Standard Problem Domainwall Pinning
Google Colab Link
The demo can be run on Google Colab without any local installation. Use the following link to try it out.
[12]:
!pip install -q magnumnp numpy==1.22.4
Run Demo:
[13]:
from magnumnp import *
import numpy as np
Timer.enable()
Hextmax=2.5/constants.mu_0
Hextmin=0.0/constants.mu_0
tfinal = 20e-9
n = (80, 1, 1)
dx = (1e-9, 1e-9, 1e-9)
origin = (-n[0]*dx[0]/2., -n[1]*dx[1]/2., -n[2]*dx[2]/2.,)
mesh = Mesh(n, dx, origin)
state = State(mesh)
state.material = {"alpha": 1.}
x, y, z = state.SpatialCoordinate()
soft = (x < 0)
hard = (x >= 0)
Ms = state.Constant([0.0])
Ms[soft] = 0.25/constants.mu_0
Ms[hard] = 1./constants.mu_0
Ku = state.Constant([0.0])
Ku[soft] = 1e5
Ku[hard] = 1e6
A = state.Constant([0.0])
A[soft] = 0.25e-11
A[hard] = 1.e-11
state.material['Ms'] = Ms
state.material['A'] = A
state.material['Ku'] = Ku
state.material['Ku_axis'] = [0,1,0]
state.m = state.Constant([np.sin(0.3), np.cos(0.3), 0.0])
state.m[hard] = state.Tensor([0.0, -1.0, 0.0])
state.m.normalize()
exchange = ExchangeField()
aniso = UniaxialAnisotropyField()
external = ExternalField(lambda t: state.Constant([0, (Hextmax-Hextmin)*t/tfinal+Hextmin, 0]))
llg = LLGSolver([exchange, aniso, external])
logger = ScalarLogger("data/m.dat", ['t', external.h, 'm'])
while state.t < tfinal:
llg.step(state, 1e-11)
logger << state
Timer.print_report()
2023-05-08 12:47:09 magnum.np:INFO [State] running on device: cpu (dtype = float64)
2023-05-08 12:47:09 magnum.np:INFO [Mesh] 80x1x1 (size= 1e-09 x 1e-09 x 1e-09)
2023-05-08 12:47:09 magnum.np:INFO [LLGSolver] using RKF45 solver (atol = 1e-05)
2023-05-08 12:47:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1e-11
2023-05-08 12:47:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=2e-11
2023-05-08 12:47:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=3e-11
2023-05-08 12:47:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=4e-11
2023-05-08 12:47:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=5e-11
2023-05-08 12:47:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=6e-11
2023-05-08 12:47:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=7e-11
2023-05-08 12:47:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=8e-11
2023-05-08 12:47:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=9e-11
2023-05-08 12:47:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1e-10
2023-05-08 12:47:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.1e-10
2023-05-08 12:47:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.2e-10
2023-05-08 12:47:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.3e-10
2023-05-08 12:47:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.4e-10
2023-05-08 12:47:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.5e-10
2023-05-08 12:47:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.6e-10
2023-05-08 12:47:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.7e-10
2023-05-08 12:47:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.8e-10
2023-05-08 12:47:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.9e-10
2023-05-08 12:47:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=2e-10
2023-05-08 12:47:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.1e-10
2023-05-08 12:47:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.2e-10
2023-05-08 12:47:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.3e-10
2023-05-08 12:47:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.4e-10
2023-05-08 12:47:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.5e-10
2023-05-08 12:47:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.6e-10
2023-05-08 12:47:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.7e-10
2023-05-08 12:47:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.8e-10
2023-05-08 12:47:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.9e-10
2023-05-08 12:47:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=3e-10
2023-05-08 12:47:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.1e-10
2023-05-08 12:47:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.2e-10
2023-05-08 12:47:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.3e-10
2023-05-08 12:47:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.4e-10
2023-05-08 12:47:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.5e-10
2023-05-08 12:47:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.6e-10
2023-05-08 12:47:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.7e-10
2023-05-08 12:47:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.8e-10
2023-05-08 12:47:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.9e-10
2023-05-08 12:47:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=4e-10
2023-05-08 12:47:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.1e-10
2023-05-08 12:47:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.2e-10
2023-05-08 12:47:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.3e-10
2023-05-08 12:47:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.4e-10
2023-05-08 12:47:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.5e-10
2023-05-08 12:47:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.6e-10
2023-05-08 12:47:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.7e-10
2023-05-08 12:47:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.8e-10
2023-05-08 12:47:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.9e-10
2023-05-08 12:47:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=5e-10
2023-05-08 12:47:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.1e-10
2023-05-08 12:47:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.2e-10
2023-05-08 12:47:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.3e-10
2023-05-08 12:47:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.4e-10
2023-05-08 12:47:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.5e-10
2023-05-08 12:47:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.6e-10
2023-05-08 12:47:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.7e-10
2023-05-08 12:47:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.8e-10
2023-05-08 12:47:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.9e-10
2023-05-08 12:47:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=6e-10
2023-05-08 12:47:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.1e-10
2023-05-08 12:47:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.2e-10
2023-05-08 12:47:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.3e-10
2023-05-08 12:47:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.4e-10
2023-05-08 12:47:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.5e-10
2023-05-08 12:47:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.6e-10
2023-05-08 12:47:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.7e-10
2023-05-08 12:48:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.8e-10
2023-05-08 12:48:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.9e-10
2023-05-08 12:48:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=7e-10
2023-05-08 12:48:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.1e-10
2023-05-08 12:48:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.2e-10
2023-05-08 12:48:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.3e-10
2023-05-08 12:48:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.4e-10
2023-05-08 12:48:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.5e-10
2023-05-08 12:48:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.6e-10
2023-05-08 12:48:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.7e-10
2023-05-08 12:48:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.8e-10
2023-05-08 12:48:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.9e-10
2023-05-08 12:48:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=8e-10
2023-05-08 12:48:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.1e-10
2023-05-08 12:48:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.2e-10
2023-05-08 12:48:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.3e-10
2023-05-08 12:48:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.4e-10
2023-05-08 12:48:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.5e-10
2023-05-08 12:48:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.6e-10
2023-05-08 12:48:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.7e-10
2023-05-08 12:48:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.8e-10
2023-05-08 12:48:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.9e-10
2023-05-08 12:48:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=9e-10
2023-05-08 12:48:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.1e-10
2023-05-08 12:48:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.2e-10
2023-05-08 12:48:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.3e-10
2023-05-08 12:48:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.4e-10
2023-05-08 12:48:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.5e-10
2023-05-08 12:48:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.6e-10
2023-05-08 12:48:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.7e-10
2023-05-08 12:48:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.8e-10
2023-05-08 12:48:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.9e-10
2023-05-08 12:48:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1e-09
2023-05-08 12:48:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.01e-09
2023-05-08 12:48:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.02e-09
2023-05-08 12:48:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.03e-09
2023-05-08 12:48:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.04e-09
2023-05-08 12:48:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.05e-09
2023-05-08 12:48:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.06e-09
2023-05-08 12:48:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.07e-09
2023-05-08 12:48:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.08e-09
2023-05-08 12:48:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.09e-09
2023-05-08 12:48:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.1e-09
2023-05-08 12:48:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.11e-09
2023-05-08 12:48:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.12e-09
2023-05-08 12:48:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.13e-09
2023-05-08 12:48:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.14e-09
2023-05-08 12:48:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.15e-09
2023-05-08 12:48:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.16e-09
2023-05-08 12:48:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.17e-09
2023-05-08 12:48:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.18e-09
2023-05-08 12:48:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.19e-09
2023-05-08 12:48:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.2e-09
2023-05-08 12:48:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.21e-09
2023-05-08 12:48:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.22e-09
2023-05-08 12:48:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.23e-09
2023-05-08 12:48:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.24e-09
2023-05-08 12:48:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.25e-09
2023-05-08 12:48:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.26e-09
2023-05-08 12:48:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.27e-09
2023-05-08 12:48:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.28e-09
2023-05-08 12:48:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.29e-09
2023-05-08 12:48:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.3e-09
2023-05-08 12:48:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.31e-09
2023-05-08 12:48:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.32e-09
2023-05-08 12:48:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.33e-09
2023-05-08 12:48:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.34e-09
2023-05-08 12:49:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.35e-09
2023-05-08 12:49:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.36e-09
2023-05-08 12:49:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.37e-09
2023-05-08 12:49:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.38e-09
2023-05-08 12:49:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.39e-09
2023-05-08 12:49:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.4e-09
2023-05-08 12:49:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.41e-09
2023-05-08 12:49:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.42e-09
2023-05-08 12:49:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.43e-09
2023-05-08 12:49:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.44e-09
2023-05-08 12:49:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.45e-09
2023-05-08 12:49:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.46e-09
2023-05-08 12:49:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.47e-09
2023-05-08 12:49:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.48e-09
2023-05-08 12:49:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.49e-09
2023-05-08 12:49:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.5e-09
2023-05-08 12:49:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.51e-09
2023-05-08 12:49:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.52e-09
2023-05-08 12:49:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.53e-09
2023-05-08 12:49:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.54e-09
2023-05-08 12:49:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.55e-09
2023-05-08 12:49:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.56e-09
2023-05-08 12:49:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.57e-09
2023-05-08 12:49:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.58e-09
2023-05-08 12:49:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.59e-09
2023-05-08 12:49:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.6e-09
2023-05-08 12:49:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.61e-09
2023-05-08 12:49:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.62e-09
2023-05-08 12:49:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.63e-09
2023-05-08 12:49:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.64e-09
2023-05-08 12:49:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.65e-09
2023-05-08 12:49:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.66e-09
2023-05-08 12:49:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.67e-09
2023-05-08 12:49:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.68e-09
2023-05-08 12:49:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.69e-09
2023-05-08 12:49:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.7e-09
2023-05-08 12:49:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.71e-09
2023-05-08 12:49:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.72e-09
2023-05-08 12:49:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.73e-09
2023-05-08 12:49:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.74e-09
2023-05-08 12:49:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.75e-09
2023-05-08 12:49:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.76e-09
2023-05-08 12:49:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.77e-09
2023-05-08 12:49:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.78e-09
2023-05-08 12:49:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.79e-09
2023-05-08 12:49:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.8e-09
2023-05-08 12:49:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.81e-09
2023-05-08 12:49:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.82e-09
2023-05-08 12:49:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.83e-09
2023-05-08 12:49:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.84e-09
2023-05-08 12:49:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.85e-09
2023-05-08 12:49:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.86e-09
2023-05-08 12:49:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.87e-09
2023-05-08 12:49:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.88e-09
2023-05-08 12:49:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.89e-09
2023-05-08 12:49:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.9e-09
2023-05-08 12:49:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.91e-09
2023-05-08 12:49:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.92e-09
2023-05-08 12:49:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.93e-09
2023-05-08 12:49:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.94e-09
2023-05-08 12:49:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.95e-09
2023-05-08 12:49:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.96e-09
2023-05-08 12:49:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.97e-09
2023-05-08 12:49:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.98e-09
2023-05-08 12:49:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.99e-09
2023-05-08 12:49:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=2e-09
2023-05-08 12:49:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.01e-09
2023-05-08 12:49:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.02e-09
2023-05-08 12:49:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.03e-09
2023-05-08 12:49:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.04e-09
2023-05-08 12:49:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.05e-09
2023-05-08 12:49:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.06e-09
2023-05-08 12:49:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.07e-09
2023-05-08 12:49:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.08e-09
2023-05-08 12:49:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.09e-09
2023-05-08 12:49:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.1e-09
2023-05-08 12:49:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.11e-09
2023-05-08 12:50:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.12e-09
2023-05-08 12:50:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.13e-09
2023-05-08 12:50:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.14e-09
2023-05-08 12:50:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.15e-09
2023-05-08 12:50:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.16e-09
2023-05-08 12:50:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.17e-09
2023-05-08 12:50:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.18e-09
2023-05-08 12:50:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.19e-09
2023-05-08 12:50:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.2e-09
2023-05-08 12:50:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.21e-09
2023-05-08 12:50:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.22e-09
2023-05-08 12:50:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.23e-09
2023-05-08 12:50:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.24e-09
2023-05-08 12:50:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.25e-09
2023-05-08 12:50:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.26e-09
2023-05-08 12:50:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.27e-09
2023-05-08 12:50:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.28e-09
2023-05-08 12:50:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.29e-09
2023-05-08 12:50:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.3e-09
2023-05-08 12:50:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.31e-09
2023-05-08 12:50:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.32e-09
2023-05-08 12:50:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.33e-09
2023-05-08 12:50:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.34e-09
2023-05-08 12:50:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.35e-09
2023-05-08 12:50:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.36e-09
2023-05-08 12:50:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.37e-09
2023-05-08 12:50:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.38e-09
2023-05-08 12:50:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.39e-09
2023-05-08 12:50:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.4e-09
2023-05-08 12:50:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.41e-09
2023-05-08 12:50:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.42e-09
2023-05-08 12:50:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.43e-09
2023-05-08 12:50:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.44e-09
2023-05-08 12:50:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.45e-09
2023-05-08 12:50:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.46e-09
2023-05-08 12:50:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.47e-09
2023-05-08 12:50:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.48e-09
2023-05-08 12:50:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.49e-09
2023-05-08 12:50:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.5e-09
2023-05-08 12:50:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.51e-09
2023-05-08 12:50:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.52e-09
2023-05-08 12:50:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.53e-09
2023-05-08 12:50:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.54e-09
2023-05-08 12:50:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.55e-09
2023-05-08 12:50:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.56e-09
2023-05-08 12:50:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.57e-09
2023-05-08 12:50:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.58e-09
2023-05-08 12:50:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.59e-09
2023-05-08 12:50:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.6e-09
2023-05-08 12:50:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.61e-09
2023-05-08 12:50:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.62e-09
2023-05-08 12:50:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.63e-09
2023-05-08 12:50:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.64e-09
2023-05-08 12:50:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.65e-09
2023-05-08 12:50:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.66e-09
2023-05-08 12:50:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.67e-09
2023-05-08 12:50:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.68e-09
2023-05-08 12:50:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.69e-09
2023-05-08 12:50:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.7e-09
2023-05-08 12:50:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.71e-09
2023-05-08 12:50:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.72e-09
2023-05-08 12:50:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.73e-09
2023-05-08 12:50:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.74e-09
2023-05-08 12:50:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.75e-09
2023-05-08 12:50:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.76e-09
2023-05-08 12:50:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.77e-09
2023-05-08 12:50:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.78e-09
2023-05-08 12:50:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.79e-09
2023-05-08 12:50:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.8e-09
2023-05-08 12:50:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.81e-09
2023-05-08 12:50:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.82e-09
2023-05-08 12:50:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.83e-09
2023-05-08 12:50:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.84e-09
2023-05-08 12:50:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.85e-09
2023-05-08 12:50:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.86e-09
2023-05-08 12:50:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.87e-09
2023-05-08 12:51:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.88e-09
2023-05-08 12:51:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.89e-09
2023-05-08 12:51:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.9e-09
2023-05-08 12:51:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.91e-09
2023-05-08 12:51:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.92e-09
2023-05-08 12:51:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.93e-09
2023-05-08 12:51:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.94e-09
2023-05-08 12:51:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.95e-09
2023-05-08 12:51:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.96e-09
2023-05-08 12:51:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.97e-09
2023-05-08 12:51:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.98e-09
2023-05-08 12:51:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=2.99e-09
2023-05-08 12:51:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=3e-09
2023-05-08 12:51:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.01e-09
2023-05-08 12:51:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.02e-09
2023-05-08 12:51:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.03e-09
2023-05-08 12:51:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.04e-09
2023-05-08 12:51:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.05e-09
2023-05-08 12:51:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.06e-09
2023-05-08 12:51:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.07e-09
2023-05-08 12:51:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.08e-09
2023-05-08 12:51:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.09e-09
2023-05-08 12:51:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.1e-09
2023-05-08 12:51:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.11e-09
2023-05-08 12:51:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.12e-09
2023-05-08 12:51:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.13e-09
2023-05-08 12:51:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.14e-09
2023-05-08 12:51:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.15e-09
2023-05-08 12:51:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.16e-09
2023-05-08 12:51:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.17e-09
2023-05-08 12:51:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.18e-09
2023-05-08 12:51:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.19e-09
2023-05-08 12:51:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.2e-09
2023-05-08 12:51:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.21e-09
2023-05-08 12:51:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.22e-09
2023-05-08 12:51:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.23e-09
2023-05-08 12:51:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.24e-09
2023-05-08 12:51:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.25e-09
2023-05-08 12:51:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.26e-09
2023-05-08 12:51:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.27e-09
2023-05-08 12:51:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.28e-09
2023-05-08 12:51:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.29e-09
2023-05-08 12:51:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.3e-09
2023-05-08 12:51:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.31e-09
2023-05-08 12:51:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.32e-09
2023-05-08 12:51:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.33e-09
2023-05-08 12:51:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.34e-09
2023-05-08 12:51:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.35e-09
2023-05-08 12:51:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.36e-09
2023-05-08 12:51:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.37e-09
2023-05-08 12:51:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.38e-09
2023-05-08 12:51:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.39e-09
2023-05-08 12:51:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.4e-09
2023-05-08 12:51:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.41e-09
2023-05-08 12:51:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.42e-09
2023-05-08 12:51:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.43e-09
2023-05-08 12:51:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.44e-09
2023-05-08 12:51:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.45e-09
2023-05-08 12:51:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.46e-09
2023-05-08 12:51:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.47e-09
2023-05-08 12:51:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.48e-09
2023-05-08 12:51:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.49e-09
2023-05-08 12:51:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.5e-09
2023-05-08 12:51:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.51e-09
2023-05-08 12:51:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.52e-09
2023-05-08 12:51:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.53e-09
2023-05-08 12:51:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.54e-09
2023-05-08 12:51:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.55e-09
2023-05-08 12:51:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.56e-09
2023-05-08 12:51:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.57e-09
2023-05-08 12:51:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.58e-09
2023-05-08 12:51:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.59e-09
2023-05-08 12:51:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.6e-09
2023-05-08 12:51:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.61e-09
2023-05-08 12:51:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.62e-09
2023-05-08 12:51:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.63e-09
2023-05-08 12:51:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.64e-09
2023-05-08 12:51:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.65e-09
2023-05-08 12:52:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.66e-09
2023-05-08 12:52:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.67e-09
2023-05-08 12:52:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.68e-09
2023-05-08 12:52:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.69e-09
2023-05-08 12:52:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.7e-09
2023-05-08 12:52:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.71e-09
2023-05-08 12:52:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.72e-09
2023-05-08 12:52:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.73e-09
2023-05-08 12:52:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.74e-09
2023-05-08 12:52:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.75e-09
2023-05-08 12:52:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.76e-09
2023-05-08 12:52:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.77e-09
2023-05-08 12:52:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.78e-09
2023-05-08 12:52:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.79e-09
2023-05-08 12:52:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.8e-09
2023-05-08 12:52:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.81e-09
2023-05-08 12:52:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.82e-09
2023-05-08 12:52:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.83e-09
2023-05-08 12:52:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.84e-09
2023-05-08 12:52:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.85e-09
2023-05-08 12:52:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.86e-09
2023-05-08 12:52:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.87e-09
2023-05-08 12:52:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.88e-09
2023-05-08 12:52:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.89e-09
2023-05-08 12:52:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.9e-09
2023-05-08 12:52:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.91e-09
2023-05-08 12:52:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.92e-09
2023-05-08 12:52:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.93e-09
2023-05-08 12:52:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.94e-09
2023-05-08 12:52:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.95e-09
2023-05-08 12:52:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.96e-09
2023-05-08 12:52:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.97e-09
2023-05-08 12:52:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.98e-09
2023-05-08 12:52:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=3.99e-09
2023-05-08 12:52:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=4e-09
2023-05-08 12:52:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.01e-09
2023-05-08 12:52:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.02e-09
2023-05-08 12:52:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.03e-09
2023-05-08 12:52:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.04e-09
2023-05-08 12:52:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.05e-09
2023-05-08 12:52:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.06e-09
2023-05-08 12:52:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.07e-09
2023-05-08 12:52:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.08e-09
2023-05-08 12:52:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.09e-09
2023-05-08 12:52:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.1e-09
2023-05-08 12:52:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.11e-09
2023-05-08 12:52:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.12e-09
2023-05-08 12:52:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.13e-09
2023-05-08 12:52:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.14e-09
2023-05-08 12:52:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.15e-09
2023-05-08 12:52:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.16e-09
2023-05-08 12:52:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.17e-09
2023-05-08 12:52:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.18e-09
2023-05-08 12:52:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.19e-09
2023-05-08 12:52:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.2e-09
2023-05-08 12:52:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.21e-09
2023-05-08 12:52:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.22e-09
2023-05-08 12:52:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.23e-09
2023-05-08 12:52:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.24e-09
2023-05-08 12:52:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.25e-09
2023-05-08 12:52:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.26e-09
2023-05-08 12:52:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.27e-09
2023-05-08 12:52:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.28e-09
2023-05-08 12:52:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.29e-09
2023-05-08 12:52:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.3e-09
2023-05-08 12:52:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.31e-09
2023-05-08 12:52:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.32e-09
2023-05-08 12:52:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.33e-09
2023-05-08 12:52:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.34e-09
2023-05-08 12:52:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.35e-09
2023-05-08 12:52:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.36e-09
2023-05-08 12:52:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.37e-09
2023-05-08 12:52:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.38e-09
2023-05-08 12:52:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.39e-09
2023-05-08 12:52:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.4e-09
2023-05-08 12:52:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.41e-09
2023-05-08 12:52:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.42e-09
2023-05-08 12:52:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.43e-09
2023-05-08 12:52:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.44e-09
2023-05-08 12:52:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.45e-09
2023-05-08 12:52:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.46e-09
2023-05-08 12:52:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.47e-09
2023-05-08 12:53:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.48e-09
2023-05-08 12:53:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.49e-09
2023-05-08 12:53:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.5e-09
2023-05-08 12:53:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.51e-09
2023-05-08 12:53:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.52e-09
2023-05-08 12:53:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.53e-09
2023-05-08 12:53:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.54e-09
2023-05-08 12:53:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.55e-09
2023-05-08 12:53:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.56e-09
2023-05-08 12:53:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.57e-09
2023-05-08 12:53:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.58e-09
2023-05-08 12:53:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.59e-09
2023-05-08 12:53:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.6e-09
2023-05-08 12:53:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.61e-09
2023-05-08 12:53:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.62e-09
2023-05-08 12:53:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.63e-09
2023-05-08 12:53:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.64e-09
2023-05-08 12:53:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.65e-09
2023-05-08 12:53:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.66e-09
2023-05-08 12:53:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.67e-09
2023-05-08 12:53:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.68e-09
2023-05-08 12:53:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.69e-09
2023-05-08 12:53:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.7e-09
2023-05-08 12:53:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.71e-09
2023-05-08 12:53:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.72e-09
2023-05-08 12:53:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.73e-09
2023-05-08 12:53:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.74e-09
2023-05-08 12:53:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.75e-09
2023-05-08 12:53:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.76e-09
2023-05-08 12:53:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.77e-09
2023-05-08 12:53:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.78e-09
2023-05-08 12:53:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.79e-09
2023-05-08 12:53:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.8e-09
2023-05-08 12:53:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.81e-09
2023-05-08 12:53:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.82e-09
2023-05-08 12:53:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.83e-09
2023-05-08 12:53:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.84e-09
2023-05-08 12:53:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.85e-09
2023-05-08 12:53:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.86e-09
2023-05-08 12:53:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.87e-09
2023-05-08 12:53:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.88e-09
2023-05-08 12:53:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.89e-09
2023-05-08 12:53:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.9e-09
2023-05-08 12:53:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.91e-09
2023-05-08 12:53:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.92e-09
2023-05-08 12:53:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.93e-09
2023-05-08 12:53:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.94e-09
2023-05-08 12:53:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.95e-09
2023-05-08 12:53:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.96e-09
2023-05-08 12:53:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.97e-09
2023-05-08 12:53:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.98e-09
2023-05-08 12:53:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=4.99e-09
2023-05-08 12:53:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=5e-09
2023-05-08 12:53:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.01e-09
2023-05-08 12:53:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.02e-09
2023-05-08 12:53:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.03e-09
2023-05-08 12:53:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.04e-09
2023-05-08 12:53:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.05e-09
2023-05-08 12:53:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.06e-09
2023-05-08 12:53:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.07e-09
2023-05-08 12:53:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.08e-09
2023-05-08 12:53:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.09e-09
2023-05-08 12:53:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.1e-09
2023-05-08 12:53:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.11e-09
2023-05-08 12:53:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.12e-09
2023-05-08 12:53:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.13e-09
2023-05-08 12:53:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.14e-09
2023-05-08 12:53:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.15e-09
2023-05-08 12:53:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.16e-09
2023-05-08 12:53:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.17e-09
2023-05-08 12:53:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.18e-09
2023-05-08 12:53:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.19e-09
2023-05-08 12:53:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.2e-09
2023-05-08 12:53:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.21e-09
2023-05-08 12:53:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.22e-09
2023-05-08 12:53:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.23e-09
2023-05-08 12:53:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.24e-09
2023-05-08 12:53:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.25e-09
2023-05-08 12:53:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.26e-09
2023-05-08 12:53:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.27e-09
2023-05-08 12:53:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.28e-09
2023-05-08 12:53:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.29e-09
2023-05-08 12:53:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.3e-09
2023-05-08 12:53:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.31e-09
2023-05-08 12:53:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.32e-09
2023-05-08 12:53:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.33e-09
2023-05-08 12:54:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.34e-09
2023-05-08 12:54:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.35e-09
2023-05-08 12:54:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.36e-09
2023-05-08 12:54:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.37e-09
2023-05-08 12:54:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.38e-09
2023-05-08 12:54:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.39e-09
2023-05-08 12:54:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.4e-09
2023-05-08 12:54:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.41e-09
2023-05-08 12:54:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.42e-09
2023-05-08 12:54:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.43e-09
2023-05-08 12:54:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.44e-09
2023-05-08 12:54:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.45e-09
2023-05-08 12:54:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.46e-09
2023-05-08 12:54:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.47e-09
2023-05-08 12:54:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.48e-09
2023-05-08 12:54:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.49e-09
2023-05-08 12:54:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.5e-09
2023-05-08 12:54:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.51e-09
2023-05-08 12:54:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.52e-09
2023-05-08 12:54:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.53e-09
2023-05-08 12:54:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.54e-09
2023-05-08 12:54:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.55e-09
2023-05-08 12:54:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.56e-09
2023-05-08 12:54:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.57e-09
2023-05-08 12:54:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.58e-09
2023-05-08 12:54:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.59e-09
2023-05-08 12:54:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.6e-09
2023-05-08 12:54:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.61e-09
2023-05-08 12:54:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.62e-09
2023-05-08 12:54:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.63e-09
2023-05-08 12:54:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.64e-09
2023-05-08 12:54:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.65e-09
2023-05-08 12:54:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.66e-09
2023-05-08 12:54:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.67e-09
2023-05-08 12:54:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.68e-09
2023-05-08 12:54:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.69e-09
2023-05-08 12:54:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.7e-09
2023-05-08 12:54:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.71e-09
2023-05-08 12:54:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.72e-09
2023-05-08 12:54:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.73e-09
2023-05-08 12:54:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.74e-09
2023-05-08 12:54:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.75e-09
2023-05-08 12:54:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.76e-09
2023-05-08 12:54:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.77e-09
2023-05-08 12:54:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.78e-09
2023-05-08 12:54:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.79e-09
2023-05-08 12:54:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.8e-09
2023-05-08 12:54:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.81e-09
2023-05-08 12:54:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.82e-09
2023-05-08 12:54:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.83e-09
2023-05-08 12:54:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.84e-09
2023-05-08 12:54:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.85e-09
2023-05-08 12:54:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.86e-09
2023-05-08 12:54:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.87e-09
2023-05-08 12:54:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.88e-09
2023-05-08 12:54:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.89e-09
2023-05-08 12:54:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.9e-09
2023-05-08 12:54:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.91e-09
2023-05-08 12:54:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.92e-09
2023-05-08 12:54:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.93e-09
2023-05-08 12:54:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.94e-09
2023-05-08 12:54:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.95e-09
2023-05-08 12:54:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.96e-09
2023-05-08 12:54:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.97e-09
2023-05-08 12:54:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.98e-09
2023-05-08 12:54:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=5.99e-09
2023-05-08 12:54:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=6e-09
2023-05-08 12:54:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.01e-09
2023-05-08 12:54:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.02e-09
2023-05-08 12:54:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.03e-09
2023-05-08 12:54:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.04e-09
2023-05-08 12:54:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.05e-09
2023-05-08 12:54:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.06e-09
2023-05-08 12:54:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.07e-09
2023-05-08 12:54:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.08e-09
2023-05-08 12:54:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.09e-09
2023-05-08 12:54:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.1e-09
2023-05-08 12:54:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.11e-09
2023-05-08 12:55:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.12e-09
2023-05-08 12:55:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.13e-09
2023-05-08 12:55:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.14e-09
2023-05-08 12:55:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.15e-09
2023-05-08 12:55:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.16e-09
2023-05-08 12:55:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.17e-09
2023-05-08 12:55:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.18e-09
2023-05-08 12:55:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.19e-09
2023-05-08 12:55:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.2e-09
2023-05-08 12:55:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.21e-09
2023-05-08 12:55:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.22e-09
2023-05-08 12:55:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.23e-09
2023-05-08 12:55:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.24e-09
2023-05-08 12:55:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.25e-09
2023-05-08 12:55:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.26e-09
2023-05-08 12:55:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.27e-09
2023-05-08 12:55:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.28e-09
2023-05-08 12:55:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.29e-09
2023-05-08 12:55:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.3e-09
2023-05-08 12:55:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.31e-09
2023-05-08 12:55:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.32e-09
2023-05-08 12:55:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.33e-09
2023-05-08 12:55:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.34e-09
2023-05-08 12:55:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.35e-09
2023-05-08 12:55:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.36e-09
2023-05-08 12:55:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.37e-09
2023-05-08 12:55:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.38e-09
2023-05-08 12:55:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.39e-09
2023-05-08 12:55:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.4e-09
2023-05-08 12:55:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.41e-09
2023-05-08 12:55:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.42e-09
2023-05-08 12:55:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.43e-09
2023-05-08 12:55:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.44e-09
2023-05-08 12:55:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.45e-09
2023-05-08 12:55:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.46e-09
2023-05-08 12:55:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.47e-09
2023-05-08 12:55:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.48e-09
2023-05-08 12:55:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.49e-09
2023-05-08 12:55:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.5e-09
2023-05-08 12:55:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.51e-09
2023-05-08 12:55:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.52e-09
2023-05-08 12:55:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.53e-09
2023-05-08 12:55:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.54e-09
2023-05-08 12:55:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.55e-09
2023-05-08 12:55:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.56e-09
2023-05-08 12:55:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.57e-09
2023-05-08 12:55:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.58e-09
2023-05-08 12:55:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.59e-09
2023-05-08 12:55:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.6e-09
2023-05-08 12:55:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.61e-09
2023-05-08 12:55:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.62e-09
2023-05-08 12:55:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.63e-09
2023-05-08 12:55:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.64e-09
2023-05-08 12:55:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.65e-09
2023-05-08 12:55:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.66e-09
2023-05-08 12:55:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.67e-09
2023-05-08 12:55:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.68e-09
2023-05-08 12:55:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.69e-09
2023-05-08 12:55:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.7e-09
2023-05-08 12:55:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.71e-09
2023-05-08 12:55:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.72e-09
2023-05-08 12:55:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.73e-09
2023-05-08 12:55:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.74e-09
2023-05-08 12:55:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.75e-09
2023-05-08 12:55:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.76e-09
2023-05-08 12:55:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.77e-09
2023-05-08 12:55:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.78e-09
2023-05-08 12:55:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.79e-09
2023-05-08 12:55:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.8e-09
2023-05-08 12:55:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.81e-09
2023-05-08 12:55:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.82e-09
2023-05-08 12:55:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.83e-09
2023-05-08 12:55:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.84e-09
2023-05-08 12:55:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.85e-09
2023-05-08 12:55:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.86e-09
2023-05-08 12:55:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.87e-09
2023-05-08 12:55:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.88e-09
2023-05-08 12:56:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.89e-09
2023-05-08 12:56:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.9e-09
2023-05-08 12:56:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.91e-09
2023-05-08 12:56:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.92e-09
2023-05-08 12:56:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.93e-09
2023-05-08 12:56:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.94e-09
2023-05-08 12:56:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.95e-09
2023-05-08 12:56:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.96e-09
2023-05-08 12:56:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.97e-09
2023-05-08 12:56:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.98e-09
2023-05-08 12:56:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=6.99e-09
2023-05-08 12:56:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=7e-09
2023-05-08 12:56:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.01e-09
2023-05-08 12:56:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.02e-09
2023-05-08 12:56:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.03e-09
2023-05-08 12:56:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.04e-09
2023-05-08 12:56:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.05e-09
2023-05-08 12:56:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.06e-09
2023-05-08 12:56:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.07e-09
2023-05-08 12:56:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.08e-09
2023-05-08 12:56:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.09e-09
2023-05-08 12:56:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.1e-09
2023-05-08 12:56:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.11e-09
2023-05-08 12:56:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.12e-09
2023-05-08 12:56:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.13e-09
2023-05-08 12:56:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.14e-09
2023-05-08 12:56:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.15e-09
2023-05-08 12:56:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.16e-09
2023-05-08 12:56:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.17e-09
2023-05-08 12:56:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.18e-09
2023-05-08 12:56:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.19e-09
2023-05-08 12:56:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.2e-09
2023-05-08 12:56:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.21e-09
2023-05-08 12:56:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.22e-09
2023-05-08 12:56:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.23e-09
2023-05-08 12:56:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.24e-09
2023-05-08 12:56:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.25e-09
2023-05-08 12:56:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.26e-09
2023-05-08 12:56:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.27e-09
2023-05-08 12:56:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.28e-09
2023-05-08 12:56:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.29e-09
2023-05-08 12:56:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.3e-09
2023-05-08 12:56:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.31e-09
2023-05-08 12:56:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.32e-09
2023-05-08 12:56:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.33e-09
2023-05-08 12:56:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.34e-09
2023-05-08 12:56:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.35e-09
2023-05-08 12:56:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.36e-09
2023-05-08 12:56:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.37e-09
2023-05-08 12:56:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.38e-09
2023-05-08 12:56:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.39e-09
2023-05-08 12:56:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.4e-09
2023-05-08 12:56:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.41e-09
2023-05-08 12:56:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.42e-09
2023-05-08 12:56:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.43e-09
2023-05-08 12:56:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.44e-09
2023-05-08 12:56:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.45e-09
2023-05-08 12:56:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.46e-09
2023-05-08 12:56:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.47e-09
2023-05-08 12:56:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.48e-09
2023-05-08 12:56:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.49e-09
2023-05-08 12:56:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.5e-09
2023-05-08 12:56:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.51e-09
2023-05-08 12:56:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.52e-09
2023-05-08 12:56:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.53e-09
2023-05-08 12:56:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.54e-09
2023-05-08 12:56:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.55e-09
2023-05-08 12:56:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.56e-09
2023-05-08 12:56:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.57e-09
2023-05-08 12:56:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.58e-09
2023-05-08 12:56:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.59e-09
2023-05-08 12:56:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.6e-09
2023-05-08 12:56:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.61e-09
2023-05-08 12:56:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.62e-09
2023-05-08 12:56:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.63e-09
2023-05-08 12:56:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.64e-09
2023-05-08 12:56:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.65e-09
2023-05-08 12:56:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.66e-09
2023-05-08 12:56:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.67e-09
2023-05-08 12:56:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.68e-09
2023-05-08 12:56:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.69e-09
2023-05-08 12:56:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.7e-09
2023-05-08 12:56:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.71e-09
2023-05-08 12:56:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.72e-09
2023-05-08 12:56:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.73e-09
2023-05-08 12:57:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.74e-09
2023-05-08 12:57:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.75e-09
2023-05-08 12:57:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.76e-09
2023-05-08 12:57:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.77e-09
2023-05-08 12:57:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.78e-09
2023-05-08 12:57:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.79e-09
2023-05-08 12:57:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.8e-09
2023-05-08 12:57:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.81e-09
2023-05-08 12:57:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.82e-09
2023-05-08 12:57:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.83e-09
2023-05-08 12:57:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.84e-09
2023-05-08 12:57:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.85e-09
2023-05-08 12:57:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.86e-09
2023-05-08 12:57:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.87e-09
2023-05-08 12:57:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.88e-09
2023-05-08 12:57:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.89e-09
2023-05-08 12:57:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.9e-09
2023-05-08 12:57:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.91e-09
2023-05-08 12:57:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.92e-09
2023-05-08 12:57:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.93e-09
2023-05-08 12:57:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.94e-09
2023-05-08 12:57:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.95e-09
2023-05-08 12:57:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.96e-09
2023-05-08 12:57:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.97e-09
2023-05-08 12:57:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.98e-09
2023-05-08 12:57:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=7.99e-09
2023-05-08 12:57:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=8e-09
2023-05-08 12:57:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.01e-09
2023-05-08 12:57:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.02e-09
2023-05-08 12:57:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.03e-09
2023-05-08 12:57:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.04e-09
2023-05-08 12:57:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.05e-09
2023-05-08 12:57:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.06e-09
2023-05-08 12:57:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.07e-09
2023-05-08 12:57:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.08e-09
2023-05-08 12:57:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.09e-09
2023-05-08 12:57:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.1e-09
2023-05-08 12:57:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.11e-09
2023-05-08 12:57:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.12e-09
2023-05-08 12:57:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.13e-09
2023-05-08 12:57:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.14e-09
2023-05-08 12:57:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.15e-09
2023-05-08 12:57:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.16e-09
2023-05-08 12:57:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.17e-09
2023-05-08 12:57:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.18e-09
2023-05-08 12:57:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.19e-09
2023-05-08 12:57:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.2e-09
2023-05-08 12:57:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.21e-09
2023-05-08 12:57:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.22e-09
2023-05-08 12:57:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.23e-09
2023-05-08 12:57:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.24e-09
2023-05-08 12:57:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.25e-09
2023-05-08 12:57:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.26e-09
2023-05-08 12:57:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.27e-09
2023-05-08 12:57:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.28e-09
2023-05-08 12:57:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.29e-09
2023-05-08 12:57:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.3e-09
2023-05-08 12:57:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.31e-09
2023-05-08 12:57:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.32e-09
2023-05-08 12:57:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.33e-09
2023-05-08 12:57:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.34e-09
2023-05-08 12:57:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.35e-09
2023-05-08 12:57:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.36e-09
2023-05-08 12:57:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.37e-09
2023-05-08 12:57:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.38e-09
2023-05-08 12:57:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.39e-09
2023-05-08 12:57:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.4e-09
2023-05-08 12:57:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.41e-09
2023-05-08 12:57:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.42e-09
2023-05-08 12:57:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.43e-09
2023-05-08 12:57:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.44e-09
2023-05-08 12:57:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.45e-09
2023-05-08 12:57:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.46e-09
2023-05-08 12:57:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.47e-09
2023-05-08 12:57:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.48e-09
2023-05-08 12:57:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.49e-09
2023-05-08 12:57:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.5e-09
2023-05-08 12:57:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.51e-09
2023-05-08 12:57:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.52e-09
2023-05-08 12:57:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.53e-09
2023-05-08 12:57:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.54e-09
2023-05-08 12:57:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.55e-09
2023-05-08 12:57:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.56e-09
2023-05-08 12:57:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.57e-09
2023-05-08 12:57:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.58e-09
2023-05-08 12:57:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.59e-09
2023-05-08 12:57:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.6e-09
2023-05-08 12:57:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.61e-09
2023-05-08 12:57:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.62e-09
2023-05-08 12:57:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.63e-09
2023-05-08 12:57:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.64e-09
2023-05-08 12:57:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.65e-09
2023-05-08 12:57:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.66e-09
2023-05-08 12:57:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.67e-09
2023-05-08 12:57:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.68e-09
2023-05-08 12:57:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.69e-09
2023-05-08 12:57:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.7e-09
2023-05-08 12:57:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.71e-09
2023-05-08 12:57:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.72e-09
2023-05-08 12:57:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.73e-09
2023-05-08 12:57:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.74e-09
2023-05-08 12:57:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.75e-09
2023-05-08 12:57:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.76e-09
2023-05-08 12:57:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.77e-09
2023-05-08 12:57:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.78e-09
2023-05-08 12:57:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.79e-09
2023-05-08 12:57:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.8e-09
2023-05-08 12:57:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.81e-09
2023-05-08 12:57:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.82e-09
2023-05-08 12:57:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.83e-09
2023-05-08 12:57:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.84e-09
2023-05-08 12:57:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.85e-09
2023-05-08 12:58:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.86e-09
2023-05-08 12:58:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.87e-09
2023-05-08 12:58:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.88e-09
2023-05-08 12:58:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.89e-09
2023-05-08 12:58:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.9e-09
2023-05-08 12:58:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.91e-09
2023-05-08 12:58:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.92e-09
2023-05-08 12:58:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.93e-09
2023-05-08 12:58:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.94e-09
2023-05-08 12:58:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.95e-09
2023-05-08 12:58:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.96e-09
2023-05-08 12:58:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.97e-09
2023-05-08 12:58:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.98e-09
2023-05-08 12:58:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=8.99e-09
2023-05-08 12:58:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=9e-09
2023-05-08 12:58:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.01e-09
2023-05-08 12:58:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.02e-09
2023-05-08 12:58:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.03e-09
2023-05-08 12:58:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.04e-09
2023-05-08 12:58:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.05e-09
2023-05-08 12:58:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.06e-09
2023-05-08 12:58:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.07e-09
2023-05-08 12:58:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.08e-09
2023-05-08 12:58:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.09e-09
2023-05-08 12:58:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.1e-09
2023-05-08 12:58:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.11e-09
2023-05-08 12:58:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.12e-09
2023-05-08 12:58:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.13e-09
2023-05-08 12:58:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.14e-09
2023-05-08 12:58:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.15e-09
2023-05-08 12:58:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.16e-09
2023-05-08 12:58:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.17e-09
2023-05-08 12:58:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.18e-09
2023-05-08 12:58:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.19e-09
2023-05-08 12:58:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.2e-09
2023-05-08 12:58:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.21e-09
2023-05-08 12:58:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.22e-09
2023-05-08 12:58:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.23e-09
2023-05-08 12:58:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.24e-09
2023-05-08 12:58:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.25e-09
2023-05-08 12:58:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.26e-09
2023-05-08 12:58:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.27e-09
2023-05-08 12:58:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.28e-09
2023-05-08 12:58:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.29e-09
2023-05-08 12:58:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.3e-09
2023-05-08 12:58:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.31e-09
2023-05-08 12:58:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.32e-09
2023-05-08 12:58:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.33e-09
2023-05-08 12:58:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.34e-09
2023-05-08 12:58:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.35e-09
2023-05-08 12:58:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.36e-09
2023-05-08 12:58:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.37e-09
2023-05-08 12:58:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.38e-09
2023-05-08 12:58:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.39e-09
2023-05-08 12:58:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.4e-09
2023-05-08 12:58:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.41e-09
2023-05-08 12:58:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.42e-09
2023-05-08 12:58:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.43e-09
2023-05-08 12:58:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.44e-09
2023-05-08 12:58:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.45e-09
2023-05-08 12:58:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.46e-09
2023-05-08 12:58:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.47e-09
2023-05-08 12:58:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.48e-09
2023-05-08 12:58:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.49e-09
2023-05-08 12:58:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.5e-09
2023-05-08 12:58:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.51e-09
2023-05-08 12:58:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.52e-09
2023-05-08 12:58:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.53e-09
2023-05-08 12:58:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.54e-09
2023-05-08 12:58:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.55e-09
2023-05-08 12:58:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.56e-09
2023-05-08 12:58:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.57e-09
2023-05-08 12:58:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.58e-09
2023-05-08 12:58:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.59e-09
2023-05-08 12:58:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.6e-09
2023-05-08 12:58:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.61e-09
2023-05-08 12:58:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.62e-09
2023-05-08 12:58:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.63e-09
2023-05-08 12:58:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.64e-09
2023-05-08 12:58:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.65e-09
2023-05-08 12:58:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.66e-09
2023-05-08 12:58:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.67e-09
2023-05-08 12:58:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.68e-09
2023-05-08 12:58:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.69e-09
2023-05-08 12:58:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.7e-09
2023-05-08 12:58:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.71e-09
2023-05-08 12:58:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.72e-09
2023-05-08 12:58:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.73e-09
2023-05-08 12:58:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.74e-09
2023-05-08 12:58:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.75e-09
2023-05-08 12:58:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.76e-09
2023-05-08 12:58:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.77e-09
2023-05-08 12:58:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.78e-09
2023-05-08 12:58:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.79e-09
2023-05-08 12:58:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.8e-09
2023-05-08 12:58:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.81e-09
2023-05-08 12:58:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.82e-09
2023-05-08 12:58:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.83e-09
2023-05-08 12:58:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.84e-09
2023-05-08 12:58:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.85e-09
2023-05-08 12:58:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.86e-09
2023-05-08 12:58:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.87e-09
2023-05-08 12:58:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.88e-09
2023-05-08 12:58:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.89e-09
2023-05-08 12:58:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.9e-09
2023-05-08 12:58:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.91e-09
2023-05-08 12:58:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.92e-09
2023-05-08 12:58:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.93e-09
2023-05-08 12:58:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.94e-09
2023-05-08 12:58:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.95e-09
2023-05-08 12:58:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.96e-09
2023-05-08 12:58:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.97e-09
2023-05-08 12:59:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.98e-09
2023-05-08 12:59:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=9.99e-09
2023-05-08 12:59:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1e-08
2023-05-08 12:59:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.001e-08
2023-05-08 12:59:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.002e-08
2023-05-08 12:59:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.003e-08
2023-05-08 12:59:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.004e-08
2023-05-08 12:59:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.005e-08
2023-05-08 12:59:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.006e-08
2023-05-08 12:59:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.007e-08
2023-05-08 12:59:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.008e-08
2023-05-08 12:59:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.009e-08
2023-05-08 12:59:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.01e-08
2023-05-08 12:59:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.011e-08
2023-05-08 12:59:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.012e-08
2023-05-08 12:59:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.013e-08
2023-05-08 12:59:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.014e-08
2023-05-08 12:59:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.015e-08
2023-05-08 12:59:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.016e-08
2023-05-08 12:59:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.017e-08
2023-05-08 12:59:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.018e-08
2023-05-08 12:59:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.019e-08
2023-05-08 12:59:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.02e-08
2023-05-08 12:59:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.021e-08
2023-05-08 12:59:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.022e-08
2023-05-08 12:59:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.023e-08
2023-05-08 12:59:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.024e-08
2023-05-08 12:59:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.025e-08
2023-05-08 12:59:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.026e-08
2023-05-08 12:59:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.027e-08
2023-05-08 12:59:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.028e-08
2023-05-08 12:59:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.029e-08
2023-05-08 12:59:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.03e-08
2023-05-08 12:59:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.031e-08
2023-05-08 12:59:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.032e-08
2023-05-08 12:59:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.033e-08
2023-05-08 12:59:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.034e-08
2023-05-08 12:59:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.035e-08
2023-05-08 12:59:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.036e-08
2023-05-08 12:59:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.037e-08
2023-05-08 12:59:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.038e-08
2023-05-08 12:59:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.039e-08
2023-05-08 12:59:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.04e-08
2023-05-08 12:59:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.041e-08
2023-05-08 12:59:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.042e-08
2023-05-08 12:59:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.043e-08
2023-05-08 12:59:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.044e-08
2023-05-08 12:59:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.045e-08
2023-05-08 12:59:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.046e-08
2023-05-08 12:59:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.047e-08
2023-05-08 12:59:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.048e-08
2023-05-08 12:59:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.049e-08
2023-05-08 12:59:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.05e-08
2023-05-08 12:59:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.051e-08
2023-05-08 12:59:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.052e-08
2023-05-08 12:59:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.053e-08
2023-05-08 12:59:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.054e-08
2023-05-08 12:59:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.055e-08
2023-05-08 12:59:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.056e-08
2023-05-08 12:59:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.057e-08
2023-05-08 12:59:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.058e-08
2023-05-08 12:59:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.059e-08
2023-05-08 12:59:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.06e-08
2023-05-08 12:59:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.061e-08
2023-05-08 12:59:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.062e-08
2023-05-08 12:59:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.063e-08
2023-05-08 12:59:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.064e-08
2023-05-08 12:59:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.065e-08
2023-05-08 12:59:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.066e-08
2023-05-08 12:59:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.067e-08
2023-05-08 12:59:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.068e-08
2023-05-08 12:59:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.069e-08
2023-05-08 12:59:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.07e-08
2023-05-08 12:59:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.071e-08
2023-05-08 12:59:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.072e-08
2023-05-08 12:59:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.073e-08
2023-05-08 12:59:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.074e-08
2023-05-08 12:59:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.075e-08
2023-05-08 12:59:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.076e-08
2023-05-08 12:59:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.077e-08
2023-05-08 12:59:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.078e-08
2023-05-08 12:59:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.079e-08
2023-05-08 12:59:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.08e-08
2023-05-08 12:59:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.081e-08
2023-05-08 12:59:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.082e-08
2023-05-08 12:59:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.083e-08
2023-05-08 12:59:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.084e-08
2023-05-08 12:59:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.085e-08
2023-05-08 12:59:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.086e-08
2023-05-08 12:59:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.087e-08
2023-05-08 12:59:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.088e-08
2023-05-08 12:59:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.089e-08
2023-05-08 12:59:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.09e-08
2023-05-08 12:59:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.091e-08
2023-05-08 12:59:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.092e-08
2023-05-08 12:59:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.093e-08
2023-05-08 12:59:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.094e-08
2023-05-08 12:59:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.095e-08
2023-05-08 12:59:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.096e-08
2023-05-08 12:59:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.097e-08
2023-05-08 12:59:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.098e-08
2023-05-08 12:59:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.099e-08
2023-05-08 12:59:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.1e-08
2023-05-08 12:59:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.101e-08
2023-05-08 12:59:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.102e-08
2023-05-08 12:59:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.103e-08
2023-05-08 12:59:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.104e-08
2023-05-08 12:59:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.105e-08
2023-05-08 12:59:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.106e-08
2023-05-08 12:59:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.107e-08
2023-05-08 12:59:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.108e-08
2023-05-08 12:59:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.109e-08
2023-05-08 12:59:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.11e-08
2023-05-08 12:59:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.111e-08
2023-05-08 12:59:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.112e-08
2023-05-08 13:00:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.113e-08
2023-05-08 13:00:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.114e-08
2023-05-08 13:00:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.115e-08
2023-05-08 13:00:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.116e-08
2023-05-08 13:00:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.117e-08
2023-05-08 13:00:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.118e-08
2023-05-08 13:00:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.119e-08
2023-05-08 13:00:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.12e-08
2023-05-08 13:00:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.121e-08
2023-05-08 13:00:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.122e-08
2023-05-08 13:00:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.123e-08
2023-05-08 13:00:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.124e-08
2023-05-08 13:00:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.125e-08
2023-05-08 13:00:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.126e-08
2023-05-08 13:00:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.127e-08
2023-05-08 13:00:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.128e-08
2023-05-08 13:00:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.129e-08
2023-05-08 13:00:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.13e-08
2023-05-08 13:00:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.131e-08
2023-05-08 13:00:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.132e-08
2023-05-08 13:00:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.133e-08
2023-05-08 13:00:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.134e-08
2023-05-08 13:00:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.135e-08
2023-05-08 13:00:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.136e-08
2023-05-08 13:00:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.137e-08
2023-05-08 13:00:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.138e-08
2023-05-08 13:00:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.139e-08
2023-05-08 13:00:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.14e-08
2023-05-08 13:00:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.141e-08
2023-05-08 13:00:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.142e-08
2023-05-08 13:00:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.143e-08
2023-05-08 13:00:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.144e-08
2023-05-08 13:00:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.145e-08
2023-05-08 13:00:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.146e-08
2023-05-08 13:00:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.147e-08
2023-05-08 13:00:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.148e-08
2023-05-08 13:00:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.149e-08
2023-05-08 13:00:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.15e-08
2023-05-08 13:00:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.151e-08
2023-05-08 13:00:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.152e-08
2023-05-08 13:00:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.153e-08
2023-05-08 13:00:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.154e-08
2023-05-08 13:00:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.155e-08
2023-05-08 13:00:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.156e-08
2023-05-08 13:00:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.157e-08
2023-05-08 13:00:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.158e-08
2023-05-08 13:00:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.159e-08
2023-05-08 13:00:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.16e-08
2023-05-08 13:00:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.161e-08
2023-05-08 13:00:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.162e-08
2023-05-08 13:00:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.163e-08
2023-05-08 13:00:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.164e-08
2023-05-08 13:00:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.165e-08
2023-05-08 13:00:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.166e-08
2023-05-08 13:00:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.167e-08
2023-05-08 13:00:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.168e-08
2023-05-08 13:00:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.169e-08
2023-05-08 13:00:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.17e-08
2023-05-08 13:00:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.171e-08
2023-05-08 13:00:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.172e-08
2023-05-08 13:00:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.173e-08
2023-05-08 13:00:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.174e-08
2023-05-08 13:00:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.175e-08
2023-05-08 13:00:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.176e-08
2023-05-08 13:00:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.177e-08
2023-05-08 13:00:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.178e-08
2023-05-08 13:00:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.179e-08
2023-05-08 13:00:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.18e-08
2023-05-08 13:00:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.181e-08
2023-05-08 13:00:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.182e-08
2023-05-08 13:00:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.183e-08
2023-05-08 13:00:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.184e-08
2023-05-08 13:00:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.185e-08
2023-05-08 13:00:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.186e-08
2023-05-08 13:00:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.187e-08
2023-05-08 13:00:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.188e-08
2023-05-08 13:00:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.189e-08
2023-05-08 13:00:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.19e-08
2023-05-08 13:00:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.191e-08
2023-05-08 13:00:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.192e-08
2023-05-08 13:00:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.193e-08
2023-05-08 13:00:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.194e-08
2023-05-08 13:00:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.195e-08
2023-05-08 13:00:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.196e-08
2023-05-08 13:00:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.197e-08
2023-05-08 13:00:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.198e-08
2023-05-08 13:00:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.199e-08
2023-05-08 13:00:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.2e-08
2023-05-08 13:00:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.201e-08
2023-05-08 13:00:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.202e-08
2023-05-08 13:00:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.203e-08
2023-05-08 13:00:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.204e-08
2023-05-08 13:00:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.205e-08
2023-05-08 13:00:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.206e-08
2023-05-08 13:00:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.207e-08
2023-05-08 13:00:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.208e-08
2023-05-08 13:00:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.209e-08
2023-05-08 13:00:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.21e-08
2023-05-08 13:00:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.211e-08
2023-05-08 13:00:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.212e-08
2023-05-08 13:00:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.213e-08
2023-05-08 13:00:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.214e-08
2023-05-08 13:00:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.215e-08
2023-05-08 13:00:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.216e-08
2023-05-08 13:00:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.217e-08
2023-05-08 13:00:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.218e-08
2023-05-08 13:00:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.219e-08
2023-05-08 13:00:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.22e-08
2023-05-08 13:00:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.221e-08
2023-05-08 13:00:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.222e-08
2023-05-08 13:00:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.223e-08
2023-05-08 13:00:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.224e-08
2023-05-08 13:00:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.225e-08
2023-05-08 13:00:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.226e-08
2023-05-08 13:00:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.227e-08
2023-05-08 13:00:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.228e-08
2023-05-08 13:00:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.229e-08
2023-05-08 13:00:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.23e-08
2023-05-08 13:00:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.231e-08
2023-05-08 13:00:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.232e-08
2023-05-08 13:00:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.233e-08
2023-05-08 13:00:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.234e-08
2023-05-08 13:00:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.235e-08
2023-05-08 13:00:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.236e-08
2023-05-08 13:00:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.237e-08
2023-05-08 13:00:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.238e-08
2023-05-08 13:00:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.239e-08
2023-05-08 13:00:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.24e-08
2023-05-08 13:00:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.241e-08
2023-05-08 13:00:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.242e-08
2023-05-08 13:00:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.243e-08
2023-05-08 13:00:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.244e-08
2023-05-08 13:00:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.245e-08
2023-05-08 13:00:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.246e-08
2023-05-08 13:00:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.247e-08
2023-05-08 13:00:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.248e-08
2023-05-08 13:00:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.249e-08
2023-05-08 13:00:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.25e-08
2023-05-08 13:00:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.251e-08
2023-05-08 13:00:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.252e-08
2023-05-08 13:00:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.253e-08
2023-05-08 13:00:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.254e-08
2023-05-08 13:00:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.255e-08
2023-05-08 13:00:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.256e-08
2023-05-08 13:00:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.257e-08
2023-05-08 13:00:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.258e-08
2023-05-08 13:00:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.259e-08
2023-05-08 13:00:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.26e-08
2023-05-08 13:00:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.261e-08
2023-05-08 13:00:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.262e-08
2023-05-08 13:00:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.263e-08
2023-05-08 13:00:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.264e-08
2023-05-08 13:00:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.265e-08
2023-05-08 13:00:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.266e-08
2023-05-08 13:00:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.267e-08
2023-05-08 13:00:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.268e-08
2023-05-08 13:00:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.269e-08
2023-05-08 13:00:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.27e-08
2023-05-08 13:00:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.271e-08
2023-05-08 13:00:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.272e-08
2023-05-08 13:00:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.273e-08
2023-05-08 13:00:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.274e-08
2023-05-08 13:00:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.275e-08
2023-05-08 13:00:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.276e-08
2023-05-08 13:00:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.277e-08
2023-05-08 13:00:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.278e-08
2023-05-08 13:00:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.279e-08
2023-05-08 13:00:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.28e-08
2023-05-08 13:00:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.281e-08
2023-05-08 13:00:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.282e-08
2023-05-08 13:00:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.283e-08
2023-05-08 13:00:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.284e-08
2023-05-08 13:00:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.285e-08
2023-05-08 13:00:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.286e-08
2023-05-08 13:00:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.287e-08
2023-05-08 13:00:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.288e-08
2023-05-08 13:00:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.289e-08
2023-05-08 13:00:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.29e-08
2023-05-08 13:00:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.291e-08
2023-05-08 13:01:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.292e-08
2023-05-08 13:01:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.293e-08
2023-05-08 13:01:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.294e-08
2023-05-08 13:01:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.295e-08
2023-05-08 13:01:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.296e-08
2023-05-08 13:01:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.297e-08
2023-05-08 13:01:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.298e-08
2023-05-08 13:01:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.299e-08
2023-05-08 13:01:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.3e-08
2023-05-08 13:01:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.301e-08
2023-05-08 13:01:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.302e-08
2023-05-08 13:01:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.303e-08
2023-05-08 13:01:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.304e-08
2023-05-08 13:01:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.305e-08
2023-05-08 13:01:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.306e-08
2023-05-08 13:01:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.307e-08
2023-05-08 13:01:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.308e-08
2023-05-08 13:01:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.309e-08
2023-05-08 13:01:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.31e-08
2023-05-08 13:01:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.311e-08
2023-05-08 13:01:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.312e-08
2023-05-08 13:01:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.313e-08
2023-05-08 13:01:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.314e-08
2023-05-08 13:01:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.315e-08
2023-05-08 13:01:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.316e-08
2023-05-08 13:01:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.317e-08
2023-05-08 13:01:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.318e-08
2023-05-08 13:01:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.319e-08
2023-05-08 13:01:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.32e-08
2023-05-08 13:01:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.321e-08
2023-05-08 13:01:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.322e-08
2023-05-08 13:01:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.323e-08
2023-05-08 13:01:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.324e-08
2023-05-08 13:01:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.325e-08
2023-05-08 13:01:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.326e-08
2023-05-08 13:01:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.327e-08
2023-05-08 13:01:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.328e-08
2023-05-08 13:01:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.329e-08
2023-05-08 13:01:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.33e-08
2023-05-08 13:01:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.331e-08
2023-05-08 13:01:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.332e-08
2023-05-08 13:01:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.333e-08
2023-05-08 13:01:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.334e-08
2023-05-08 13:01:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.335e-08
2023-05-08 13:01:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.336e-08
2023-05-08 13:01:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.337e-08
2023-05-08 13:01:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.338e-08
2023-05-08 13:01:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.339e-08
2023-05-08 13:01:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.34e-08
2023-05-08 13:01:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.341e-08
2023-05-08 13:01:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.342e-08
2023-05-08 13:01:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.343e-08
2023-05-08 13:01:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.344e-08
2023-05-08 13:01:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.345e-08
2023-05-08 13:01:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.346e-08
2023-05-08 13:01:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.347e-08
2023-05-08 13:01:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.348e-08
2023-05-08 13:01:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.349e-08
2023-05-08 13:01:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.35e-08
2023-05-08 13:01:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.351e-08
2023-05-08 13:01:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.352e-08
2023-05-08 13:01:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.353e-08
2023-05-08 13:01:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.354e-08
2023-05-08 13:01:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.355e-08
2023-05-08 13:01:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.356e-08
2023-05-08 13:01:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.357e-08
2023-05-08 13:01:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.358e-08
2023-05-08 13:01:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.359e-08
2023-05-08 13:01:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.36e-08
2023-05-08 13:01:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.361e-08
2023-05-08 13:01:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.362e-08
2023-05-08 13:01:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.363e-08
2023-05-08 13:01:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.364e-08
2023-05-08 13:01:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.365e-08
2023-05-08 13:01:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.366e-08
2023-05-08 13:01:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.367e-08
2023-05-08 13:01:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.368e-08
2023-05-08 13:01:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.369e-08
2023-05-08 13:01:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.37e-08
2023-05-08 13:01:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.371e-08
2023-05-08 13:01:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.372e-08
2023-05-08 13:01:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.373e-08
2023-05-08 13:01:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.374e-08
2023-05-08 13:01:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.375e-08
2023-05-08 13:01:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.376e-08
2023-05-08 13:01:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.377e-08
2023-05-08 13:01:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.378e-08
2023-05-08 13:01:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.379e-08
2023-05-08 13:01:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.38e-08
2023-05-08 13:01:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.381e-08
2023-05-08 13:01:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.382e-08
2023-05-08 13:01:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.383e-08
2023-05-08 13:01:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.384e-08
2023-05-08 13:01:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.385e-08
2023-05-08 13:01:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.386e-08
2023-05-08 13:01:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.387e-08
2023-05-08 13:01:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.388e-08
2023-05-08 13:01:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.389e-08
2023-05-08 13:01:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.39e-08
2023-05-08 13:01:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.391e-08
2023-05-08 13:01:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.392e-08
2023-05-08 13:01:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.393e-08
2023-05-08 13:01:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.394e-08
2023-05-08 13:01:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.395e-08
2023-05-08 13:01:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.396e-08
2023-05-08 13:01:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.397e-08
2023-05-08 13:01:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.398e-08
2023-05-08 13:01:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.399e-08
2023-05-08 13:01:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.4e-08
2023-05-08 13:01:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.401e-08
2023-05-08 13:01:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.402e-08
2023-05-08 13:01:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.403e-08
2023-05-08 13:01:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.404e-08
2023-05-08 13:01:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.405e-08
2023-05-08 13:01:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.406e-08
2023-05-08 13:01:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.407e-08
2023-05-08 13:01:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.408e-08
2023-05-08 13:01:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.409e-08
2023-05-08 13:01:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.41e-08
2023-05-08 13:01:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.411e-08
2023-05-08 13:01:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.412e-08
2023-05-08 13:01:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.413e-08
2023-05-08 13:01:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.414e-08
2023-05-08 13:01:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.415e-08
2023-05-08 13:01:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.416e-08
2023-05-08 13:01:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.417e-08
2023-05-08 13:01:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.418e-08
2023-05-08 13:01:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.419e-08
2023-05-08 13:01:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.42e-08
2023-05-08 13:01:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.421e-08
2023-05-08 13:01:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.422e-08
2023-05-08 13:01:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.423e-08
2023-05-08 13:01:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.424e-08
2023-05-08 13:01:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.425e-08
2023-05-08 13:01:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.426e-08
2023-05-08 13:01:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.427e-08
2023-05-08 13:01:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.428e-08
2023-05-08 13:01:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.429e-08
2023-05-08 13:01:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.43e-08
2023-05-08 13:01:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.431e-08
2023-05-08 13:01:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.432e-08
2023-05-08 13:01:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.433e-08
2023-05-08 13:01:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.434e-08
2023-05-08 13:01:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.435e-08
2023-05-08 13:01:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.436e-08
2023-05-08 13:01:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.437e-08
2023-05-08 13:01:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.438e-08
2023-05-08 13:01:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.439e-08
2023-05-08 13:01:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.44e-08
2023-05-08 13:01:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.441e-08
2023-05-08 13:01:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.442e-08
2023-05-08 13:01:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.443e-08
2023-05-08 13:01:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.444e-08
2023-05-08 13:01:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.445e-08
2023-05-08 13:01:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.446e-08
2023-05-08 13:01:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.447e-08
2023-05-08 13:01:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.448e-08
2023-05-08 13:01:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.449e-08
2023-05-08 13:01:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.45e-08
2023-05-08 13:01:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.451e-08
2023-05-08 13:01:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.452e-08
2023-05-08 13:01:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.453e-08
2023-05-08 13:01:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.454e-08
2023-05-08 13:01:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.455e-08
2023-05-08 13:01:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.456e-08
2023-05-08 13:01:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.457e-08
2023-05-08 13:01:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.458e-08
2023-05-08 13:01:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.459e-08
2023-05-08 13:01:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.46e-08
2023-05-08 13:01:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.461e-08
2023-05-08 13:01:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.462e-08
2023-05-08 13:01:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.463e-08
2023-05-08 13:01:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.464e-08
2023-05-08 13:01:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.465e-08
2023-05-08 13:01:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.466e-08
2023-05-08 13:01:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.467e-08
2023-05-08 13:01:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.468e-08
2023-05-08 13:01:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.469e-08
2023-05-08 13:01:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.47e-08
2023-05-08 13:01:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.471e-08
2023-05-08 13:01:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.472e-08
2023-05-08 13:01:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.473e-08
2023-05-08 13:01:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.474e-08
2023-05-08 13:01:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.475e-08
2023-05-08 13:01:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.476e-08
2023-05-08 13:01:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.477e-08
2023-05-08 13:01:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.478e-08
2023-05-08 13:01:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.479e-08
2023-05-08 13:01:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.48e-08
2023-05-08 13:01:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.481e-08
2023-05-08 13:01:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.482e-08
2023-05-08 13:01:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.483e-08
2023-05-08 13:01:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.484e-08
2023-05-08 13:01:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.485e-08
2023-05-08 13:01:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.486e-08
2023-05-08 13:01:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.487e-08
2023-05-08 13:01:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.488e-08
2023-05-08 13:01:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.489e-08
2023-05-08 13:02:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.49e-08
2023-05-08 13:02:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.491e-08
2023-05-08 13:02:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.492e-08
2023-05-08 13:02:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.493e-08
2023-05-08 13:02:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.494e-08
2023-05-08 13:02:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.495e-08
2023-05-08 13:02:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.496e-08
2023-05-08 13:02:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.497e-08
2023-05-08 13:02:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.498e-08
2023-05-08 13:02:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.499e-08
2023-05-08 13:02:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.5e-08
2023-05-08 13:02:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.501e-08
2023-05-08 13:02:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.502e-08
2023-05-08 13:02:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.503e-08
2023-05-08 13:02:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.504e-08
2023-05-08 13:02:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.505e-08
2023-05-08 13:02:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.506e-08
2023-05-08 13:02:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.507e-08
2023-05-08 13:02:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.508e-08
2023-05-08 13:02:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.509e-08
2023-05-08 13:02:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.51e-08
2023-05-08 13:02:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.511e-08
2023-05-08 13:02:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.512e-08
2023-05-08 13:02:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.513e-08
2023-05-08 13:02:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.514e-08
2023-05-08 13:02:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.515e-08
2023-05-08 13:02:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.516e-08
2023-05-08 13:02:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.517e-08
2023-05-08 13:02:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.518e-08
2023-05-08 13:02:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.519e-08
2023-05-08 13:02:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.52e-08
2023-05-08 13:02:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.521e-08
2023-05-08 13:02:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.522e-08
2023-05-08 13:02:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.523e-08
2023-05-08 13:02:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.524e-08
2023-05-08 13:02:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.525e-08
2023-05-08 13:02:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.526e-08
2023-05-08 13:02:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.527e-08
2023-05-08 13:02:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.528e-08
2023-05-08 13:02:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.529e-08
2023-05-08 13:02:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.53e-08
2023-05-08 13:02:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.531e-08
2023-05-08 13:02:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.532e-08
2023-05-08 13:02:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.533e-08
2023-05-08 13:02:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.534e-08
2023-05-08 13:02:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.535e-08
2023-05-08 13:02:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.536e-08
2023-05-08 13:02:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.537e-08
2023-05-08 13:02:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.538e-08
2023-05-08 13:02:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.539e-08
2023-05-08 13:02:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.54e-08
2023-05-08 13:02:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.541e-08
2023-05-08 13:02:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.542e-08
2023-05-08 13:02:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.543e-08
2023-05-08 13:02:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.544e-08
2023-05-08 13:02:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.545e-08
2023-05-08 13:02:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.546e-08
2023-05-08 13:02:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.547e-08
2023-05-08 13:02:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.548e-08
2023-05-08 13:02:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.549e-08
2023-05-08 13:02:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.55e-08
2023-05-08 13:02:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.551e-08
2023-05-08 13:02:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.552e-08
2023-05-08 13:02:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.553e-08
2023-05-08 13:02:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.554e-08
2023-05-08 13:02:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.555e-08
2023-05-08 13:02:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.556e-08
2023-05-08 13:02:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.557e-08
2023-05-08 13:02:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.558e-08
2023-05-08 13:02:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.559e-08
2023-05-08 13:02:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.56e-08
2023-05-08 13:02:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.561e-08
2023-05-08 13:02:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.562e-08
2023-05-08 13:02:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.563e-08
2023-05-08 13:02:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.564e-08
2023-05-08 13:02:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.565e-08
2023-05-08 13:02:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.566e-08
2023-05-08 13:02:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.567e-08
2023-05-08 13:02:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.568e-08
2023-05-08 13:02:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.569e-08
2023-05-08 13:02:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.57e-08
2023-05-08 13:02:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.571e-08
2023-05-08 13:02:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.572e-08
2023-05-08 13:02:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.573e-08
2023-05-08 13:02:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.574e-08
2023-05-08 13:02:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.575e-08
2023-05-08 13:02:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.576e-08
2023-05-08 13:02:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.577e-08
2023-05-08 13:02:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.578e-08
2023-05-08 13:02:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.579e-08
2023-05-08 13:02:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.58e-08
2023-05-08 13:02:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.581e-08
2023-05-08 13:02:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.582e-08
2023-05-08 13:02:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.583e-08
2023-05-08 13:02:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.584e-08
2023-05-08 13:02:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.585e-08
2023-05-08 13:02:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.586e-08
2023-05-08 13:02:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.587e-08
2023-05-08 13:02:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.588e-08
2023-05-08 13:02:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.589e-08
2023-05-08 13:02:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.59e-08
2023-05-08 13:02:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.591e-08
2023-05-08 13:02:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.592e-08
2023-05-08 13:02:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.593e-08
2023-05-08 13:02:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.594e-08
2023-05-08 13:02:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.595e-08
2023-05-08 13:02:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.596e-08
2023-05-08 13:02:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.597e-08
2023-05-08 13:02:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.598e-08
2023-05-08 13:02:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.599e-08
2023-05-08 13:02:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.6e-08
2023-05-08 13:02:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.601e-08
2023-05-08 13:02:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.602e-08
2023-05-08 13:02:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.603e-08
2023-05-08 13:02:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.604e-08
2023-05-08 13:02:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.605e-08
2023-05-08 13:02:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.606e-08
2023-05-08 13:02:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.607e-08
2023-05-08 13:02:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.608e-08
2023-05-08 13:02:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.609e-08
2023-05-08 13:02:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.61e-08
2023-05-08 13:02:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.611e-08
2023-05-08 13:02:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.612e-08
2023-05-08 13:02:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.613e-08
2023-05-08 13:02:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.614e-08
2023-05-08 13:02:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.615e-08
2023-05-08 13:02:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.616e-08
2023-05-08 13:02:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.617e-08
2023-05-08 13:02:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.618e-08
2023-05-08 13:02:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.619e-08
2023-05-08 13:02:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.62e-08
2023-05-08 13:02:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.621e-08
2023-05-08 13:02:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.622e-08
2023-05-08 13:02:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.623e-08
2023-05-08 13:02:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.624e-08
2023-05-08 13:02:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.625e-08
2023-05-08 13:02:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.626e-08
2023-05-08 13:02:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.627e-08
2023-05-08 13:02:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.628e-08
2023-05-08 13:02:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.629e-08
2023-05-08 13:02:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.63e-08
2023-05-08 13:02:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.631e-08
2023-05-08 13:02:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.632e-08
2023-05-08 13:02:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.633e-08
2023-05-08 13:02:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.634e-08
2023-05-08 13:02:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.635e-08
2023-05-08 13:02:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.636e-08
2023-05-08 13:02:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.637e-08
2023-05-08 13:02:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.638e-08
2023-05-08 13:02:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.639e-08
2023-05-08 13:02:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.64e-08
2023-05-08 13:02:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.641e-08
2023-05-08 13:02:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.642e-08
2023-05-08 13:02:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.643e-08
2023-05-08 13:02:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.644e-08
2023-05-08 13:02:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.645e-08
2023-05-08 13:02:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.646e-08
2023-05-08 13:02:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.647e-08
2023-05-08 13:02:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.648e-08
2023-05-08 13:02:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.649e-08
2023-05-08 13:02:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.65e-08
2023-05-08 13:02:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.651e-08
2023-05-08 13:02:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.652e-08
2023-05-08 13:02:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.653e-08
2023-05-08 13:02:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.654e-08
2023-05-08 13:02:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.655e-08
2023-05-08 13:02:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.656e-08
2023-05-08 13:02:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.657e-08
2023-05-08 13:02:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.658e-08
2023-05-08 13:02:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.659e-08
2023-05-08 13:02:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.66e-08
2023-05-08 13:02:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.661e-08
2023-05-08 13:02:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.662e-08
2023-05-08 13:02:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.663e-08
2023-05-08 13:02:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.664e-08
2023-05-08 13:02:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.665e-08
2023-05-08 13:02:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.666e-08
2023-05-08 13:02:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.667e-08
2023-05-08 13:02:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.668e-08
2023-05-08 13:02:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.669e-08
2023-05-08 13:02:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.67e-08
2023-05-08 13:02:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.671e-08
2023-05-08 13:02:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.672e-08
2023-05-08 13:02:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.673e-08
2023-05-08 13:02:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.674e-08
2023-05-08 13:02:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.675e-08
2023-05-08 13:02:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.676e-08
2023-05-08 13:02:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.677e-08
2023-05-08 13:02:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.678e-08
2023-05-08 13:02:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.679e-08
2023-05-08 13:02:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.68e-08
2023-05-08 13:02:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.681e-08
2023-05-08 13:02:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.682e-08
2023-05-08 13:02:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.683e-08
2023-05-08 13:02:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.684e-08
2023-05-08 13:02:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.685e-08
2023-05-08 13:02:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.686e-08
2023-05-08 13:02:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.687e-08
2023-05-08 13:02:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.688e-08
2023-05-08 13:02:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.689e-08
2023-05-08 13:02:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.69e-08
2023-05-08 13:02:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.691e-08
2023-05-08 13:02:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.692e-08
2023-05-08 13:02:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.693e-08
2023-05-08 13:02:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.694e-08
2023-05-08 13:02:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.695e-08
2023-05-08 13:02:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.696e-08
2023-05-08 13:02:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.697e-08
2023-05-08 13:02:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.698e-08
2023-05-08 13:02:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.699e-08
2023-05-08 13:02:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.7e-08
2023-05-08 13:02:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.701e-08
2023-05-08 13:02:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.702e-08
2023-05-08 13:02:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.703e-08
2023-05-08 13:03:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.704e-08
2023-05-08 13:03:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.705e-08
2023-05-08 13:03:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.706e-08
2023-05-08 13:03:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.707e-08
2023-05-08 13:03:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.708e-08
2023-05-08 13:03:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.709e-08
2023-05-08 13:03:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.71e-08
2023-05-08 13:03:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.711e-08
2023-05-08 13:03:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.712e-08
2023-05-08 13:03:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.713e-08
2023-05-08 13:03:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.714e-08
2023-05-08 13:03:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.715e-08
2023-05-08 13:03:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.716e-08
2023-05-08 13:03:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.717e-08
2023-05-08 13:03:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.718e-08
2023-05-08 13:03:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.719e-08
2023-05-08 13:03:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.72e-08
2023-05-08 13:03:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.721e-08
2023-05-08 13:03:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.722e-08
2023-05-08 13:03:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.723e-08
2023-05-08 13:03:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.724e-08
2023-05-08 13:03:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.725e-08
2023-05-08 13:03:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.726e-08
2023-05-08 13:03:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.727e-08
2023-05-08 13:03:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.728e-08
2023-05-08 13:03:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.729e-08
2023-05-08 13:03:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.73e-08
2023-05-08 13:03:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.731e-08
2023-05-08 13:03:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.732e-08
2023-05-08 13:03:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.733e-08
2023-05-08 13:03:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.734e-08
2023-05-08 13:03:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.735e-08
2023-05-08 13:03:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.736e-08
2023-05-08 13:03:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.737e-08
2023-05-08 13:03:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.738e-08
2023-05-08 13:03:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.739e-08
2023-05-08 13:03:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.74e-08
2023-05-08 13:03:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.741e-08
2023-05-08 13:03:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.742e-08
2023-05-08 13:03:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.743e-08
2023-05-08 13:03:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.744e-08
2023-05-08 13:03:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.745e-08
2023-05-08 13:03:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.746e-08
2023-05-08 13:03:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.747e-08
2023-05-08 13:03:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.748e-08
2023-05-08 13:03:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.749e-08
2023-05-08 13:03:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.75e-08
2023-05-08 13:03:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.751e-08
2023-05-08 13:03:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.752e-08
2023-05-08 13:03:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.753e-08
2023-05-08 13:03:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.754e-08
2023-05-08 13:03:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.755e-08
2023-05-08 13:03:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.756e-08
2023-05-08 13:03:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.757e-08
2023-05-08 13:03:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.758e-08
2023-05-08 13:03:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.759e-08
2023-05-08 13:03:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.76e-08
2023-05-08 13:03:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.761e-08
2023-05-08 13:03:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.762e-08
2023-05-08 13:03:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.763e-08
2023-05-08 13:03:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.764e-08
2023-05-08 13:03:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.765e-08
2023-05-08 13:03:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.766e-08
2023-05-08 13:03:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.767e-08
2023-05-08 13:03:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.768e-08
2023-05-08 13:03:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.769e-08
2023-05-08 13:03:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.77e-08
2023-05-08 13:03:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.771e-08
2023-05-08 13:03:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.772e-08
2023-05-08 13:03:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.773e-08
2023-05-08 13:03:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.774e-08
2023-05-08 13:03:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.775e-08
2023-05-08 13:03:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.776e-08
2023-05-08 13:03:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.777e-08
2023-05-08 13:03:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.778e-08
2023-05-08 13:03:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.779e-08
2023-05-08 13:03:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.78e-08
2023-05-08 13:03:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.781e-08
2023-05-08 13:03:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.782e-08
2023-05-08 13:03:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.783e-08
2023-05-08 13:03:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.784e-08
2023-05-08 13:03:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.785e-08
2023-05-08 13:03:22 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.786e-08
2023-05-08 13:03:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.787e-08
2023-05-08 13:03:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.788e-08
2023-05-08 13:03:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.789e-08
2023-05-08 13:03:23 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.79e-08
2023-05-08 13:03:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.791e-08
2023-05-08 13:03:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.792e-08
2023-05-08 13:03:24 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.793e-08
2023-05-08 13:03:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.794e-08
2023-05-08 13:03:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.795e-08
2023-05-08 13:03:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.796e-08
2023-05-08 13:03:25 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.797e-08
2023-05-08 13:03:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.798e-08
2023-05-08 13:03:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.799e-08
2023-05-08 13:03:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.8e-08
2023-05-08 13:03:26 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.801e-08
2023-05-08 13:03:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.802e-08
2023-05-08 13:03:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.803e-08
2023-05-08 13:03:27 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.804e-08
2023-05-08 13:03:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.805e-08
2023-05-08 13:03:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.806e-08
2023-05-08 13:03:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.807e-08
2023-05-08 13:03:28 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.808e-08
2023-05-08 13:03:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.809e-08
2023-05-08 13:03:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.81e-08
2023-05-08 13:03:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.811e-08
2023-05-08 13:03:29 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.812e-08
2023-05-08 13:03:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.813e-08
2023-05-08 13:03:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.814e-08
2023-05-08 13:03:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.815e-08
2023-05-08 13:03:30 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.816e-08
2023-05-08 13:03:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.817e-08
2023-05-08 13:03:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.818e-08
2023-05-08 13:03:31 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.819e-08
2023-05-08 13:03:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.82e-08
2023-05-08 13:03:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.821e-08
2023-05-08 13:03:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.822e-08
2023-05-08 13:03:32 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.823e-08
2023-05-08 13:03:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.824e-08
2023-05-08 13:03:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.825e-08
2023-05-08 13:03:33 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.826e-08
2023-05-08 13:03:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.827e-08
2023-05-08 13:03:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.828e-08
2023-05-08 13:03:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.829e-08
2023-05-08 13:03:34 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.83e-08
2023-05-08 13:03:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.831e-08
2023-05-08 13:03:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.832e-08
2023-05-08 13:03:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.833e-08
2023-05-08 13:03:35 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.834e-08
2023-05-08 13:03:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.835e-08
2023-05-08 13:03:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.836e-08
2023-05-08 13:03:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.837e-08
2023-05-08 13:03:36 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.838e-08
2023-05-08 13:03:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.839e-08
2023-05-08 13:03:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.84e-08
2023-05-08 13:03:37 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.841e-08
2023-05-08 13:03:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.842e-08
2023-05-08 13:03:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.843e-08
2023-05-08 13:03:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.844e-08
2023-05-08 13:03:38 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.845e-08
2023-05-08 13:03:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.846e-08
2023-05-08 13:03:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.847e-08
2023-05-08 13:03:39 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.848e-08
2023-05-08 13:03:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.849e-08
2023-05-08 13:03:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.85e-08
2023-05-08 13:03:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.851e-08
2023-05-08 13:03:40 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.852e-08
2023-05-08 13:03:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.853e-08
2023-05-08 13:03:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.854e-08
2023-05-08 13:03:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.855e-08
2023-05-08 13:03:41 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.856e-08
2023-05-08 13:03:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.857e-08
2023-05-08 13:03:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.858e-08
2023-05-08 13:03:42 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.859e-08
2023-05-08 13:03:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.86e-08
2023-05-08 13:03:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.861e-08
2023-05-08 13:03:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.862e-08
2023-05-08 13:03:43 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.863e-08
2023-05-08 13:03:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.864e-08
2023-05-08 13:03:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.865e-08
2023-05-08 13:03:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.866e-08
2023-05-08 13:03:44 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.867e-08
2023-05-08 13:03:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.868e-08
2023-05-08 13:03:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.869e-08
2023-05-08 13:03:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.87e-08
2023-05-08 13:03:45 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.871e-08
2023-05-08 13:03:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.872e-08
2023-05-08 13:03:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.873e-08
2023-05-08 13:03:46 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.874e-08
2023-05-08 13:03:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.875e-08
2023-05-08 13:03:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.876e-08
2023-05-08 13:03:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.877e-08
2023-05-08 13:03:47 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.878e-08
2023-05-08 13:03:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.879e-08
2023-05-08 13:03:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.88e-08
2023-05-08 13:03:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.881e-08
2023-05-08 13:03:48 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.882e-08
2023-05-08 13:03:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.883e-08
2023-05-08 13:03:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.884e-08
2023-05-08 13:03:49 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.885e-08
2023-05-08 13:03:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.886e-08
2023-05-08 13:03:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.887e-08
2023-05-08 13:03:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.888e-08
2023-05-08 13:03:50 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.889e-08
2023-05-08 13:03:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.89e-08
2023-05-08 13:03:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.891e-08
2023-05-08 13:03:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.892e-08
2023-05-08 13:03:51 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.893e-08
2023-05-08 13:03:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.894e-08
2023-05-08 13:03:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.895e-08
2023-05-08 13:03:52 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.896e-08
2023-05-08 13:03:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.897e-08
2023-05-08 13:03:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.898e-08
2023-05-08 13:03:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.899e-08
2023-05-08 13:03:53 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.9e-08
2023-05-08 13:03:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.901e-08
2023-05-08 13:03:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.902e-08
2023-05-08 13:03:54 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.903e-08
2023-05-08 13:03:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.904e-08
2023-05-08 13:03:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.905e-08
2023-05-08 13:03:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.906e-08
2023-05-08 13:03:55 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.907e-08
2023-05-08 13:03:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.908e-08
2023-05-08 13:03:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.909e-08
2023-05-08 13:03:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.91e-08
2023-05-08 13:03:56 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.911e-08
2023-05-08 13:03:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.912e-08
2023-05-08 13:03:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.913e-08
2023-05-08 13:03:57 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.914e-08
2023-05-08 13:03:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.915e-08
2023-05-08 13:03:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.916e-08
2023-05-08 13:03:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.917e-08
2023-05-08 13:03:58 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.918e-08
2023-05-08 13:03:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.919e-08
2023-05-08 13:03:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.92e-08
2023-05-08 13:03:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.921e-08
2023-05-08 13:03:59 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.922e-08
2023-05-08 13:04:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.923e-08
2023-05-08 13:04:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.924e-08
2023-05-08 13:04:00 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.925e-08
2023-05-08 13:04:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.926e-08
2023-05-08 13:04:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.927e-08
2023-05-08 13:04:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.928e-08
2023-05-08 13:04:01 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.929e-08
2023-05-08 13:04:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.93e-08
2023-05-08 13:04:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.931e-08
2023-05-08 13:04:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.932e-08
2023-05-08 13:04:02 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.933e-08
2023-05-08 13:04:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.934e-08
2023-05-08 13:04:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.935e-08
2023-05-08 13:04:03 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.936e-08
2023-05-08 13:04:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.937e-08
2023-05-08 13:04:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.938e-08
2023-05-08 13:04:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.939e-08
2023-05-08 13:04:04 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.94e-08
2023-05-08 13:04:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.941e-08
2023-05-08 13:04:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.942e-08
2023-05-08 13:04:05 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.943e-08
2023-05-08 13:04:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.944e-08
2023-05-08 13:04:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.945e-08
2023-05-08 13:04:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.946e-08
2023-05-08 13:04:06 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.947e-08
2023-05-08 13:04:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.948e-08
2023-05-08 13:04:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.949e-08
2023-05-08 13:04:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.95e-08
2023-05-08 13:04:07 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.951e-08
2023-05-08 13:04:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.952e-08
2023-05-08 13:04:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.953e-08
2023-05-08 13:04:08 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.954e-08
2023-05-08 13:04:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.955e-08
2023-05-08 13:04:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.956e-08
2023-05-08 13:04:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.957e-08
2023-05-08 13:04:09 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.958e-08
2023-05-08 13:04:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.959e-08
2023-05-08 13:04:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.96e-08
2023-05-08 13:04:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.961e-08
2023-05-08 13:04:10 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.962e-08
2023-05-08 13:04:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.963e-08
2023-05-08 13:04:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.964e-08
2023-05-08 13:04:11 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.965e-08
2023-05-08 13:04:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.966e-08
2023-05-08 13:04:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.967e-08
2023-05-08 13:04:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.968e-08
2023-05-08 13:04:12 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.969e-08
2023-05-08 13:04:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.97e-08
2023-05-08 13:04:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.971e-08
2023-05-08 13:04:13 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.972e-08
2023-05-08 13:04:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.973e-08
2023-05-08 13:04:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.974e-08
2023-05-08 13:04:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.975e-08
2023-05-08 13:04:14 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.976e-08
2023-05-08 13:04:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.977e-08
2023-05-08 13:04:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.978e-08
2023-05-08 13:04:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.979e-08
2023-05-08 13:04:15 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.98e-08
2023-05-08 13:04:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.981e-08
2023-05-08 13:04:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.982e-08
2023-05-08 13:04:16 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.983e-08
2023-05-08 13:04:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.984e-08
2023-05-08 13:04:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.985e-08
2023-05-08 13:04:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.986e-08
2023-05-08 13:04:17 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.987e-08
2023-05-08 13:04:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.988e-08
2023-05-08 13:04:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.989e-08
2023-05-08 13:04:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.99e-08
2023-05-08 13:04:18 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.991e-08
2023-05-08 13:04:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.992e-08
2023-05-08 13:04:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.993e-08
2023-05-08 13:04:19 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.994e-08
2023-05-08 13:04:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.995e-08
2023-05-08 13:04:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.996e-08
2023-05-08 13:04:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.997e-08
2023-05-08 13:04:20 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.998e-08
2023-05-08 13:04:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=1.999e-08
2023-05-08 13:04:21 magnum.np:INFO [LLG] step: dt= 1e-11 t=2e-08
===========================================================================================
TIMER REPORT
===========================================================================================
Operation No of calls Avg time [ms] Total time [s]
----------------------------------------- ------------- --------------- ----------------
LLGSolver.step 2283 480.905 1097.91
ExchangeField.h 656306 0.308665 202.579
UniaxialAnisotropyField.h 656306 0.271603 178.255
ExternalField.h 656325 0.268436 176.182
LLGSolver.step 19 290.308 5.51586
ExchangeField.h 5614 0.178445 1.00179
LLGSolver.step 2000 513.678 1027.36
ExchangeField.h 575472 0.313705 180.528
UniaxialAnisotropyField.h 575472 0.29 166.887
ExternalField.h 575472 0.280644 161.503
ExternalField.h 2000 1.21345 2.42689
UniaxialAnisotropyField.h 5614 0.165044 0.92656
ExternalField.h 5614 0.161228 0.905136
ExternalField.h 2283 1.34831 3.07819
----------------------------------------- ------------- --------------- ----------------
Total 1031.88
Missing -69.1004
===========================================================================================
Plot Results:
[19]:
from os import path
if not path.isdir("ref"):
!mkdir ref
!wget -P ref https://gitlab.com/magnum.np/magnum.np/raw/main/demos/sp_domainwall_pinning/ref/m_ref.dat
!wget -P ref https://gitlab.com/magnum.np/magnum.np/raw/main/demos/sp_domainwall_pinning/ref/m_dieter.dat
!wget -P ref https://gitlab.com/magnum.np/magnum.np/raw/main/demos/sp_domainwall_pinning/ref/m_magnumaf.dat
[20]:
import matplotlib.pyplot as plt
data = np.loadtxt("data/m.dat")
ref = np.loadtxt("ref/m_ref.dat")
data_dieter = np.loadtxt("ref/m_dieter.dat")
data_magnumaf = np.loadtxt("ref/m_magnumaf.dat")
fig, ax = plt.subplots(figsize=(10,5))
cycle = plt.rcParams['axes.prop_cycle'].by_key()['color']
ax.plot(data[:,2]*4*np.pi*1e-7, data[:,5], '-', color = cycle[0], label = "magnum.np")
ax.plot(ref[:,2][0::4]*4*np.pi*1e-7, ref[:,5][0::4], 'x', color = cycle[0], linewidth = 6, label = "reference")
ax.plot(data_dieter[:,1], data_dieter[:,3], '-', color = cycle[1], label = "dieter")
ax.plot(data_magnumaf[:,4]*4*np.pi*1e-7, data_magnumaf[:,2], '-', color = cycle[2], label = "magnum.af")
ax.set_xlim([1.4,1.8])
ax.set_ylim([-0.2,1.2])
ax.set_title("Standard Problem - Domainwall Pinning")
ax.set_xlabel("Applied Field $\mu$$_0$ H$_{ext}$ [T]")
ax.set_ylabel("Magnetization m$_y$")
ax.legend(ncol=2, loc='lower right')
ax.grid()
fig.savefig("data/results.png")
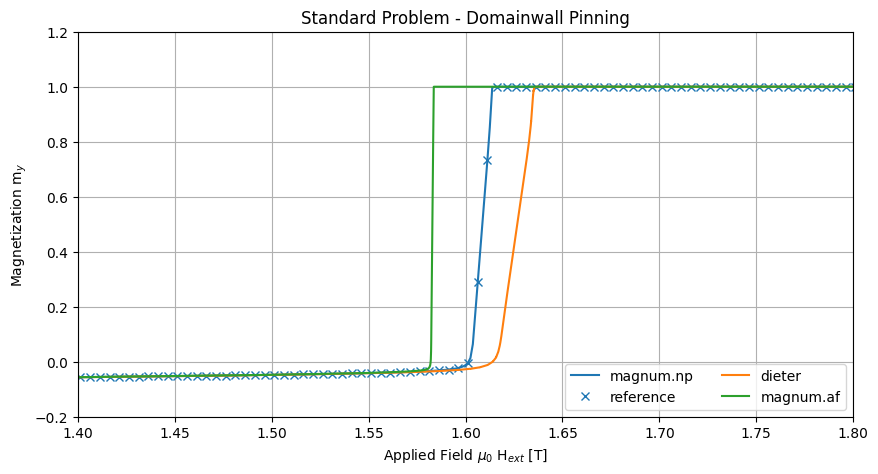