08 13:00:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.975e-09
2023-05-08 13:00:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.98e-09
2023-05-08 13:00:15 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.985e-09
2023-05-08 13:00:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.99e-09
2023-05-08 13:00:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=9.995e-09
2023-05-08 13:00:16 magnum.np:INFO [LLG] step: dt= 5e-12 t=1e-08
=====================================================================
TIMER REPORT
=====================================================================
Operation No of calls Avg time [ms] Total time [s]
------------------- ------------- --------------- ----------------
LLGSolver.relax 1 65323.9 65.3239
DemagField.h 2053 17.3304 35.5794
ExchangeField.h 2053 12.9098 26.5039
ExternalField.h 2053 0.176763 0.362895
LLGSolver.step 2000 261.859 523.718
DemagField.h 70020 5.18752 363.23
ExchangeField.h 70020 0.523453 36.6522
ExternalField.h 70020 0.160674 11.2504
------------------- ------------- --------------- ----------------
Total 722.954
Missing 133.912
=====================================================================
Plot Results:
[3]:
from os import path
if not path.isdir("ref"):
!mkdir ref
!wget -P ref https://gitlab.com/magnum.np/magnum.np/raw/main/demos/sp_FMR/ref/m_ref.dat
[4]:
import numpy as np
import matplotlib.pyplot as plt
data = np.loadtxt("data/log.dat")
data_ref = np.loadtxt("ref/m_ref.dat")
t, mx, my, mz = data.T
t_ref, mx_ref, my_ref, mz_ref = data_ref.T
sy = abs((np.fft.fft(my)**2.))
sy_ref = abs((np.fft.fft(my_ref)**2.))
f = np.fft.fftfreq(len(t), t[1]-t[0])*1e-9
f_ref = np.fft.fftfreq(len(t_ref), t_ref[1]-t_ref[0])*1e-9
fig, (ax0, ax1) = plt.subplots(2,1)
cycle = plt.rcParams['axes.prop_cycle'].by_key()['color']
ax0.plot(t*1e9, my, color=cycle[0])
ax0.plot(t_ref*1e9, my_ref, '-', color=cycle[0], linewidth=6, alpha=0.4)
ax0.set_xlabel("Time (ns)")
ax0.set_ylabel("$m_y (A/m)$")
ax0.set_xlim(0, 2.5)
ax0.set_ylim(0.580, 0.594)
ax0.grid()
ax1.plot(f[2: -len(f)//2], sy[2: -len(f)//2], '-', color=cycle[1])
ax1.plot(f_ref[2: -len(f_ref)//2], sy_ref[2: -len(f_ref)//2], '-', color=cycle[1], linewidth=6, alpha=0.4)
ax1.set_yscale("log")
ax1.set_xlabel("Frequency (GHz)")
ax1.set_ylabel("$m_y (A/m)$")
ax1.set_xlim(0, 20)
ax1.grid()
fig.tight_layout()
fig.savefig("data/results.png")
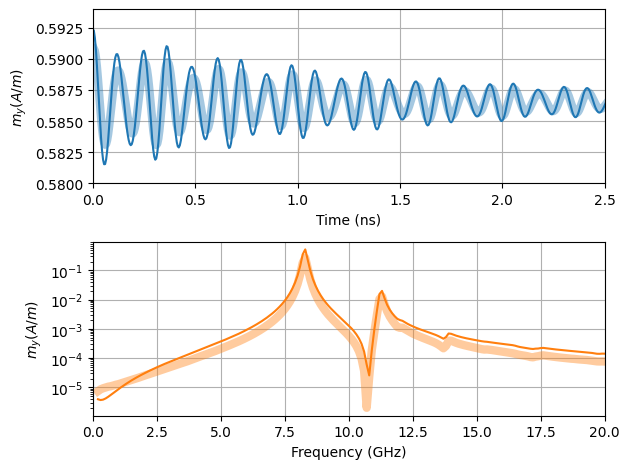